
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
LaosDisplay.h
00001 /** 00002 * LaosDisplay.cpp 00003 * User interface class, for 2x16 display and keypad 00004 * 00005 * Copyright (c) 2011 Peter Brier 00006 * 00007 * This file is part of the LaOS project (see: http://wiki.protospace.nl/index.php/LaOS) 00008 * 00009 * LaOS is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * LaOS is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00021 * 00022 * Assume (USB) serial connected display (or terminal) in future, this could be CAN or I2C connected 00023 * 00024 */ 00025 #ifndef _LAOS_DISPLAY_H_ 00026 #define _LAOS_DISPLAY_H_ 00027 00028 extern "C" void mbed_reset(); 00029 /** LaosDisplay 00030 * Connect to LCD terminal or PC 00031 * Example: 00032 * @code 00033 * @endcode 00034 */ 00035 class LaosDisplay { 00036 public: 00037 /** Make new LaosDisplay object. 00038 */ 00039 LaosDisplay(); 00040 void Simulate(); 00041 void EnableI2C(int baud); 00042 /** Display string at position (x,y) 00043 * @param x x position in characters (zero based) 00044 * @param y y position in characters (zero based) 00045 * @param s The string to display 00046 */ 00047 void write(char *s); 00048 void ShowScreen(const char *l, int *arg, char *s); 00049 void testI2C(); 00050 00051 00052 /** Clear screen 00053 */ 00054 void cls(); 00055 00056 /** Read key value. (non blocking) 00057 * @return (ASCII) character value, zero if no character is available 00058 */ 00059 int read(); 00060 00061 private: 00062 bool sim; 00063 int i2cBaud; 00064 }; 00065 00066 /* PC keyboard and I2C display 00067 * have the same keys 00068 */ 00069 #define K_UP '8' 00070 #define K_DOWN '2' 00071 #define K_LEFT '4' 00072 #define K_RIGHT '6' 00073 #define K_FUP '9' 00074 #define K_FDOWN '3' 00075 #define K_OK '5' 00076 #define K_CANCEL '7' 00077 #define K_ORIGIN '1' 00078 00079 #endif
Generated on Tue Jul 12 2022 21:03:03 by
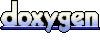