
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
EthConfig.cpp
00001 /* 00002 * EthSetup.cpp 00003 * Setup ethernet interface, based on config file 00004 * Read IP, Gateway, DNS and port for server. 00005 * if IP is not defined, or dhcp is set, use dhcp for Ethernet 00006 * 00007 * Copyright (c) 2011 Peter Brier 00008 * 00009 * This file is part of the LaOS project (see: http://wiki.protospace.nl/index.php/LaOS) 00010 * 00011 * LaOS is free software: you can redistribute it and/or modify 00012 * it under the terms of the GNU General Public License as published by 00013 * the Free Software Foundation, either version 3 of the License, or 00014 * (at your option) any later version. 00015 * 00016 * LaOS is distributed in the hope that it will be useful, 00017 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 * MERCHANTABILITY or FpITNESS FOR A PARTICULAR PURPOSE. See the 00019 * GNU General Public License for more details. 00020 * 00021 * You should have received a copy of the GNU General Public License 00022 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00023 * 00024 */ 00025 #include "EthConfig.h" 00026 00027 // Ethernet connection status inputs (from phy) and outputs (to leds) 00028 #define ETH_LINK (1<<25) 00029 #define ETH_SPEED (1<<26) 00030 PortIn eth_conn(Port1, ETH_LINK | ETH_SPEED); // p25: link, 0=connected, 1=NC, p26: speed, 0=100mbit, 1=10mbit 00031 00032 00033 /** 00034 *** Return Speed status, true==100mbps 00035 **/ 00036 bool EthSpeed(void) 00037 { 00038 int s = eth_conn.read(); 00039 return !(s & ETH_SPEED) && !(s & ETH_LINK); 00040 } 00041 00042 /** 00043 *** Return Link status, true==connected 00044 **/ 00045 bool EthLink(void) 00046 { 00047 int s = eth_conn.read(); 00048 return !(s & ETH_LINK); 00049 } 00050 00051 #define IP(x) IpAddr(x[0], x[1], x[2], x[3]) 00052 00053 00054 /** 00055 *** EthConfig 00056 **/ 00057 EthernetNetIf * EthConfig() 00058 { 00059 EthernetNetIf *eth; 00060 if ( cfg->dhcp ) 00061 { 00062 printf("DHCP...\n"); 00063 eth = new EthernetNetIf(); 00064 } 00065 else 00066 { 00067 printf("FIXED IP...\n"); 00068 eth = new EthernetNetIf(IP(cfg->ip), IP(cfg->nm), IP(cfg->gw), IP(cfg->dns)); 00069 } 00070 printf("Ethernet Setup...\n"); 00071 if ( eth->setup() == ETH_TIMEOUT ) 00072 { 00073 printf("Timeout!\n"); 00074 delete eth; 00075 eth = new EthernetNetIf(IP(cfg->ip), IP(cfg->nm), IP(cfg->gw), IP(cfg->dns)); 00076 } 00077 00078 IpAddr myip = eth->getIp(); 00079 cfg->ip[0] = myip[0]; 00080 cfg->ip[1] = myip[1]; 00081 cfg->ip[2] = myip[2]; 00082 cfg->ip[3] = myip[3]; 00083 00084 printf("mbed IP Address is %d.%d.%d.%d\r\n", myip[0], myip[1], myip[2], myip[3]); 00085 return eth; 00086 }
Generated on Tue Jul 12 2022 21:03:02 by
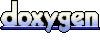