
Example of using a Text LCD with the LPC4088 Experiment Base Board
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /****************************************************************************** 00002 * Includes 00003 *****************************************************************************/ 00004 #include "mbed.h" 00005 #include "TextLCD.h" 00006 00007 /****************************************************************************** 00008 * Typedefs and defines 00009 *****************************************************************************/ 00010 00011 /****************************************************************************** 00012 * Local variables 00013 *****************************************************************************/ 00014 00015 DigitalOut myled(LED1); 00016 00017 SPI spi_lcd(p5, p6, p7); // MOSI, MISO, SCLK 00018 00019 /****************************************************************************** 00020 * Local functions 00021 *****************************************************************************/ 00022 00023 /* 00024 Hardware Setup: 00025 00026 - Jumpers JP1..JP6 should be in position 1-2 00027 - Jumpers in J14 should NOT be inserted 00028 - Jumper J7 should be inserted 00029 - Display in connector J10. Display should be over the base board - not outside 00030 */ 00031 00032 int main() { 00033 TextLCD_SPI lcd(&spi_lcd, p30, TextLCD::LCD16x2); // SPI bus, CS pin, LCD Type ok 00034 00035 lcd.cls(); 00036 lcd.locate(0, 0); 00037 lcd.putc('E'); 00038 lcd.putc('m'); 00039 lcd.putc('b'); 00040 lcd.putc('e'); 00041 lcd.putc('d'); 00042 lcd.putc('d'); 00043 lcd.putc('e'); 00044 lcd.putc('d'); 00045 lcd.putc(' '); 00046 lcd.putc('A'); 00047 lcd.putc('r'); 00048 lcd.putc('t'); 00049 lcd.putc('i'); 00050 lcd.putc('s'); 00051 lcd.putc('t'); 00052 lcd.putc('s'); 00053 lcd.putc('!'); 00054 lcd.setCursor(TextLCD::CurOff_BlkOn); 00055 00056 while(1) { 00057 myled = 1; 00058 wait(0.2); 00059 myled = 0; 00060 wait(0.2); 00061 } 00062 }
Generated on Mon Jul 18 2022 03:55:56 by
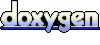