
Graphical demo for the LPC4088 Experiment Base Board with one of the Display Expansion Kits. This program decodes decodes and shows two png images.
Image.h
00001 00002 #ifndef IMAGE_H 00003 #define IMAGE_H 00004 00005 class Image { 00006 public: 00007 00008 enum Type { 00009 BMP = 0, 00010 PNG, 00011 UNKNOWN 00012 }; 00013 00014 typedef struct { 00015 uint16_t* pixels; 00016 uint32_t width; 00017 uint32_t height; 00018 } ImageData_t; 00019 00020 /** Decodes the specified image data 00021 * 00022 * Note that if this function returns a zero, indicating success, 00023 * the pixels member of the pDataOut structure must be 00024 * deallocated using lpc_free() when no longer needed. 00025 * 00026 * @param pDataIn the image data 00027 * @param sizeIn the number of bytes in the pDataIn array 00028 * @param pDataOut the decoded image (only valid if 0 is returned) 00029 * 00030 * @returns 00031 * 0 on success 00032 * 1 on failure 00033 */ 00034 static int decode(const unsigned char* pDataIn, unsigned int sizeIn, ImageData_t* pDataOut); 00035 00036 private: 00037 00038 /** No instance needed 00039 * 00040 */ 00041 Image(); 00042 00043 static Type imageType(const unsigned char* pDataIn, unsigned int sizeIn); 00044 }; 00045 00046 #endif 00047 00048
Generated on Fri Jul 15 2022 02:02:38 by
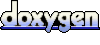