
Remote TX using NUCLEO_L152RE + AUREL RTX-MID-3V (Sub1GHz module). This SW working in conjunction of the RX part that is: Remote RX using NUCLEO_L152RE + AUREL RTX-MID-3V (Sub1GHz module)
main.cpp
00001 /* 00002 00003 TX for remote Thermostat 00004 https://developer.mbed.org/users/emcu/code/0D_NUCLEOL152RE_RemoteIO_TX/file/c11167ef6bd1/main.cpp 00005 00006 By: www.emcu.eu 00007 Date: 04-January-2017 00008 00009 NUCLEO-L152RE and AUREL RTX-MID-3V (433.92 MHz - mod. ASK - 3Vcc) 00010 00011 AUREL RTX-MID-3V 00012 Italian AUREL manual is here: http://www.aurelwireless.com/wp-content/uploads/manuale-uso/650201033G_mu.pdf 00013 English AUREL manual is here: http://www.aurelwireless.com/en/radiomodem-data-transceivers/ 00014 https://www.futurashop.it/index.php?route=product/product&filter_name=RTX-MID-3V&product_id=2788 00015 00016 CONNECTIONS from AUREL RTX-MID-3V to NUCLEO L152RE 00017 PIN1 Antenna 17cm 00018 PIN2 GND 00019 PIN4 TX connected to TX on NUCLEO L152RE 00020 PIN5 Tx/Rx connected to PA_10 on NUCLEO L152RE 00021 PIN6 ENABLE connected to PB_3 on NUCLEO L152RE 00022 PIN7 GND 00023 PIN8 Analog Out not connected 00024 PIN9 RX connected to RX on NUCLEO L152RE 00025 PIN10 VCC connected to 3,3V on NUCLEO L152RE 00026 00027 NOTE: between GND and VCC put a capacitor of 0,1uF and a second capacitor 00028 of 10uF 00029 00030 00031 ********** IMPORTANT IMPORTANT IMPORTANT IMPORTANT ********** 00032 00033 This project use the USART for this reason you must do the below 00034 modifications on NUCLEO-L152RE, more info are here: 00035 http://www.emcu.it/NUCLEOevaBoards/U2andL152/U2andL152.html#USART2_for_communication_with_shield_or 00036 00037 Put a jumper on SB62 and on SB63 00038 Remove a 0 ohm resistor from SB13 and SB14 00039 00040 ATTENTION: 00041 After this modifications, you lost the possibility to use the virtual comm 00042 to connect the NUCLEO to the PC . 00043 00044 ************************************************************* 00045 00046 00047 ********** EXPLANATIONS: 00048 00049 Build two boards one with RX software and another with TX software. 00050 When you press Blue button (and hold it) 00051 on the board_TX, the green LED must turn ON, the same 00052 on the board_RX the green LED must turn ON. 00053 If you release the Blue button (on the board_TX) the green LED must go OFF 00054 on both on the boards. 00055 00056 * 00057 * Permission is hereby granted, free of charge, to any person obtaining a copy 00058 * of this software and associated documentation files (the "Software"), to deal 00059 * in the Software without restriction, including without limitation the rights 00060 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00061 * copies of the Software, and to permit persons to whom the Software is 00062 * furnished to do so, subject to the following conditions: 00063 * 00064 * The above copyright notice and this permission notice shall be included in 00065 * all copies or substantial portions of the Software. 00066 * 00067 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00068 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00069 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00070 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00071 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00072 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00073 * THE SOFTWARE. 