
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
global.h
00001 /* 00002 * global.h 00003 * Laos Controller, global header file, included in all other files 00004 * 00005 * Copyright (c) 2011 Peter Brier 00006 * 00007 * This file is part of the LaOS project (see: http://wiki.protospace.nl/index.php/LaOS) 00008 * 00009 * LaOS is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * LaOS is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00021 * 00022 */ 00023 #ifndef _GLOBAL_H_ 00024 #define _GLOBAL_H_ 00025 00026 #include "mbed.h" 00027 #include "LaosDisplay.h" 00028 #include "LaosMotion.h" 00029 00030 // Global configuration struct 00031 class GlobalConfig 00032 { 00033 public: 00034 int ip[4], gw[4], nm[4], dns[4], port, dhcp; // network settings 00035 int enable; // enable state (1 or 0) 00036 int autohome; // automatically home the axis at startup 00037 int autozhome; // automatically home the zaxis as well 00038 int nodisplay; // there is no display 00039 int cleandir; // remove files from SD at startup 00040 int i2cbaud; // i2cBaudrate 00041 int xmax, ymax, zmax, emax; // max values 00042 int xmin, ymin, zmin, emin; // min values 00043 int xpol, ypol, zpol, epol; // polarity for the home switches 00044 int xinv, yinv, zinv, einv; // invert signal polarity for step/dir 00045 int xhome, yhome, zhome, ehome; // home position 00046 int xrest, yrest, zrest, erest; // rest positon (moveto after job) 00047 int xhomedir, yhomedir, zhomedir, ehomedir; 00048 int homespeed; // speed used for homing [usec] 00049 int speed, xspeed, yspeed, zspeed, espeed; // Maximum linear speed and max speed per axis [mm/sec] 00050 int accel; // defaul accelletaion [mm/sec2] 00051 int tolerance; // corner tolerance [micrometer] 00052 int xscale; // steps per meter 00053 int yscale; // steps per meter 00054 int zscale; // steps per meter 00055 int escale; // steps per meter 00056 int lenable, lon, pwmmin, pwmmax, pwmfreq; // laser enable, laser on and pwm min/max [%] and frequency [Hz]; 00057 GlobalConfig(char *filename); 00058 }; 00059 extern GlobalConfig *cfg; 00060 00061 #define VERSION_STRING "\033LAOS v0.3" __DATE__ " " __TIME__ 00062 00063 #endif
Generated on Tue Jul 12 2022 21:03:03 by
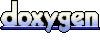