
Solutions for the XBee experiments for LPC812 MAX
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /****************************************************************************** 00002 * Includes 00003 *****************************************************************************/ 00004 #include "mbed.h" 00005 #include "XBee.h" 00006 00007 /****************************************************************************** 00008 * Typedefs and defines 00009 *****************************************************************************/ 00010 00011 // define NODE_IS_COORDINATOR if this board should act 00012 // as XBee coordinator. Make sure it is undefined it the board 00013 // should act as End-Device 00014 #define NODE_IS_COORDINATOR (1) 00015 00016 #define CMD_BTN_MSG (0) 00017 #define CMD_ACK_MSG (1) 00018 00019 /****************************************************************************** 00020 * Local variables 00021 *****************************************************************************/ 00022 00023 static XBee xbee(P0_4, P0_0, P0_17, P0_7); //tx,rx,reset,sleep 00024 00025 static Serial pc(USBTX, USBRX); // tx, rx 00026 00027 // all LEDs active low 00028 static DigitalOut xbeeInitLed(D13); 00029 static DigitalOut remoteBtnLed(D12); 00030 static DigitalOut ackLed(D11); 00031 static DigitalOut led4(D10); 00032 00033 static DigitalIn button(D3); 00034 static bool xbeeIsUp = false; 00035 00036 /****************************************************************************** 00037 * Local functions 00038 *****************************************************************************/ 00039 00040 static void xbeeDeviceUp(void) { 00041 xbeeIsUp = true; 00042 } 00043 00044 static void xbeeDeviceDown(void) { 00045 xbeeIsUp = false; 00046 } 00047 00048 static void xbeeNodeFound(void) { 00049 uint32_t addrHi = 0; 00050 uint32_t addrLo = 0; 00051 uint8_t rssi = 0; 00052 00053 xbee.getRemoteAddress(&addrHi, &addrLo); 00054 xbee.getRssi(&rssi); 00055 00056 pc.printf("XBee: node found: hi=%lx, lo=%lx, rssi=%d\n", 00057 addrHi, addrLo, rssi); 00058 } 00059 00060 static void xbeeTxStat(void) { 00061 00062 uint8_t frameId = 0; 00063 XBee::XBeeTxStatus status = XBee::TxStatusOk; 00064 00065 xbee.getTxStatus(&frameId, &status); 00066 00067 pc.printf("XBee: Tx Stat. Id=%u, status=%d\n", frameId, (int)status); 00068 } 00069 00070 00071 00072 static void xbeeDataAvailable(void) { 00073 char* data = NULL; 00074 char ackMsg = CMD_ACK_MSG; 00075 uint8_t frameId = 0; 00076 uint8_t len = 0; 00077 uint32_t addrHi = 0; 00078 uint32_t addrLo = 0; 00079 uint8_t rssi = 0; 00080 00081 xbee.getRemoteAddress(&addrHi, &addrLo); 00082 xbee.getData(&data, &len); 00083 xbee.getRssi(&rssi); 00084 00085 pc.printf("XBee: Data available: len=%d hi=%lx, lo=%lx, rssi=%d\n", 00086 (int)len, addrHi, addrLo, rssi); 00087 00088 if (len > 0) { 00089 00090 switch(data[0]) { 00091 case CMD_BTN_MSG: 00092 if (len > 1) { 00093 // change led to indicate button state 00094 remoteBtnLed = ((data[1] == 1) ? 