
Remote RX using NUCLEO_L152RE + AUREL RTX-MID-3V (Sub1GHz module). This SW working in conjunction of the TX part that is: Remote TX using NUCLEO_L152RE + AUREL RTX-MID-3V (Sub1GHz module)
main.cpp
00001 /* 00002 00003 RX for remote Thermostat 00004 00005 By: www.emcu.eu 00006 Date: 04-January-2017 00007 00008 NUCLEO-L152RE and AUREL RTX-MID-3V (433.92 MHz - mod. ASK - 3Vcc) 00009 00010 AUREL RTX-MID-3V 00011 Italian AUREL manual is here: http://www.aurelwireless.com/wp-content/uploads/manuale-uso/650201033G_mu.pdf 00012 English AUREL manual is here: http://www.aurelwireless.com/en/radiomodem-data-transceivers/ 00013 https://www.futurashop.it/index.php?route=product/product&filter_name=RTX-MID-3V&product_id=2788 00014 00015 CONNECTIONS from AUREL RTX-MID-3V to NUCLEO L152RE 00016 PIN1 Antenna 17cm 00017 PIN2 GND 00018 PIN4 TX connected to TX on NUCLEO L152RE 00019 PIN5 Tx/Rx connected to PA_10 on NUCLEO L152RE 00020 PIN6 ENABLE connected to PB_3 on NUCLEO L152RE 00021 PIN7 GND 00022 PIN8 Analog Out not connected 00023 PIN9 RX connected to RX on NUCLEO L152RE 00024 PIN10 VCC connected to 3,3V on NUCLEO L152RE 00025 00026 NOTE: between GND and VCC put a capacitor of 0,1uF and a second capacitor 00027 of 10uF 00028 00029 00030 ********** IMPORTANT IMPORTANT IMPORTANT IMPORTANT ********** 00031 00032 This project use the USART for this reason you must do the below 00033 modifications on NUCLEO-L152RE, more info are here: 00034 http://www.emcu.it/NUCLEOevaBoards/U2andL152/U2andL152.html#USART2_for_communication_with_shield_or 00035 00036 Put a jumper on SB62 and on SB63 00037 Remove a 0 ohm resistor from SB13 and SB14 00038 00039 ATTENTION: 00040 After this modifications, you lost the possibility to use the virtual comm 00041 to connect the NUCLEO to the PC . 00042 00043 ************************************************************* 00044 00045 00046 ********** EXPLANATIONS: 00047 00048 Build two boards one with RX software and another with TX software. 00049 When you press Blue button (and hold it) 00050 on the board_TX, the green LED must turn ON, the same 00051 on the board_RX the green LED must turn ON. 00052 If you release the Blue button (on the board_TX) the green LED must go OFF 00053 on both on the boards. 00054 00055 * 00056 * Permission is hereby granted, free of charge, to any person obtaining a copy 00057 * of this software and associated documentation files (the "Software"), to deal 00058 * in the Software without restriction, including without limitation the rights 00059 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00060 * copies of the Software, and to permit persons to whom the Software is 00061 * furnished to do so, subject to the following conditions: 00062 * 00063 * The above copyright notice and this permission notice shall be included in 00064 * all copies or substantial portions of the Software. 00065 * 00066 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00067 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00068 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00069 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00070 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00071 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00072 * THE SOFTWARE. 00073 00074 00075 */ 00076 00077 00078 #include "mbed.h" 00079 00080 Serial pc(SERIAL_TX, SERIAL_RX); // tx, rx 00081 DigitalOut RTX_DIR(PA_10); // 1 == TX 0 == RX 00082 DigitalOut RTX_Enable(PB_3); // 1 == RTX enable 0 == RTX disable 00083 00084 DigitalOut myled(LED1); // This LED is on NUCLEO-L152RE 00085 DigitalIn BlueButton(USER_BUTTON); // This is Blue-Button and is on NUCLEO-L153RE 00086 00087 #define Pressed 0 00088 #define NotPressed 1 00089 #define Enable 1 00090 #define Disable 0 00091 #define ACK 2 00092 #define OK 1 00093 #define FAIL 0 00094 #define Received 0 00095 #define NotReceived 1 00096 00097 #define ON 1 00098 #define OFF 0 00099 #define TX 1 00100 #define RX 0 00101 00102 #define Baud 1200 // 9600 00103 #define DlyForEndTX 220 // 220 00104 #define MaxReTX 40 00105 #define DebTime 100 // Debounce time 00106 00107 int Car='\0'; 00108 int RisultatoRX=FAIL; 00109 int MemBlueButton=10; 00110 int MemOkFail=FAIL; 00111 int MemOK=FAIL; 00112 int MemOFF=NotReceived; 00113 int MemON=NotReceived; 00114 00115 int MemBB = NotPressed; 00116 00117 00118 int TestRX_Data(void); 00119 void RTX_StartUp(void); 00120 void RTX_PowerDown(void); 00121 void RTX_PowerON(void); 00122 void RTX_TX(void); 