
RX sw for DoorBell - Based on SPIRIT1 & NUCLEO
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 /** 00002 00003 Wireless Door Bell based on SPIRIT1 & NUCLEO boards 00004 RX RX RX 00005 00006 Date: Jan 2018 00007 Version: 1.0 00008 More info are here: 00009 http://www.emcu.eu/wireless-doorbell-based-on-spirit1-nucleo-boards/ 00010 00011 ****************************************************************************** 00012 * @file main.cpp 00013 * @author Rosarium PILA, STMicroelectronics 00014 * @version V1.0.0 00015 * @date June 19th, 2017 00016 * @brief mbed test application for the STMicroelectronics X-NUCLEO-IDB01A4/5 00017 * Spirit1 Expansion Board 00018 ****************************************************************************** 00019 * @attention 00020 * 00021 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00022 * 00023 * Redistribution and use in source and binary forms, with or without modification, 00024 * are permitted provided that the following conditions are met: 00025 * 1. Redistributions of source code must retain the above copyright notice, 00026 * this list of conditions and the following disclaimer. 00027 * 2. Redistributions in binary form must reproduce the above copyright notice, 00028 * this list of conditions and the following disclaimer in the documentation 00029 * and/or other materials provided with the distribution. 00030 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00031 * may be used to endorse or promote products derived from this software 00032 * without specific prior written permission. 00033 * 00034 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00035 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00036 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00037 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00038 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00039 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00040 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00041 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00042 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00043 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ****************************************************************************** 00046 */ 00047 00048 #include "mbed.h" 00049 #include "SimpleSpirit1.h" 00050 00051 #include <stdio.h> 00052 #include <string.h> 00053 00054 #define TEST_STR_LEN (32) 00055 static char send_buf[TEST_STR_LEN] ={'S','P','I','R','I','T','1',' ','H','E','L','L','O',' ','W','O','R','L','D',' ','P','2','P',' ','D','E','M','O'}; 00056 static char read_buf[TEST_STR_LEN] ={'0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0'}; 00057 00058 static SimpleSpirit1 &myspirit = SimpleSpirit1::CreateInstance(D11, D12, D3, D9, D10, D2); /* (SPI_CLK) = (D13:PA5:resistorR7 --> D3:PB3:resistorR4)*/ 00059 00060 static volatile bool rx_done_flag = false; 00061 static volatile bool tx_done_flag = false; 00062 static volatile bool send_data_flag = false; 00063 00064 static DigitalOut TestLED(D5); /* LED of IDS01A4/5 */ 00065 static InterruptIn event(USER_BUTTON); /* Interrupt event to give userinterface to send pkt. */ 00066 00067 /** 00068 * @brief callback_func 00069 * @param callback event 00070 * @retval None. 00071 */ 00072 static void callback_func(int event) 00073 { 00074 if(event == SimpleSpirit1::RX_DONE) 00075 { 00076 rx_done_flag = 1; 00077 } 00078 else if (event == SimpleSpirit1::TX_DONE) 00079 { 00080 tx_done_flag = 1; 00081 } 00082 } 00083 00084 /** 00085 * @brief set_send_data_flag 00086 * @param None 00087 * @retval None 00088 */ 00089 static void set_send_data_flag(void) 00090 { 00091 send_data_flag = 1 ; 00092 } 00093 00094 /** 00095 * @brief send_data 00096 * @param None 00097 * @retval None 00098 */ 00099 static void send_data(void) 00100 { 00101 printf("\r\n***Sending a packet***"); 00102 00103 while(myspirit.is_receiving()); /* wait for ongoing RX ends */ 00104 00105 size_t curr_len = strlen((const char*)send_buf) + 1; 00106 myspirit.send(send_buf, curr_len); 00107 } 00108 00109 /** 00110 * @brief read_rcvd_data 00111 * @param None 00112 * @retval None 00113 */ 00114 static void read_rcvd_data(void) 00115 { 00116 00117 for(unsigned int flush_count = 0; flush_count < TEST_STR_LEN; flush_count++) read_buf[flush_count] = 0 ;/* clear the read buffer */ 00118 00119 int ret = myspirit.read(read_buf, sizeof(read_buf)); 00120 00121 if(ret == 0) 00122 { 00123 printf("\nNothing to read\n\r"); 00124 return; 00125 } 00126 printf("\r\n***Received a packet***\r\n\rReceived string = '%s' (len=%d) \n\r", read_buf, ret); 00127 00128 wait_ms(100); 00129 } 00130 00131 00132 /** 00133 * @brief main routine 00134 * @param None 00135 * @retval int 00136 */ 00137 int main() 00138 { 00139 TestLED = 0; /* LED off */ 00140 00141 myspirit.attach_irq_callback(callback_func); 00142 00143 myspirit.on(); 00144 00145 printf("\n\r************** RX (X-NUCLEO-IDS01A4/5) **************\r\n"); 00146 printf("Receive a strinbg and back it to Tx\n\r\n\n"); 00147 00148 event.rise(&set_send_data_flag); /*User button interrupt trigger to set send data flag */ 00149 00150 while(1) 00151 { 00152 __WFE(); /* low power in idle condition., waiting for an event */ 00153 00154 if(rx_done_flag) 00155 { 00156 rx_done_flag = false; 00157 read_rcvd_data(); 00158 00159 if(strcmp(send_buf,read_buf) == 0) 00160 { 00161 printf("RxTx OK \n\r"); 00162 TestLED = !TestLED; /* Toggle LED at the receiver */ 00163 } 00164 else 00165 printf("RxTx FAIL \n\r"); 00166 00167 wait_ms(100); 00168 send_data_flag = false; 00169 send_data(); 00170 } 00171 00172 else if (tx_done_flag) 00173 { 00174 tx_done_flag = false; 00175 printf("\r\n***Packet sent ***\r\nSent string ='%s' (len=%d)\n\r", send_buf, strlen((const char*)send_buf) + 1); 00176 } 00177 00178 // Read the red LED status 00179 if(TestLED == 1) 00180 { 00181 int n=0; 00182 for(n=0; n<=6; n++) 00183 { 00184 wait_ms(500); 00185 TestLED = 0; 00186 wait_ms(150); 00187 TestLED = 1; 00188 } 00189 for(n=0; n<=12; n++) 00190 { 00191 wait_ms(250); 00192 TestLED = 0; 00193 wait_ms(100); 00194 TestLED = 1; 00195 } 00196 for(n=0; n<=18; n++) 00197 { 00198 wait_ms(150); 00199 TestLED = 0; 00200 wait_ms(70); 00201 TestLED = 1; 00202 } 00203 TestLED = 0; 00204 } 00205 } 00206 00207 /* unreachable */ 00208 // myspirit.off(); 00209 // return 0; 00210 }
Generated on Fri Jul 22 2022 19:11:01 by
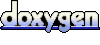