
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
planner.h
00001 /* 00002 planner.h - buffers movement commands and manages the acceleration profile plan 00003 Part of Grbl 00004 00005 Copyright (c) 2009-2011 Simen Svale Skogsrud 00006 Copyright (c) 2011 Sungeun K. Jeon 00007 00008 Grbl is free software: you can redistribute it and/or modify 00009 it under the terms of the GNU General Public License as published by 00010 the Free Software Foundation, either version 3 of the License, or 00011 (at your option) any later version. 00012 00013 Grbl is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 GNU General Public License for more details. 00017 00018 You should have received a copy of the GNU General Public License 00019 along with Grbl. If not, see <http://www.gnu.org/licenses/>. 00020 */ 00021 00022 #ifndef planner_h 00023 #define planner_h 00024 00025 #include <inttypes.h> 00026 00027 typedef enum { 00028 AT_MOVE, // move with laser off 00029 AT_LASER, // move with laser on 00030 AT_BITMAP, // move with laser modulated by bitmap 00031 AT_MOVE_ENDSTOP, // move to endstops 00032 AT_WAIT, // Dwell 00033 } eActionType; 00034 00035 typedef struct { 00036 float x; 00037 float y; 00038 float z; 00039 float e; 00040 float feed_rate; 00041 } tTarget; 00042 00043 // options for the action 00044 #define OPT_LASER_ON 1 // switch laser on 00045 #define OPT_WAIT 2 // wait at end of move 00046 #define OPT_HOME_X 4 // stop on x switch 00047 #define OPT_HOME_Y 8 00048 #define OPT_HOME_Z 16 00049 #define OPT_HOME_E 32 00050 #define OPT_BITMAP 64 // bitmap mark a line 00051 00052 00053 // This struct is used when buffering the setup for each linear movement "nominal" values are as specified in 00054 // the source g-code and may never actually be reached if acceleration management is active. 00055 typedef struct 00056 { 00057 eActionType action_type; 00058 00059 // Fields used by the bresenham algorithm for tracing the line 00060 uint32_t steps_x, steps_y, steps_z; // Step count along each axis 00061 uint32_t steps_e; 00062 uint32_t direction_bits; // The direction bit set for this block (refers to *_DIRECTION_BIT in config.h) 00063 int32_t step_event_count; // The number of step events required to complete this block 00064 uint32_t nominal_rate; // The nominal step rate for this block in step_events/minute 00065 00066 // Fields used by the motion planner to manage acceleration 00067 float nominal_speed; // The nominal speed for this block in mm/min 00068 float entry_speed; // Entry speed at previous-current junction in mm/min 00069 float max_entry_speed; // Maximum allowable junction entry speed in mm/min 00070 float millimeters; // The total travel of this block in mm 00071 uint8_t recalculate_flag; // Planner flag to recalculate trapezoids on entry junction 00072 uint8_t nominal_length_flag; // Planner flag for nominal speed always reached 00073 00074 // Settings for the trapezoid generator 00075 uint32_t initial_rate; // The jerk-adjusted step rate at start of block 00076 uint32_t final_rate; // The minimal rate at exit 00077 int32_t rate_delta; // The steps/minute to add or subtract when changing speed (must be positive) 00078 uint32_t accelerate_until; // The index of the step event on which to stop acceleration 00079 uint32_t decelerate_after; // The index of the step event on which to start decelerating 00080 00081 // extra 00082 uint8_t check_endstops; // for homing moves 00083 uint8_t options; // for further options (e.g. laser on/off, homing on axis, dwell, etc) 00084 uint16_t power; // laser power setpoint 00085 } block_t; 00086 00087 // This defines an action to enque, with its target position 00088 typedef struct { 00089 eActionType ActionType; 00090 tTarget target; 00091 uint16_t param; // argument for the action 00092 } tActionRequest; 00093 00094 00095 00096 extern tTarget startpoint; 00097 00098 // Initialize the motion plan subsystem 00099 void plan_init(); 00100 00101 // Add a new linear movement to the buffer. x, y and z is the signed, absolute target position in 00102 // millimeters. Feed rate specifies the speed of the motion. (in mm/min) 00103 void plan_buffer_line (tActionRequest *pAction); 00104 00105 void plan_buffer_action(tActionRequest *pAction); 00106 00107 // Called when the current block is no longer needed. Discards the block and makes the memory 00108 // availible for new blocks. 00109 void plan_discard_current_block(); 00110 00111 // Gets the current block. Returns NULL if buffer empty 00112 block_t *plan_get_current_block(); 00113 00114 // Enables or disables acceleration-management for upcoming blocks 00115 void plan_set_acceleration_manager_enabled(uint8_t enabled); 00116 00117 // Is acceleration-management currently enabled? 00118 int plan_is_acceleration_manager_enabled(); 00119 00120 // Reset the position vector 00121 void plan_set_current_position(tTarget *new_position); 00122 00123 void plan_set_current_position_xyz(float x, float y, float z); 00124 void plan_get_current_position_xyz(float *x, float *y, float *z); 00125 00126 void plan_set_feed_rate (tTarget *new_position); 00127 00128 uint8_t plan_queue_full (void); 00129 00130 uint8_t plan_queue_empty(void); 00131 00132 uint8_t plan_queue_items(void) ; 00133 00134 #endif
Generated on Tue Jul 12 2022 21:03:03 by
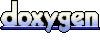