
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
LaosDisplay.cpp
00001 /** 00002 * LaosDisplay.cpp 00003 * User interface class, for 00004 * => 2x16 display and keypad 00005 * => serial connection 00006 * 00007 * Copyright (c) 2011 Peter Brier and Jaap Vermaas 00008 * 00009 * This file is part of the LaOS project (see: http://wiki.laoslaser.org/) 00010 * 00011 * LaOS is free software: you can redistribute it and/or modify 00012 * it under the terms of the GNU General Public License as published by 00013 * the Free Software Foundation, either version 3 of the License, or 00014 * (at your option) any later version. 00015 * 00016 * LaOS is distributed in the hope that it will be useful, 00017 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00019 * GNU General Public License for more details. 00020 * 00021 * You should have received a copy of the GNU General Public License 00022 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00023 * 00024 */ 00025 #include "LaosDisplay.h" 00026 #include "mbed.h" 00027 00028 // Serial 00029 Serial serial(USBTX, USBRX); 00030 #define _SERIAL_BAUD 115200 00031 00032 // I2C 00033 I2C i2c(p9, p10); // sda, scl 00034 #define _I2C_ADDRESS 0x04 00035 #define _I2C_HOME 0xFE 00036 #define _I2C_CLS 0xFF 00037 #define _I2C_BAUD 9600 00038 00039 // Make new config file object 00040 LaosDisplay::LaosDisplay() 00041 { 00042 int i2cBaud = _I2C_BAUD; 00043 i2c.frequency(i2cBaud ); 00044 int serialBaud = _SERIAL_BAUD; 00045 serial.baud(serialBaud); 00046 00047 char key; 00048 // test I2C, if we cannot read, display is not attached, enable simulation 00049 // wait 1 second to make sure that I2C has time to power on! 00050 wait(3); 00051 sim = i2c.read(_I2C_ADDRESS ,&key, 1) != 0; 00052 if (sim) { 00053 printf("LaosDisplay()\n"); 00054 printf("Display() Simulation=ON, I2C Baudrate=%d\n", i2cBaud ); 00055 } 00056 } 00057 00058 // Test I2C again when config file is read 00059 void LaosDisplay::testI2C() { 00060 char key; 00061 sim = i2c.read(_I2C_ADDRESS ,&key, 1) != 0; 00062 if (sim) mbed_reset(); 00063 } 00064 00065 // Write string 00066 void LaosDisplay::write(char *s) 00067 { 00068 if ( sim ) 00069 { 00070 while (*s) 00071 serial.putc(*s++); 00072 return; 00073 } else { 00074 while (*s) 00075 i2c.write(_I2C_ADDRESS, s++, 1); 00076 } 00077 } 00078 00079 // Clear screen 00080 void LaosDisplay::cls() 00081 { 00082 char s[2]; 00083 s[0] = _I2C_HOME; 00084 s[1] = 0; 00085 write(s); 00086 } 00087 00088 // Read Key 00089 int LaosDisplay::read() 00090 { 00091 char key = 0; 00092 if (sim) 00093 { 00094 if ( serial.readable() ) 00095 key = serial.getc(); 00096 else 00097 key = 0; 00098 } 00099 else 00100 i2c.read(_I2C_ADDRESS ,&key, 1); 00101 if ((key < '1') || (key > '9')) 00102 key = 0; 00103 return key; 00104 } 00105 00106 /** 00107 *** Screens are defined with: 00108 *** name, line[2], int* i[4], char *s; 00109 *** 00110 *** in the lines, the following replacements are made: 00111 *** $$$$$$ : "$" chars are replaced with the string argument for this screen (left alligned), if argument is NULL, spaces are inserted 00112 *** +9876543210: "+" and numbers are replaced with decimal digits from the integer arguments (the "+" sign is replaced with '-' if the argument is negative 00113 *** 00114 *** 00115 **/ 00116 inline char digit(int i, int n) 00117 { 00118 int d=1; 00119 char c; 00120 if ( i<0 ) i *= -1; 00121 while(n--) d *= 10; 00122 c = '0' + ((i/d) % 10); 00123 return c; 00124 } 00125 00126 void LaosDisplay::ShowScreen(const char *l, int *arg, char *s) 00127 { 00128 char c, next=0,surpress=1; 00129 char str[128],*p; 00130 p = str; 00131 *p++ = _I2C_HOME; 00132 while ( *l ) 00133 { 00134 c=*l; 00135 switch ( c ) 00136 { 00137 case '$': 00138 if (s != NULL && *s) 00139 c = *s++; 00140 else 00141 c = ' '; 00142 break; 00143 00144 case '+': 00145 if (arg != NULL && *arg < 0) 00146 c = '-'; 00147 else 00148 c = '+'; 00149 break; 00150 00151 case '0': next=1; surpress=0; 00152 case '1': case '2': case '3': case '4': 00153 case '5': case '6': case '7': case '8': case '9': 00154 char d = ' '; 00155 if (arg != NULL ) 00156 { 00157 d = digit(*arg, *l-'0'); 00158 if ( d == '0' && surpress ) // supress leading zeros 00159 d = ' '; 00160 else 00161 surpress = 0; 00162 if ( next && arg != NULL ) // take next numeric argument 00163 { 00164 arg++; 00165 next=0; 00166 surpress=1; 00167 } 00168 } 00169 c = d; 00170 break; 00171 } 00172 *p++ = c; 00173 l++; 00174 } 00175 *p=0; 00176 write(str); 00177 } 00178 00179 // EOF
Generated on Tue Jul 12 2022 21:03:03 by
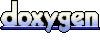