1 : 0); 00095 00096 00097 // Send an ACK directly to the remote node (only for 00098 // buttons state == 0) 00099 if (data[1] == 0) { 00100 xbee.send(addrHi, addrLo, &ackMsg, 1, &frameId); 00101 } 00102 } 00103 break; 00104 case CMD_ACK_MSG: 00105 for (int i = 0; i < 3; i++) { 00106 ackLed = 0; 00107 wait_ms(100); 00108 ackLed = 1; 00109 wait_ms(100); 00110 } 00111 break; 00112 } 00113 00114 } 00115 00116 } 00117 00118 static bool xbeeInit() 00119 { 00120 xbee.registerCallback(xbeeDeviceUp, XBee::CbDeviceUp); 00121 xbee.registerCallback(xbeeDeviceDown, XBee::CbDeviceDown); 00122 xbee.registerCallback(xbeeNodeFound, XBee::CbNodeFound); 00123 xbee.registerCallback(xbeeTxStat, XBee::CbTxStat); 00124 xbee.registerCallback(xbeeDataAvailable, XBee::CbDataAvailable); 00125 00126 #ifdef NODE_IS_COORDINATOR 00127 XBee::XBeeError err = xbee.init(XBee::Coordinator, "EAEA"); 00128 #else 00129 XBee::XBeeError err = xbee.init(XBee::EndDevice, "EAEA"); 00130 #endif 00131 if (err != XBee::Ok) { 00132 return false; 00133 } 00134 00135 return true; 00136 00137 } 00138 00139 static void reportInitFailed() { 00140 while (true) { 00141 xbeeInitLed = !xbeeInitLed; 00142 wait_ms(200); 00143 } 00144 } 00145 00146 static void ledInit() { 00147 xbeeInitLed = 0; // turn on 00148 remoteBtnLed = 1; // turn off 00149 ackLed = 1; // turn off 00150 led4 = 1; // turn off 00151 } 00152 00153 00154 /****************************************************************************** 00155 * Main function 00156 *****************************************************************************/ 00157 00158 int main() { 00159 00160 /* 00161 * For this example to work at least two boards with XBee nodes must 00162 * be available; one which is configured as Coordinator and another 00163 * which is configured as End-Device. 00164 * 00165 * LED1 shows the state of XBee initialization. This LED is turned 00166 * on when initialization starts and turned off when initialization 00167 * is ready and the device us up. If initialization fails LED1 00168 * will blink. 00169 * 00170 * When the Push-button on the LPC4088 QuickStart Board is pushed 00171 * a message will be broadcasted. The board(s) that receives the message 00172 * will show the state of the remote board's button by turning LED2 00173 * on or off. 00174 * 00175 * The board that receives the button state message will send back 00176 * an ACK message (only for button down). The board that receives 00177 * the ACK message will blink with LED3. This can be used to verify 00178 * that a board that is not close to you actually received a message 00179 * without having to look at that board's LED2. 00180 */ 00181 00182 int buttonState = 1; 00183 char data[2]; 00184 uint8_t frameId = 0; 00185 00186 // pull-up must be enabled for button 00187 button.mode(PullUp); 00188 00189 ledInit(); 00190 00191 pc.printf("Initializing\n"); 00192 if (!xbeeInit()) { 00193 reportInitFailed(); 00194 } 00195 00196 // Wait until XBee node is reported to be up. 00197 // - For End-device this means that the node is associated with a 00198 // coordinator 00199 // - For a coordinator this means that the node is initialized and ready 00200 pc.printf("Wait until up\n"); 00201 while(!xbeeIsUp) { 00202 xbee.process(); 00203 } 00204 00205 // turn off led to indicate initialization is okay and node is up 00206 xbeeInitLed = 1; 00207 00208 pc.printf("Node is Up\n"); 00209 while (1) { 00210 00211 if (button != buttonState) { 00212 buttonState = button; 00213 00214 data[0] = CMD_BTN_MSG; 00215 data[1] = buttonState; 00216 00217 // Send the button state. In this example we are broadcasting the 00218 // message to all nodes on the same network (PAN ID). In reality 00219 // direct messaging is preferred. 00220 xbee.send(XBEE_ADDRHI_BROADCAST, XBEE_ADDRLO_BROADCAST, 00221 data, 2, &frameId); 00222 } 00223 00224 00225 xbee.process(); 00226 00227 } 00228 }
Generated on Thu Jul 21 2022 14:46:27 by
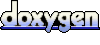