
Dependencies: EthernetNetIf NetServices mbed HTTPServer
fonctions.cpp
00001 #include "stdio.h" 00002 #include "time.h" 00003 #include "stdlib.h" 00004 #include "string.h" 00005 #include "fonctions.h" 00006 00007 // Fonctions permettant de travailler sur les chaines de caractere (source: http://nicolasj.developpez.com/articles/libc/string/#LII-J) 00008 00009 //Fournit l'index d'une sous chaine 00010 int str_istr (const char *cs, const char *ct) { 00011 int index = -1; 00012 00013 if (cs != NULL && ct != NULL) { 00014 char *ptr_pos = NULL; 00015 00016 ptr_pos = (char*)strstr(cs, ct); 00017 if (ptr_pos != NULL) { 00018 index = ptr_pos - cs; 00019 } 00020 } 00021 return index; 00022 } 00023 00024 //Extrait une sous chaine en fonction d'indices limites 00025 char *str_sub (const char *s, unsigned int start, unsigned int end) { 00026 char *new_s = NULL; 00027 00028 if (s != NULL && start < end) { 00029 00030 new_s =(char*) malloc (sizeof (*new_s) * (end - start + 2)); 00031 if (new_s != NULL) { 00032 int i; 00033 00034 for (i = start; i <= end; i++) { 00035 new_s[i-start] = s[i]; 00036 } 00037 new_s[i-start] = '\0'; 00038 } else { 00039 fprintf (stderr, "Memoire insuffisante\n"); 00040 exit (EXIT_FAILURE); 00041 } 00042 } 00043 return new_s; 00044 } 00045 00046 // Extrait en plusieurs sous chaines en fonction d'une chaine de s�paration 00047 char **str_split (char *s, const char *ct) { 00048 char **tab = NULL; 00049 00050 if (s != NULL && ct != NULL) { 00051 int i; 00052 char *cs = NULL; 00053 size_t size = 1; 00054 00055 for (i=0;(cs =(char *) strtok (s, ct)); i++) { 00056 if (size <= i + 1) { 00057 void *tmp = NULL; 00058 00059 00060 size <<= 1; 00061 tmp = realloc (tab, sizeof (*tab) * size); 00062 if (tmp != NULL) { 00063 tab =(char**) tmp; 00064 } else { 00065 fprintf (stderr, "Memoire insuffisante\n"); 00066 free (tab); 00067 tab = NULL; 00068 exit (EXIT_FAILURE); 00069 } 00070 } 00071 00072 tab[i] = cs; 00073 s = NULL; 00074 } 00075 tab[i] = NULL; 00076 } 00077 return tab; 00078 }
Generated on Sat Jul 16 2022 09:37:44 by
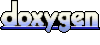