
QWERTY Keypad Touchscreen LCD Demo - MBED + SmartGPU2 board
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /**************************************************************************************/ 00002 /**************************************************************************************/ 00003 /*SMARTGPU2 intelligent embedded graphics processor unit 00004 those examples are for using the SMARTGPU2 with the mbed microcontoller, just connect tx,rx,and reset 00005 Board: 00006 http://www.vizictechnologies.com/ 00007 00008 www.vizictechnologies.com 00009 Vizic Technologies copyright 2014 */ 00010 /**************************************************************************************/ 00011 /**************************************************************************************/ 00012 00013 #include "mbed.h" 00014 #include "SMARTGPU2.h" 00015 00016 SMARTGPU2 lcd(TXPIN,RXPIN,RESETPIN); //create our object called "lcd" 00017 00018 //KEYBOARD DEFINITIONS 00019 #define LETTERSLOWER 0 00020 #define LETTERSUPPER 1 00021 #define NUMBERS 2 00022 #define SPECCHAR 3 00023 #define KEYBOARD_X_SIZE LCD_WIDTH 00024 #define KEYBOARD_Y_SIZE KEYBOARD_X_SIZE/2 00025 #define KEY_Y_TOP MAX_Y_LANDSCAPE - KEYBOARD_Y_SIZE //at the bottom of screen 00026 #define KEYXSIZE KEYBOARD_X_SIZE/10 //10 - columns 00027 #define KEYYSIZE KEYBOARD_Y_SIZE/4 //4 - rows 00028 00029 //keys definitions 00030 #define SPACE ' ' 00031 #define OK 0x01 00032 #define DEL 0x02 00033 #define TYPE 0x03 00034 #define KEYCASE 0x04 00035 00036 //Keyboards type data, row1: 10 characters, row2: 9 characters, row3: 7 characters 00037 const char lettL[]= {"qwertyuiopasdfghjklzxcvbnm"}; //10-9-7 lower case 00038 const char lettU[]= {"QWERTYUIOPASDFGHJKLZXCVBNM"}; //10-9-7 upper case 00039 const char num []= {"1234567890-/:;()&@\"_.,?!'*"};//10-9-7 00040 const char spc []= {"[]{}#%^*+=:|~<>$-/\\_.,?!'*"};//10-9-7 00041 const char* keyboards[4]={lettL, lettU, num, spc}; 00042 00043 /*********************************************************/ 00044 void drawSingleKey(char key, char keyboardType, ACTIVE state){ //draws the received key as "state"(SELECTED or DESELECTED) 00045 unsigned int i=0; 00046 char *data = (char*)keyboards[keyboardType]; 00047 char letter[2]={key,0}; 00048 00049 //special case when key is ' '(space) or 0x01 "enter" or 0x02 "del" or 0x03 "type" or 0x04 "keycase" 00050 if(key == ' ' || key <= KEYCASE){ 00051 switch(key){ 00052 case ' ': //space 00053 lcd.objButton(KEYXSIZE+(KEYXSIZE/2), KEY_Y_TOP+(KEYYSIZE*3), (7*KEYXSIZE+(KEYXSIZE/2))+KEYXSIZE-1, KEY_Y_TOP+(KEYYSIZE*4)-1, state, "space"); 00054 break; 00055 case OK: //OK 00056 lcd.objButton(8*KEYXSIZE+(KEYXSIZE/2), KEY_Y_TOP+(KEYYSIZE*3), KEYBOARD_X_SIZE-1, KEY_Y_TOP+(KEYYSIZE*4)-1, state, "OK"); 00057 break; 00058 case DEL: //delete 00059 lcd.objButton(8*KEYXSIZE+(KEYXSIZE/2), KEY_Y_TOP+(KEYYSIZE*2), (8*KEYXSIZE+(KEYXSIZE/2))+KEYXSIZE-1, KEY_Y_TOP+(KEYYSIZE*3), state, "del"); 00060 break; 00061 case TYPE: //keyboard type 00062 lcd.objButton(KEYXSIZE/2, KEY_Y_TOP+(KEYYSIZE*2), (KEYXSIZE/2)+KEYXSIZE-1, KEY_Y_TOP+(KEYYSIZE*3), state, "type"); 