
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
laosfilesystem.h
00001 /* 00002 * 00003 * LaosFilesystem.h 00004 * Simple Long Filename system on top of SDFilesystem.h 00005 * 00006 * Copyright (c) 2011 Jaap Vermaas 00007 * 00008 * This file is part of the LaOS project (see: http://wiki.laoslaser.org 00009 * 00010 * LaOS is free software: you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation, either version 3 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * LaOS is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License 00021 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00022 */ 00023 #ifndef _LAOSFILESYSTEM_ 00024 #define _LAOSFILESYSTEM_ 00025 00026 #include "SDFileSystem.h" 00027 #include "FATFileSystem.h" 00028 #include <string> 00029 #include <ctype.h> 00030 00031 #define _LAOSFILE_TRANSTABLE "longname.sys" 00032 #define MAXFILESIZE 21 00033 #define SHORTFILESIZE 13 00034 00035 class LaosFileSystem : public SDFileSystem { 00036 public: 00037 LaosFileSystem(PinName mosi, PinName miso, PinName sclk, PinName cs, 00038 const char* name); // Create the filesystem on SD 00039 virtual ~LaosFileSystem(); // destructor 00040 FILE* openfile(char* fname, char* iom); // open a file 00041 void getlongname(char *result, char *searchname); // return long names 00042 void getshortname(char* shortname, char* name); //get a short name 00043 char pathname[MAXFILESIZE+2]; 00044 void cleanlist(); 00045 void shorten(char* name, int max); 00046 00047 private: 00048 int islegalname(char* name); 00049 int isshortname(char* name); 00050 void removespaces(char* name); 00051 void makeshortname(char* shortname, char* name); 00052 size_t dirread(char* longname, char* shortname, FILE *fp); 00053 size_t dirwrite(char* longname, char* shortname, FILE* fp); 00054 char tablename[MAXFILESIZE + SHORTFILESIZE + 1]; 00055 }; 00056 00057 void showfile(); // debug: list contents of long filesytem file 00058 void cleandir(); // delete all files in directory 00059 void printdir(); // list all files in directory (with long names) 00060 //void getfilename(char *name, int filenr); // get name of the #filenr file 00061 //int getfilenum(char *name); // get number of this filename 00062 void getprevjob(char *name); // previous job 00063 void getnextjob(char *name); // next job 00064 void writefile(char *name); // example code to open a file 00065 void removefile(char *name); // example code to remove a file 00066 int readint(FILE *fp); // read integers from open file 00067 void strtolower(char *name); // change characters to lowercase 00068 int isFirmware(char *name); // check if it's firmware 00069 void installFirmware(char *filename); // put firmware in place 00070 void removeFirmware(); // remove old firmware 00071 int SDcheckFirmware(); // check for firmware 00072 int isLaosFile(char *filename); // check extension for LaOS compatibility 00073 #endif
Generated on Tue Jul 12 2022 21:03:03 by
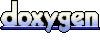