00074 00075 00076 */ 00077 00078 00079 #include "mbed.h" 00080 00081 Serial pc(SERIAL_TX, SERIAL_RX); // tx, rx 00082 DigitalOut RTX_DIR(PA_10); // 1 == TX 0 == RX 00083 DigitalOut RTX_Enable(PB_3); // 1 == RTX enable 0 == RTX disable 00084 00085 DigitalOut myled(LED1); // This LED is on NUCLEO-L152RE 00086 DigitalIn BlueButton(USER_BUTTON); // This is Blue-Button and is on NUCLEO-L153RE 00087 00088 #define Pressed 0 00089 #define NotPressed 1 00090 #define Enable 1 00091 #define Disable 0 00092 #define ACK 2 00093 #define OK 1 00094 #define FAIL 0 00095 #define Received 0 00096 #define NotReceived 1 00097 00098 #define ON 1 00099 #define OFF 0 00100 #define TX 1 00101 #define RX 0 00102 00103 #define Baud 1200 // 9600 00104 #define DlyForEndTX 220 // 220 00105 #define MaxReTX 40 // 40 00106 #define DebTime 200 // Debounce time 00107 #define WaitBeforeToDoRX 200 // 100 00108 00109 int Car='\0'; 00110 int RisultatoRX=FAIL; 00111 int MemBlueButton=10; 00112 int MemOkFail=FAIL; 00113 int MemOK=FAIL; 00114 int MemOFF=NotReceived; 00115 int MemON=NotReceived; 00116 00117 int MemBB = NotPressed; 00118 00119 00120 int TestRX_Data(void); 00121 void RTX_StartUp(void); 00122 void RTX_PowerDown(void); 00123 void RTX_PowerON(void); 00124 void RTX_TX(void); 00125 void RTX_RX(void); 00126 00127 int CarStr[3]; 00128 int PuntCar = 0; 00129 00130 void callback() { 00131 // Note: you need to actually read from the serial to clear the RX interrupt 00132 Car = pc.getc(); 00133 if (Car == '#' & PuntCar == 0) 00134 { 00135 PuntCar = 0; 00136 CarStr[PuntCar] = Car; 00137 PuntCar++; 00138 } 00139 else if (PuntCar > 0 & PuntCar < 3) 00140 { 00141 CarStr[PuntCar] = Car; 00142 PuntCar++; 00143 } 00144 } 00145 00146 00147 // 00148 // MAIN ***************************************************************** 00149 // 00150 int main() 00151 { 00152 int n=0; 00153 int Rip=3; 00154 00155 pc.baud(Baud); 00156 pc.attach(&callback, pc.RxIrq); // It is for reading under Interrupt the 00157 // RX from USART 00158 00159 // Start Up of the RTX-MID and put it in RX mode 00160 RTX_StartUp(); 00161 00162 MemBB = Pressed; 00163 MemOFF = NotReceived; 00164 MemON = NotReceived; 00165 00166 while (1) 00167 { 00168 // Test the status of the Blue button (TX) ************************ 00169 if ((BlueButton == Pressed) && (MemBB == NotPressed)) 00170 { 00171 wait_ms(DebTime); // Debounce 00172 if (BlueButton == Pressed) 00173 { 00174 MemBB = Pressed; 00175 n=0; 00176 while (TestRX_Data() != OK) 00177 { 00178 RTX_TX(); // RTX-MID on TX mode 00179 // Send Message 00180 pc.printf("#1@"); // Send Command ON 00181 wait_ms(DlyForEndTX); 00182 RTX_RX(); // RTX-MID on RX mode 00183 wait_ms(WaitBeforeToDoRX); // 100 00184 for (Rip=0; Rip<40; Rip++) // 40 00185 { 00186 if (TestRX_Data() == OK) 00187 { 00188 myled = ON; 00189 goto EndBBpressed; 00190 // break; 00191 } 00192 } 00193 n++; 00194 if (n > MaxReTX) 00195 break; 00196 } 00197 } 00198 // myled = ON; 00199 wait(2); 00200 pc.baud(Baud); 00201 pc.attach(&callback, pc.RxIrq); // It is for reading under Interrupt the 00202 // RX from USART 00203 RTX_StartUp(); 00204 wait(1); 00205 MemBB = NotPressed; 00206 EndBBpressed: 00207 RTX_RX(); // RTX-MID on RX mode 00208 } 00209 00210 00211 if ((BlueButton == NotPressed) && (MemBB == Pressed)) 00212 { 00213 wait_ms(DebTime); // Debounce 00214 if (BlueButton == NotPressed) 00215 { 00216 MemBB = NotPressed; 00217 n=0; 00218 while (TestRX_Data() != ACK) 00219 { 00220 RTX_TX(); // RTX-MID on TX mode 00221 // Send Message 00222 pc.printf("#2@"); // Send Command OFF 00223 wait_ms(DlyForEndTX); 00224 RTX_RX(); // RTX-MID on RX mode 00225 wait_ms(WaitBeforeToDoRX); // 100 00226 for (Rip=0; Rip<40; Rip++) // 40 00227 { 00228 if (TestRX_Data() == ACK) 00229 { 00230 myled = OFF; 00231 goto EndBBnotpressed; 00232 // break; 00233 } 00234 } 00235 n++; 00236 if (n > MaxReTX) 00237 break; 00238 } 00239 } 00240 // myled = OFF; 00241 wait(2); 00242 pc.baud(Baud); 00243 pc.attach(&callback, pc.RxIrq); // It is for reading under Interrupt the 00244 // RX from USART 00245 RTX_StartUp(); 00246 wait(1); 00247 MemBB = Pressed; 00248 EndBBnotpressed: 00249 RTX_RX(); // RTX-MID on RX mode 00250 } 00251 00252 // END Test the status of the Blue button (TX) ************************ 00253 00254 RTX_RX(); // RTX-MID on RX mod 00255 RisultatoRX = TestRX_Data(); 00256 if (RisultatoRX == OK) // OK==ON 00257 myled = ON; 00258 RisultatoRX = TestRX_Data(); 00259 if (RisultatoRX == ACK) // ACK==OFF 00260 myled = OFF; 00261 00262 00263 } 00264 } 00265 00266 // 00267 // END MAIN ************************************************************* 00268 // 00269 00270 00271 // 00272 // Decode Message 00273 // 00274 // REMEMBER: 00275 // before to use this function must put in RX mode the RTX 00276 // 00277 int TestRX_Data(void) 00278 { 00279 int OkFail=0; // 1==OK==ON 2==ACK==OFF 00280 00281 if (CarStr[0]=='#' & CarStr[1]=='1' & CarStr[2]=='@') 00282 OkFail=1; // OK==ON 00283 else if (CarStr[0]=='#' & CarStr[1]=='2' & CarStr[2]=='@') 00284 OkFail=2; // ACK==OFF 00285 else; 00286 00287 if (PuntCar >= 3) // Reset the CarStr & PuntCar 00288 { 00289 CarStr[0] = '0'; 00290 CarStr[1] = '1'; 00291 CarStr[2] = '2'; 00292 PuntCar = 0; 00293 } 00294 00295 return OkFail; 00296 } 00297 00298 void RTX_StartUp(void) 00299 { 00300 RTX_Enable = Disable; 00301 wait_ms(10); 00302 RTX_Enable = Enable; 00303 wait_ms(1); 00304 RTX_DIR = TX; // RTX-MID in TX mode 00305 wait_ms(1); // Wait the stabilizzation of the transceiver 00306 RTX_Enable = Disable; 00307 wait_ms(10); 00308 RTX_Enable = Enable; 00309 wait_ms(1); 00310 RTX_DIR = RX; // RTX-MID in RX mode 00311 wait_ms(1); // Wait the stabilizzation of the transceiver 00312 RTX_Enable = Disable; 00313 wait_ms(1); 00314 RTX_Enable = Enable; 00315 wait_ms(1); 00316 } 00317 00318 void RTX_PowerDown(void) 00319 { 00320 RTX_Enable = Disable; 00321 wait_ms(10); 00322 } 00323 00324 void RTX_PowerON(void) 00325 { 00326 RTX_Enable = Disable; 00327 wait_ms(1); 00328 } 00329 00330 void RTX_TX(void) 00331 { 00332 // RTX_Enable = Disable; 00333 // wait_ms(10); 00334 // RTX_Enable = Enable; 00335 // wait_ms(1); 00336 RTX_DIR = TX; // RTX-MID in TX mode 00337 wait_us(400); // Wait the stabilizzation of the transceiver 00338 } 00339 00340 void RTX_RX(void) 00341 { 00342 // RTX_Enable = Disable; 00343 // wait_ms(10); 00344 // RTX_Enable = Enable; 00345 // wait_ms(1); 00346 // RTX_DIR = TX; // RTX-MID in TX mode 00347 // wait_ms(1); // Wait the stabilizzation of the transceiver 00348 RTX_DIR = RX; // RTX-MID in RX mode 00349 wait_us(40); 00350 RTX_Enable = Disable; 00351 wait_us(20); 00352 RTX_Enable = Enable; 00353 wait_us(200); 00354 } 00355 00356
Generated on Mon Jul 18 2022 19:16:44 by
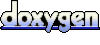