00063 break; 00064 case KEYCASE: //letters upper/lower case 00065 lcd.objButton(0, KEY_Y_TOP+(KEYYSIZE*3), KEYXSIZE+(KEYXSIZE/2)-1, KEY_Y_TOP+(KEYYSIZE*4)-1, state, "case"); 00066 break; 00067 default: //0x00 none 00068 break; 00069 } 00070 return; 00071 } 00072 //any other key case 00073 for(i=0;i<26;i++){ //search for the key in the received keyboardType data array 00074 if(key == data[i]) break; 00075 } 00076 if(i<10){ 00077 lcd.objButton(i*KEYXSIZE, KEY_Y_TOP, (i*KEYXSIZE)+KEYXSIZE-1, KEY_Y_TOP+KEYYSIZE, state, letter); 00078 }else if(i<19){ 00079 i-=10; 00080 lcd.objButton(i*KEYXSIZE+(KEYXSIZE/2), KEY_Y_TOP+KEYYSIZE, (i*KEYXSIZE+(KEYXSIZE/2))+KEYXSIZE-1, KEY_Y_TOP+(KEYYSIZE*2), state, letter); 00081 }else if(i<26){ 00082 i-=18; 00083 lcd.objButton(i*KEYXSIZE+(KEYXSIZE/2), KEY_Y_TOP+(KEYYSIZE*2), (i*KEYXSIZE+(KEYXSIZE/2))+KEYXSIZE-1, KEY_Y_TOP+(KEYYSIZE*3), state, letter); 00084 } 00085 } 00086 00087 /*********************************************************/ 00088 void drawAllKeyboard(char keyboardType){ 00089 unsigned int i=0; 00090 char *data = (char*)keyboards[keyboardType]; 00091 00092 for(i=0;i<26;i++){ //go through all keyboard data 00093 drawSingleKey(*data++, keyboardType, DESELECTED); 00094 } 00095 //draw special keys 00096 drawSingleKey(TYPE, keyboardType, DESELECTED); 00097 drawSingleKey(DEL, keyboardType, DESELECTED); 00098 drawSingleKey(KEYCASE, keyboardType, DESELECTED); 00099 drawSingleKey(SPACE, keyboardType, DESELECTED); 00100 drawSingleKey(OK, keyboardType, DESELECTED); 00101 } 00102 00103 /*********************************************************/ 00104 char getKeyTouch(char keyboardType){ //ask for a touch and if VALID inside the keyboard returns the touched key 00105 char *data = (char*)keyboards[keyboardType]; 00106 POINT p; 00107 00108 if(lcd.touchScreen(&p) == VALID){ //ask for touch, if VALID 00109 if(p.y > KEY_Y_TOP && p.y< (KEY_Y_TOP+KEYBOARD_Y_SIZE)){ //if touch inside keyboard 00110 p.y -= KEY_Y_TOP; //substract 00111 p.y /= (KEYBOARD_Y_SIZE/4); //obtain row 00112 //switch with the obtained row 00113 p.x--; 00114 switch(p.y){ 00115 case 0: //1st row 00116 p.x /= KEYXSIZE; //obtain column 00117 break; 00118 case 1: //2nd row 00119 p.x -= (KEYXSIZE/2); 00120 p.x /= KEYXSIZE; //obtain column 00121 p.x += 10; 00122 break; 00123 case 2: //3rd row 00124 p.x -= (KEYXSIZE/2); 00125 p.x /= KEYXSIZE; //obtain column 00126 p.x += 18; 00127 if(p.x==18) return TYPE; 00128 if(p.x==26) return DEL; 00129 break; 00130 default: //4rt row 00131 p.x -= (KEYXSIZE/2); 00132 p.x /= KEYXSIZE; //obtain column 00133 if(p.x==0) return KEYCASE; 00134 if(p.x>=8) return OK; 00135 return ' '; 00136 break; 00137 } 00138 return *(data+p.x); 00139 } 00140 } 00141 return 0; 00142 } 00143 00144 /***************************************************/ 00145 /***************************************************/ 00146 void initializeSmartGPU2(void){ //Initialize SMARTGPU2 Board 00147 lcd.reset(); //physically reset SMARTGPU2 00148 lcd.start(); //initialize the SMARTGPU2 processor 00149 } 00150 