
Graphical demo for the LPC4088 Experiment Base Board with one of the Display Expansion Kits. This program decodes decodes and shows two png images.
Image.cpp
00001 00002 #include "mbed.h" 00003 #include "Image.h" 00004 00005 #include "bmp.h" 00006 #include "lodepng.h" 00007 00008 int Image::decode(const unsigned char* pDataIn, unsigned int sizeIn, ImageData_t* pDataOut) 00009 { 00010 Image::Type type = imageType(pDataIn, sizeIn); 00011 int result = -1; 00012 switch (type) 00013 { 00014 case BMP: 00015 { 00016 struct BMPHeader* hdr = (struct BMPHeader *) pDataIn; 00017 pDataOut->pixels = (uint16_t*)malloc(hdr->width * hdr->height * 2); 00018 if (pDataOut->pixels != NULL) 00019 { 00020 unsigned char error = BMP_Decode((void*)pDataIn, (unsigned char*)pDataOut->pixels, hdr->width, hdr->height, 24); 00021 if (error == 0) 00022 { 00023 pDataOut->width = hdr->width; 00024 pDataOut->height = hdr->height; 00025 return 0; 00026 } 00027 free(pDataOut->pixels); 00028 } 00029 } 00030 break; 00031 00032 case PNG: 00033 { 00034 unsigned char* pTmp; 00035 unsigned error = lodepng_decode24(&pTmp, &pDataOut->width, &pDataOut->height, pDataIn, sizeIn); 00036 if (error == 0) 00037 { 00038 int x, y; 00039 uint16_t* pConverted; 00040 uint8_t r; 00041 uint8_t g; 00042 uint8_t b; 00043 int off = 0; 00044 00045 pDataOut->pixels = (uint16_t*)malloc(pDataOut->width * pDataOut->height * 2); 00046 if (pDataOut->pixels != NULL) 00047 { 00048 pConverted = pDataOut->pixels; 00049 00050 for (y = 0; y < pDataOut->height; y++) { 00051 for (x = 0; x < pDataOut->width; x++) { 00052 r = pTmp[off ]; 00053 g = pTmp[off + 1]; 00054 b = pTmp[off + 2]; 00055 *pConverted = (((unsigned short)r & 0xF8) << 8) | 00056 (((unsigned short)g & 0xFC) << 3) | 00057 (((unsigned short)b & 0xF8) >> 3); 00058 pConverted++; 00059 off += 3; 00060 } 00061 } 00062 } 00063 free(pTmp); 00064 return 0; 00065 } 00066 } 00067 break; 00068 00069 default: 00070 break; 00071 } 00072 00073 pDataOut->pixels = NULL; 00074 pDataOut->width = 0; 00075 pDataOut->height = 0; 00076 return result; 00077 } 00078 00079 Image::Type Image::imageType(const unsigned char* pDataIn, unsigned int sizeIn) 00080 { 00081 if (sizeIn > 4) 00082 { 00083 if (pDataIn[0] == 0x89 && pDataIn[1] == 'P' && pDataIn[2] == 'N' && pDataIn[3] == 'G') 00084 { 00085 return PNG; 00086 } 00087 } 00088 if (BMP_IsValid((void*)pDataIn)) 00089 { 00090 return BMP; 00091 } 00092 return UNKNOWN; 00093 } 00094 00095 00096
Generated on Fri Jul 15 2022 02:02:38 by
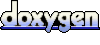