
Starter firmware project for the Imperial College EEE SWITCH lab
Dependencies: Adafruit_GFX mbed
main.cpp
00001 #include "mbed.h" 00002 #include "Adafruit_SSD1306.h" 00003 00004 //Switch input definition 00005 #define SW_PIN p22 00006 00007 //Sampling period for the switch oscillator (us) 00008 #define SW_PERIOD 20000 00009 00010 //Display interface pin definitions 00011 #define D_MOSI_PIN p5 00012 #define D_CLK_PIN p7 00013 #define D_DC_PIN p8 00014 #define D_RST_PIN p9 00015 #define D_CS_PIN p10 00016 00017 //an SPI sub-class that sets up format and clock speed 00018 class SPIPreInit : public SPI 00019 { 00020 public: 00021 SPIPreInit(PinName mosi, PinName miso, PinName clk) : SPI(mosi,miso,clk) 00022 { 00023 format(8,3); 00024 frequency(2000000); 00025 }; 00026 }; 00027 00028 //Interrupt Service Routine prototypes (functions defined below) 00029 void sedge(); 00030 void tout(); 00031 00032 //Output for the alive LED 00033 DigitalOut alive(LED1); 00034 00035 //External interrupt input from the switch oscillator 00036 InterruptIn swin(SW_PIN); 00037 00038 //Switch sampling timer 00039 Ticker swtimer; 00040 00041 //Registers for the switch counter, switch counter latch register and update flag 00042 volatile uint16_t scounter=0; 00043 volatile uint16_t scount=0; 00044 volatile uint16_t update=0; 00045 00046 //Initialise SPI instance for communication with the display 00047 SPIPreInit gSpi(D_MOSI_PIN,NC,D_CLK_PIN); //MOSI,MISO,CLK 00048 00049 //Initialise display driver instance 00050 Adafruit_SSD1306_Spi gOled1(gSpi,D_DC_PIN,D_RST_PIN,D_CS_PIN,64,128); //SPI,DC,RST,CS,Height,Width 00051 00052 int main() { 00053 //Initialisation 00054 gOled1.setRotation(2); //Set display rotation 00055 00056 //Attach switch oscillator counter ISR to the switch input instance for a rising edge 00057 swin.rise(&sedge); 00058 00059 //Attach switch sampling timer ISR to the timer instance with the required period 00060 swtimer.attach_us(&tout, SW_PERIOD); 00061 00062 //Write some sample text 00063 gOled1.printf("%ux%u OLED Display\r\n", gOled1.width(), gOled1.height()); 00064 00065 //Main loop 00066 while(1) 00067 { 00068 //Has the update flag been set? 00069 if (update) { 00070 //Clear the update flag 00071 update = 0; 00072 00073 //Set text cursor 00074 gOled1.setTextCursor(0,0); 00075 00076 //Write the latest switch osciallor count 00077 gOled1.printf("\n%05u ",scount); 00078 00079 //Copy the display buffer to the display 00080 gOled1.display(); 00081 00082 //Toggle the alive LED 00083 alive = !alive; 00084 } 00085 00086 00087 } 00088 } 00089 00090 00091 //Interrupt Service Routine for rising edge on the switch oscillator input 00092 void sedge() { 00093 //Increment the edge counter 00094 scounter++; 00095 } 00096 00097 //Interrupt Service Routine for the switch sampling timer 00098 void tout() { 00099 //Read the edge counter into the output register 00100 scount = scounter; 00101 //Reset the edge counter 00102 scounter = 0; 00103 //Trigger a display update in the main loop 00104 update = 1; 00105 } 00106
Generated on Sat Jul 16 2022 01:11:21 by
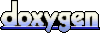