00151 /***************************************************/ 00152 /***************************************************/ 00153 /***************************************************/ 00154 /***************************************************/ 00155 int main() { 00156 unsigned int currentX=5, lastX=5, currentY=5; 00157 char key = 0, currentKeyboard = LETTERSLOWER; 00158 00159 initializeSmartGPU2(); //Init communication with SmartGPU2 board 00160 00161 lcd.baudChange(BAUD7); //set a fast baud! for fast drawing 00162 lcd.drawGradientRect(0, 0, MAX_X_LANDSCAPE, MAX_Y_LANDSCAPE, MAGENTA, BLACK, VERTICAL); //draw a background 00163 00164 lcd.drawRectangle(5, 5, MAX_X_LANDSCAPE-5, KEY_Y_TOP-5, WHITE, FILL); //draw text background 00165 lcd.setTextColour(BLACK); //set text colour as black 00166 lcd.setTextSize(FONT2); //set text size FONT2 00167 00168 drawAllKeyboard(currentKeyboard); //draw all keyboard 00169 00170 while(1){ 00171 while((key = getKeyTouch(currentKeyboard)) == 0x00); //loop until get a valid key 00172 //once obtained a valid key 00173 if(key!=OK && key!=DEL && key!=TYPE && key!=KEYCASE){ //only print if key is not special key 00174 lcd.putLetter(lastX, currentY, key, ¤tX); //print key on lastX and save updated value in currentX 00175 if(currentX<=lastX){ //if currentX couldn't advance, means end of X row 00176 currentY += 20; //jump 1 row in Y axis 00177 if(currentY >= (KEY_Y_TOP-5)){ //if we reach the start of the keyboard 00178 currentY=5; 00179 lcd.drawRectangle(5, 5, MAX_X_LANDSCAPE-5, KEY_Y_TOP-5, WHITE, FILL); //draw text background 00180 } 00181 lastX=5; //reset lastX 00182 lcd.putLetter(lastX, currentY, key, ¤tX); //print key on new lastX and currentY 00183 } 00184 lastX=currentX; //get new value 00185 }else{ 00186 switch(key){ 00187 case TYPE: 00188 if(currentKeyboard == LETTERSLOWER || currentKeyboard == LETTERSUPPER) currentKeyboard = NUMBERS; //go to next type 00189 else if(currentKeyboard == NUMBERS) currentKeyboard = SPECCHAR; 00190 else if (currentKeyboard == SPECCHAR) currentKeyboard = LETTERSLOWER; 00191 drawAllKeyboard(currentKeyboard); //update all keyboard 00192 break; 00193 case DEL: 00194 lcd.drawRectangle(5, 5, MAX_X_LANDSCAPE-5, KEY_Y_TOP-5, WHITE, FILL); //draw text background 00195 lastX=5; //reset lastX 00196 currentY=5; //reset currentY 00197 break; 00198 case KEYCASE: 00199 if(currentKeyboard == LETTERSLOWER) currentKeyboard = LETTERSUPPER; 00200 else if(currentKeyboard == LETTERSUPPER) currentKeyboard = LETTERSLOWER; 00201 drawAllKeyboard(currentKeyboard); //update all keyboard 00202 break; 00203 default: //key == OK 00204 //go to main menu - exit keyboard - or any other required action 00205 break; 00206 } 00207 } 00208 //draw the animated key 00209 drawSingleKey(key, currentKeyboard, SELECTED); //draw the obtained key button as SELECTED 00210 delay(200); //wait 200ms with key as SELECTED 00211 drawSingleKey(key, currentKeyboard, DESELECTED); //draw the obtained key button as DESELECTED 00212 } 00213 }
Generated on Thu Jul 14 2022 15:52:23 by
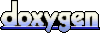