Library with PCF8591 support for the experiments for LPC812 MAX
Dependents: lpc812_exp_solution_analog-in lpc812_exp_solution_7-segment lpc812_exp_solution_7-segment-shift lpc812_exp_solution_pwm ... more
Fork of lpc812_exp_lib_PCF8591 by
PCF8591.h
00001 #ifndef PCF8591_H 00002 #define PCF8591_H 00003 00004 /** 00005 * Interface to the PCF8591 chip (http://www.nxp.com/documents/data_sheet/PCF8591.pdf) 00006 * which has four 8-bit analog inputs and one 8-bit analog output. 00007 * 00008 * @code 00009 * #include "mbed.h" 00010 * 00011 * PCF8591 adc; 00012 * 00013 * int main(void) { 00014 * 00015 * while(1) { 00016 * // read analog value 00017 * int val = adc.read(PCF8591::A0); 00018 * 00019 * // do something with value... 00020 * } 00021 * } 00022 * @endcode 00023 */ 00024 class PCF8591 { 00025 public: 00026 00027 enum AnalogIn { 00028 A0, 00029 A1, 00030 A2, 00031 A3 00032 }; 00033 00034 00035 /** Create an interface to the PCF8591 chip 00036 * 00037 * 00038 * @param sda the I2C SDA pin 00039 * @param scl the I2C SCL pin 00040 * @param i2cAddr the upper 7 bits of the chip's address 00041 */ 00042 PCF8591(PinName sda = P0_10, PinName scl = P0_11, int i2cAddr = 0x9E); 00043 00044 /** Reads one value for the specified analog port 00045 * 00046 * @param port the analog in to read (A0..A3) 00047 * 00048 * @returns 00049 * the value on success (0..255) 00050 * -1 on failure 00051 */ 00052 int read(AnalogIn port); 00053 00054 private: 00055 I2C m_i2c; 00056 int m_addr; 00057 }; 00058 00059 #endif 00060
Generated on Wed Jul 13 2022 15:08:35 by
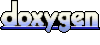