
Compact Flash I/O test
Embed:
(wiki syntax)
Show/hide line numbers
DataTypes.h
00001 #include <stdio.h> 00002 #include <stdarg.h> 00003 #include "ff.h" 00004 00005 #define INLINE_BITPLANE_PUT 00006 #define INLINE_BITPLANE_GET 00007 00008 #ifndef DATATYPES_H_ 00009 #define DATATYPES_H_ 00010 00011 //#define BYTE unsigned char 00012 #define WORD unsigned short 00013 #define DWORD unsigned long 00014 00015 #define SIGNED_BYTE signed char 00016 #define SIGNED_WORD signed short 00017 #define SIGNED_DWORD signed int 00018 00019 //#define CHAR char 00020 00021 #define BOOL BYTE 00022 00023 #ifndef TRUE 00024 #define TRUE 1 00025 #endif 00026 00027 00028 #ifndef FALSE 00029 #define FALSE 0 00030 #endif 00031 00032 #ifndef YEP 00033 #define YEP TRUE 00034 #endif 00035 00036 00037 #ifndef NOPE 00038 #define NOPE FALSE 00039 #endif 00040 00041 00042 #define STACK_FULL -1 00043 #define STACK_EMPTY -2 00044 #define STACK_PUSH_OK 0 00045 00046 #define QUEUE_FULL -1 00047 #define QUEUE_EMPTY -2 00048 #define QUEUE_OK 0 00049 00050 00051 typedef struct{ 00052 00053 BYTE *StackSpace; 00054 WORD Ptr; 00055 WORD Size; 00056 } ByteStack; 00057 00058 typedef struct{ 00059 BYTE *StackSpace; 00060 BYTE Ptr; 00061 BYTE Size; 00062 } BitStack; 00063 00064 typedef struct { 00065 00066 BYTE *BitPlaneSpace; 00067 00068 WORD SizeX; // must be a BYTE aligned 00069 WORD SizeY; 00070 00071 } BitPlane; 00072 00073 00074 typedef struct { 00075 00076 WORD ReadPtr; 00077 WORD WritePtr; 00078 WORD QueueSize; 00079 BYTE *QueueStorage; 00080 00081 } ByteQueue; 00082 00083 00084 00085 #ifdef INLINE_BITPLANE_PUT 00086 inline void BitPlane_Put(BitPlane * BP, WORD X,WORD Y, BOOL Value) 00087 { 00088 WORD Offset; 00089 BYTE Mask; 00090 00091 Offset = (Y * ((BP->SizeX)>>3)) + (X>>3); 00092 Mask = 0x01 << (X & 0x07); 00093 00094 if(Value) 00095 { 00096 BP->BitPlaneSpace[Offset] |= Mask; 00097 } 00098 else 00099 { 00100 BP->BitPlaneSpace[Offset] &= ~Mask; 00101 } 00102 } 00103 #else 00104 void BitPlane_Put(BitPlane * BP, WORD X,WORD Y, BOOL Value); 00105 #endif 00106 00107 #ifdef INLINE_BITPLANE_GET 00108 inline BOOL BitPlane_Get(BitPlane * BP, WORD X,WORD Y) 00109 { 00110 WORD Offset; 00111 BYTE Mask; 00112 00113 Offset = (Y * ((BP->SizeX)>>3)) + (X>>3); 00114 Mask = 0x01 << (X & 0x07); 00115 00116 if((BP->BitPlaneSpace[Offset])&Mask) 00117 { 00118 return TRUE; 00119 } 00120 else 00121 { 00122 return FALSE; 00123 } 00124 } 00125 #else 00126 BOOL BitPlane_Get(BitPlane * BP, WORD X,WORD Y); 00127 #endif 00128 00129 00130 void BitPlane_Clear(BitPlane * BP); 00131 00132 void InitByteQueue(ByteQueue *BQ,WORD Size,BYTE * Storage); 00133 WORD BytesInQueue(ByteQueue *BQ); 00134 SIGNED_WORD ByteDequeue(ByteQueue *BQ,BYTE *Val); 00135 SIGNED_WORD ByteEnqueue(ByteQueue *BQ,BYTE Val); 00136 SIGNED_WORD ByteArrayEnqueue(ByteQueue *BQ,BYTE *Buf,WORD Len); 00137 SIGNED_WORD PrintfEnqueue(ByteQueue *BQ, const char *FormatString,...); 00138 00139 #endif /*DATATYPES_H_*/
Generated on Sat Jul 16 2022 16:37:46 by
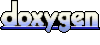