
Library and demo program for MS4525DO differential pressure sensor based pitot tube, using I2C interface
Embed:
(wiki syntax)
Show/hide line numbers
MS4525DO.h
00001 00002 #ifndef MS4525DO_H 00003 #define MS4525DO_H 00004 00005 #include "mbed.h" 00006 00007 #define I2C_ADDRESS_MS4525DO 0x28 /**< 7-bit address =0x28. 8-bit is 0x50. Depends on the order code (this is for code "I") */ 00008 00009 /* Register address */ 00010 #define ADDR_READ_MR 0x00 /* write to this address to start conversion */ 00011 00012 // MS4525D sensor full scale range and units 00013 const int16_t MS4525FullScaleRange = 1; // 1 psi 00014 00015 // MS4525D Sensor type (A or B) comment out the wrong type assignments 00016 // Type A (10% to 90%) 00017 const int16_t MS4525MinScaleCounts = 1638; 00018 const int16_t MS4525FullScaleCounts = 14746; 00019 00020 const int16_t MS4525Span=MS4525FullScaleCounts-MS4525MinScaleCounts; 00021 00022 //MS4525D sensor pressure style, differential or otherwise. Comment out the wrong one. 00023 //Differential 00024 const int16_t MS4525ZeroCounts=(MS4525MinScaleCounts+MS4525FullScaleCounts)/2; 00025 00026 00027 class MS4525DO 00028 { 00029 public: 00030 // instance methods 00031 // explicit I2C pin names 00032 MS4525DO(PinName sda, PinName sck, char slave_adr = I2C_ADDRESS_MS4525DO); 00033 00034 // pin names as an object (ideal when sharing the bus) 00035 MS4525DO(I2C &i2c_obj, char slave_adr = I2C_ADDRESS_MS4525DO); 00036 00037 virtual ~MS4525DO(); 00038 00039 // public functions 00040 void initialize(void); 00041 int measure(void); // returns status of measurement 00042 float getPSI(void); // returns the PSI of last measurement 00043 float getTemperature(void); // returns temperature of last measurement 00044 float getAirSpeed(void); // calculates and returns the airspeed 00045 int calibrate(void); // attempts to calibrate and returns a status code 00046 00047 00048 private: 00049 I2C *i2c_p; 00050 I2C &i2c; 00051 char address; 00052 char _status; 00053 float psi; 00054 float temperature; 00055 float airspeed; 00056 uint16_t P_dat; // 14 bit pressure data 00057 uint16_t T_dat; // 11 bit temperature data 00058 00059 // private functions 00060 int collect(void); 00061 char fetch_pressure(uint16_t &P_dat, uint16_t &T_dat); 00062 00063 00064 }; // end of the class 00065 00066 00067 /** airspeed scaling factors; out = (in * Vscale) + offset */ 00068 struct airspeed_scale { 00069 float offset_pa; 00070 float scale; 00071 }; 00072 00073 #endif
Generated on Tue Jul 12 2022 21:49:13 by
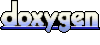