
Graphical demo for the LPC4088 Experiment Base Board with one of the Display Expansion Kits. This program decodes decodes and shows two png images.
TestDisplay.h
00001 /* 00002 * Copyright 2013 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef TESTDISPLAY_H 00018 #define TESTDISPLAY_H 00019 00020 #include "AR1021I2C.h" 00021 #include "Graphics.h" 00022 #include "LcdController.h" 00023 #include "EaLcdBoardGPIO.h" 00024 00025 /** 00026 * Test the display connected with a FPC cable to the LPC4088 Experiment Base Board 00027 * as well as the AR1021 touch sensor on the board. 00028 */ 00029 class TestDisplay { 00030 public: 00031 enum WhichDisplay { 00032 TFT_5, // 5" display 00033 TFT_4_3, // 4.3" display 00034 }; 00035 00036 /** 00037 * Create an interface to the display 00038 */ 00039 TestDisplay(WhichDisplay which); 00040 ~TestDisplay(); 00041 00042 /** 00043 * Test the display 00044 * 00045 * @return true if the test was successful; otherwise false 00046 */ 00047 bool runTest(); 00048 00049 private: 00050 00051 void calibrate_drawMarker(Graphics &g, uint16_t x, uint16_t y, bool erase); 00052 bool calibrate_display(); 00053 00054 char* _initStr; 00055 LcdController::Config* _lcdCfg; 00056 EaLcdBoardGPIO _lcdBoard; 00057 AR1021I2C _touch; 00058 00059 uint32_t _framebuffer; 00060 }; 00061 00062 #endif 00063
Generated on Fri Jul 15 2022 02:02:38 by
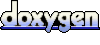