
Graphical demo for the LPC4088 Experiment Base Board with one of the Display Expansion Kits. This program displays a number of dots in a 3D-projected wave.
WaveDemo.h
00001 00002 #ifndef WAVEDEMO_H 00003 #define WAVEDEMO_H 00004 00005 #include "Graphics.h" 00006 #include "GFXFb.h" 00007 00008 #include "EaLcdBoardGPIO.h" 00009 00010 class WaveDemo { 00011 public: 00012 00013 /** Set the address of the frame buffer to use. 00014 * 00015 * It is the content of the frame buffer that is shown on the 00016 * display. All the drawing on the frame buffer can be done 00017 * 'offline' and whenever it should be shown this function 00018 * can be called with the address of the offline frame buffer. 00019 * 00020 * @param pFrameBuf Pointer to the frame buffer, which must be 00021 * 3 times as big as the frame size (for tripple 00022 * buffering). 00023 * dispWidth The width of the display (in pixels). 00024 * dispHeight The height of the display (in pixels). 00025 * loops Number of loops in the demo code. 00026 * delayMs Delay in milliseconds between schreen updates. 00027 * 00028 * @returns 00029 * none 00030 */ 00031 WaveDemo(uint8_t *pFrameBuf, uint16_t dispWidth, uint16_t dispHeight); 00032 00033 void run(EaLcdBoardGPIO& lcdBoard, uint32_t loops, uint32_t delayMs); 00034 00035 private: 00036 00037 enum Constants { 00038 N = 1024, // Number of dots 00039 SCALE = 8192, 00040 INCREMENT = 512, // INCREMENT = SCALE / sqrt(N) * 2 00041 SPEED = 5 00042 }; 00043 00044 int32_t windowX; 00045 int32_t windowY; 00046 uint16_t *pFrmBuf; 00047 uint16_t *pFrmBuf1; 00048 uint16_t *pFrmBuf2; 00049 uint16_t *pFrmBuf3; 00050 00051 Graphics graphics; 00052 00053 int16_t sine[SCALE]; 00054 int16_t cosi[SCALE]; 00055 00056 uint16_t fastsqrt(uint32_t n) const; 00057 void matrix(int16_t xyz[3][N], uint8_t rgb[3][N]); 00058 void rotate(int16_t xyz[3][N], uint8_t rgb[3][N], uint16_t angleX, uint16_t angleY, uint16_t angleZ); 00059 void draw(int16_t xyz[3][N], uint8_t rgb[3][N]); 00060 }; 00061 00062 #endif /* WAVEDEMO_H */ 00063
Generated on Tue Jul 12 2022 23:31:58 by
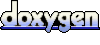