
Graphical demo for the LPC4088 Experiment Base Board with one of the Display Expansion Kits. This program decodes decodes and shows two png images.
PictureDemo.cpp
00001 /****************************************************************************** 00002 * Includes 00003 *****************************************************************************/ 00004 00005 #include "mbed.h" 00006 00007 #include "LcdController.h" 00008 #include "EaLcdBoard.h" 00009 #include "PictureDemo.h" 00010 00011 #include "Image.h" 00012 00013 extern const unsigned char eaLogo_image[]; 00014 extern int eaLogo_image_sz; 00015 00016 extern const unsigned char splash_image[]; 00017 extern int splash_image_sz; 00018 00019 /****************************************************************************** 00020 * Typedefs and defines 00021 *****************************************************************************/ 00022 00023 /****************************************************************************** 00024 * Local variables 00025 *****************************************************************************/ 00026 00027 /****************************************************************************** 00028 * External variables 00029 *****************************************************************************/ 00030 00031 00032 /****************************************************************************** 00033 * Local functions 00034 *****************************************************************************/ 00035 00036 void PictureDemo::drawCentered(const unsigned char* pData, int size, uint32_t delayMs) 00037 { 00038 Image::ImageData_t data; 00039 int result = Image::decode(pData, size, &data); 00040 if (result == 0) 00041 { 00042 // Draw the image centered on the screen 00043 unsigned int xoff = (windowX - data.width)/2; 00044 unsigned int yoff = (windowY - data.height)/2; 00045 for (int y = 0; y < data.height; y++) 00046 { 00047 memcpy(this->pFrmBuf1 + (y+yoff)*windowX + xoff, 00048 data.pixels + y*data.width, 00049 data.width*2); 00050 } 00051 00052 free(data.pixels); 00053 } 00054 else 00055 { 00056 printf("Failed to decode image\n"); 00057 } 00058 } 00059 00060 /****************************************************************************** 00061 * Public functions 00062 *****************************************************************************/ 00063 PictureDemo::PictureDemo(uint8_t *pFrameBuf, uint16_t dispWidth, uint16_t dispHeight) 00064 { 00065 this->windowX = dispWidth; 00066 this->windowY = dispHeight; 00067 this->pFrmBuf = (uint16_t *)pFrameBuf; 00068 this->pFrmBuf1 = (uint16_t *)pFrameBuf; 00069 this->pFrmBuf2 = (uint16_t *)((uint32_t)pFrameBuf + dispWidth*dispHeight*2); 00070 this->pFrmBuf3 = (uint16_t *)((uint32_t)pFrameBuf + dispWidth*dispHeight*4); 00071 } 00072 00073 void PictureDemo::run(EaLcdBoardGPIO& lcdBoard, int image, uint32_t delayMs) 00074 { 00075 printf("PictureDemo, image %d, %dms delay\n", image, delayMs); 00076 00077 memset(this->pFrmBuf1, 0xff, windowX*windowY*2); 00078 switch (image) 00079 { 00080 case 1: 00081 drawCentered(eaLogo_image, eaLogo_image_sz, delayMs); 00082 break; 00083 00084 default: 00085 drawCentered(splash_image, splash_image_sz, delayMs); 00086 break; 00087 } 00088 00089 //update framebuffer 00090 lcdBoard.setFrameBuffer((uint32_t)this->pFrmBuf1); 00091 00092 wait_ms(delayMs); 00093 } 00094
Generated on Fri Jul 15 2022 02:02:38 by
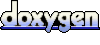