00123 void RTX_RX(void); 00124 00125 int CarStr[3]; 00126 int PuntCar = 0; 00127 00128 void callback() { 00129 // Note: you need to actually read from the serial to clear the RX interrupt 00130 Car = pc.getc(); 00131 if (Car == '#' & PuntCar == 0) 00132 { 00133 PuntCar = 0; 00134 CarStr[PuntCar] = Car; 00135 PuntCar++; 00136 } 00137 else if (PuntCar > 0 & PuntCar < 3) 00138 { 00139 CarStr[PuntCar] = Car; 00140 PuntCar++; 00141 } 00142 } 00143 00144 00145 // 00146 // MAIN ***************************************************************** 00147 // 00148 int main() 00149 { 00150 int n=0; 00151 00152 pc.baud(Baud); 00153 pc.attach(&callback, pc.RxIrq); // It is for reading under Interrupt the 00154 // RX from USART 00155 00156 // Start Up of the RTX-MID and put it in RX mode 00157 RTX_StartUp(); 00158 00159 MemBB = Pressed; 00160 MemOFF = NotReceived; 00161 MemON = NotReceived; 00162 00163 while (1) 00164 { 00165 00166 // RX mode ************************************************************ 00167 // Decode Message 00168 // 1==OK==ON 2==ACK==OFF 00169 RisultatoRX = TestRX_Data(); 00170 if (RisultatoRX == OK) // OK==ON 00171 { 00172 myled = ON; 00173 // Send ON 00174 // and test if the Message is arrived 00175 n=0; 00176 wait_ms(10); 00177 while (TestRX_Data() != OK) 00178 { 00179 RTX_TX(); // RTX-MID on TX mode 00180 // Send Message 00181 pc.printf("#1@"); // Send Command 00182 wait_ms(DlyForEndTX); 00183 RTX_RX(); // RTX-MID on RX mode 00184 n++; 00185 if (n > MaxReTX) 00186 break; 00187 } 00188 MemOFF = NotReceived; 00189 MemON = Received; 00190 RTX_RX(); // RTX-MID on RX mod 00191 } 00192 00193 if (RisultatoRX == ACK) // ACK==OFF 00194 { 00195 myled = OFF; 00196 // Send OFF 00197 // and test if the Message is arrived 00198 n=0; 00199 wait_ms(10); 00200 while (TestRX_Data() != ACK) 00201 { 00202 RTX_TX(); // RTX-MID on TX mode 00203 // Send Message 00204 pc.printf("#2@"); // Send Command OFF 00205 wait_ms(DlyForEndTX); 00206 RTX_RX(); // RTX-MID on RX mode 00207 n++; 00208 if (n > MaxReTX) 00209 break; 00210 } 00211 MemOFF = Received; 00212 MemON = NotReceived; 00213 RTX_RX(); // RTX-MID on RX mod 00214 } 00215 // END RX mode ******************************************************** 00216 } 00217 } 00218 00219 // 00220 // END MAIN ************************************************************* 00221 // 00222 00223 00224 // 00225 // Decode Message 00226 // 00227 // REMEMBER: 00228 // before to use this function must put in RX mode the RTX 00229 // 00230 int TestRX_Data(void) 00231 { 00232 int OkFail=0; // 1==OK==ON 2==ACK==OFF 00233 00234 if (CarStr[0]=='#' & CarStr[1]=='1' & CarStr[2]=='@') 00235 OkFail=1; // OK==ON 00236 else if (CarStr[0]=='#' & CarStr[1]=='2' & CarStr[2]=='@') 00237 OkFail=2; // ACK==OFF 00238 else; 00239 00240 if (PuntCar >= 3) // Reset the CarStr & PuntCar 00241 { 00242 CarStr[0] = '0'; 00243 CarStr[1] = '1'; 00244 CarStr[2] = '2'; 00245 PuntCar = 0; 00246 } 00247 00248 return OkFail; 00249 } 00250 00251 void RTX_StartUp(void) 00252 { 00253 RTX_Enable = Disable; 00254 wait_ms(10); 00255 RTX_Enable = Enable; 00256 wait_ms(1); 00257 RTX_DIR = TX; // RTX-MID in TX mode 00258 wait_ms(1); // Wait the stabilizzation of the transceiver 00259 RTX_Enable = Disable; 00260 wait_ms(10); 00261 RTX_Enable = Enable; 00262 wait_ms(1); 00263 RTX_DIR = RX; // RTX-MID in RX mode 00264 wait_ms(1); // Wait the stabilizzation of the transceiver 00265 RTX_Enable = Disable; 00266 wait_ms(1); 00267 RTX_Enable = Enable; 00268 wait_ms(1); 00269 } 00270 00271 void RTX_PowerDown(void) 00272 { 00273 RTX_Enable = Disable; 00274 wait_ms(10); 00275 } 00276 00277 void RTX_PowerON(void) 00278 { 00279 RTX_Enable = Disable; 00280 wait_ms(1); 00281 } 00282 00283 void RTX_TX(void) 00284 { 00285 // RTX_Enable = Disable; 00286 // wait_ms(10); 00287 // RTX_Enable = Enable; 00288 // wait_ms(1); 00289 RTX_DIR = TX; // RTX-MID in TX mode 00290 wait_us(400); // Wait the stabilizzation of the transceiver 00291 } 00292 00293 void RTX_RX(void) 00294 { 00295 // RTX_Enable = Disable; 00296 // wait_ms(10); 00297 // RTX_Enable = Enable; 00298 // wait_ms(1); 00299 // RTX_DIR = TX; // RTX-MID in TX mode 00300 // wait_ms(1); // Wait the stabilizzation of the transceiver 00301 RTX_DIR = RX; // RTX-MID in RX mode 00302 wait_us(40); 00303 RTX_Enable = Disable; 00304 wait_us(20); 00305 RTX_Enable = Enable; 00306 wait_us(200); 00307 } 00308 00309
Generated on Thu Jul 28 2022 06:18:01 by
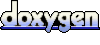