
Graphical demo for the LPC4088 Experiment Base Board with one of the Display Expansion Kits. This program displays how to use the emWin library from Segger.
Dependencies: EALib ewgui mbed
EwGuiImpl.cpp
00001 #include "EwGuiImpl.h" 00002 00003 #include "LcdController.h" 00004 #include "EaLcdBoardGPIO.h" 00005 #include "sdram.h" 00006 #include "AR1021I2C.h" 00007 00008 00009 #define PIXEL_SIZE (2) 00010 00011 #define LCD_CONFIGURATION_43 \ 00012 40, /* horizontalBackPorch */ \ 00013 5, /* horizontalFrontPorch */ \ 00014 2, /* hsync */ \ 00015 480, /* width */ \ 00016 8, /* verticalBackPorch */ \ 00017 8, /* verticalFrontPorch */ \ 00018 2, /* vsync */ \ 00019 272, /* height */ \ 00020 false, /* invertOutputEnable */ \ 00021 false, /* invertPanelClock */ \ 00022 true, /* invertHsync */ \ 00023 true, /* invertVsync */ \ 00024 1, /* acBias */ \ 00025 LcdController::Bpp_16_565, /* bpp */ \ 00026 9000000, /* optimalClock */ \ 00027 LcdController::Tft, /* panelType */ \ 00028 false /* dualPanel */ 00029 00030 #define LCD_INIT_STRING_43 (char*)"v1,cd0,c50,cc0,c30,d100,c31,d100,cd1,d10,o,c51,cc100" 00031 00032 #define LCD_CONFIGURATION_50 \ 00033 46, /* horizontalBackPorch */ \ 00034 20, /* horizontalFrontPorch */ \ 00035 2, /* hsync */ \ 00036 800, /* width */ \ 00037 23, /* verticalBackPorch */ \ 00038 10, /* verticalFrontPorch */ \ 00039 3, /* vsync */ \ 00040 480, /* height */ \ 00041 false, /* invertOutputEnable */ \ 00042 false, /* invertPanelClock */ \ 00043 true, /* invertHsync */ \ 00044 true, /* invertVsync */ \ 00045 1, /* acBias */ \ 00046 LcdController::Bpp_16_565, /* bpp */ \ 00047 30000000, /* optimalClock */ \ 00048 LcdController::Tft, /* panelType */ \ 00049 false /* dualPanel */ 00050 00051 #define LCD_INIT_STRING_50 (char*)"v1,cc0,c31,d50,o,d200,c51,cc100" 00052 00053 EwGuiImpl::EwGuiImpl(WhichDisplay which) { 00054 00055 EaLcdBoardGPIO lcdBoard(P0_27, P0_28); 00056 EaLcdBoardGPIO::Result result; 00057 LcdController::Config* lcdCfg; 00058 00059 do { 00060 00061 switch (which) { 00062 case TFT_5: 00063 lcdCfg = new LcdController::Config(LCD_CONFIGURATION_50); 00064 result = lcdBoard.open(lcdCfg, LCD_INIT_STRING_50); 00065 break; 00066 case TFT_4_3: 00067 lcdCfg = new LcdController::Config(LCD_CONFIGURATION_43); 00068 result = lcdBoard.open(lcdCfg, LCD_INIT_STRING_43); 00069 break; 00070 default: 00071 mbed_die(); 00072 } 00073 00074 if (result != EaLcdBoard::Ok) { 00075 printf("Failed to open display: %d\n", result); 00076 break; 00077 } 00078 00079 _width = lcdCfg->width; 00080 _height = lcdCfg->height; 00081 00082 // allocate buffer, width x height x 2 (2 bytes = 16 bit color data) 00083 _fb = (uint32_t)malloc(_width*_height*PIXEL_SIZE); 00084 if (_fb == 0) { 00085 printf("Failed to allocate frame buffer\n"); 00086 break; 00087 } 00088 00089 result = lcdBoard.setFrameBuffer(_fb); 00090 if (result != EaLcdBoard::Ok) { 00091 printf("Failed to activate frameBuffer: %d\n", result); 00092 break; 00093 } 00094 00095 _memSz = 1024*1024*5; 00096 _mem = (uint32_t)malloc(_memSz); 00097 if (_mem == 0) { 00098 printf("Failed to allocate memory block for emwin\n"); 00099 break; 00100 } 00101 00102 00103 memset((void*)_fb, 0x0, _width*_height*PIXEL_SIZE); 00104 00105 // create touch panel 00106 _touch = new AR1021I2C(P0_27, P0_28, P2_25); 00107 00108 if (!_touch->init(_width, _height)) { 00109 printf("TouchPanel.init failed\n"); 00110 break; 00111 } 00112 00113 init(); 00114 00115 } while(0); 00116 00117 00118 } 00119 00120 void* EwGuiImpl::getMemoryBlockAddress() { 00121 return (void*)_mem; 00122 } 00123 00124 uint32_t EwGuiImpl::getMemoryBlockSize() { 00125 return _memSz; 00126 } 00127 00128 uint32_t EwGuiImpl::getDisplayWidth() { 00129 return _width; 00130 } 00131 00132 uint32_t EwGuiImpl::getDisplayHeight() { 00133 return _height; 00134 } 00135 00136 void* EwGuiImpl::getFrameBufferAddress() { 00137 return (void*)_fb; 00138 } 00139 00140 void EwGuiImpl::getTouchValues(int32_t* x, int32_t* y, int32_t* z) { 00141 00142 if (!x || !y || !z) return; 00143 00144 TouchPanel::touchCoordinate_t coord; 00145 _touch->read(coord); 00146 00147 *x = coord.x; 00148 *y = coord.y; 00149 *z = coord.z; 00150 } 00151 00152 void EwGuiImpl::calibrate() { 00153 00154 uint16_t x = 0; 00155 uint16_t y = 0; 00156 bool hasMorePoints = false; 00157 00158 EwPainter painter; 00159 painter.saveContext(); 00160 00161 do { 00162 00163 printf("Starting calibration\n"); 00164 if (!_touch->calibrateStart()) { 00165 printf("Failed to start calibration\n"); 00166 break; 00167 } 00168 00169 do { 00170 if (!_touch->getNextCalibratePoint(&x, &y)) { 00171 printf("Failed to get next calibrate point\n"); 00172 break; 00173 } 00174 00175 printf("calib: x=%d, y=%d\n", x, y); 00176 drawCalibPoint(painter, x, y); 00177 00178 if (!_touch->waitForCalibratePoint(&hasMorePoints, 0)) { 00179 printf("Failed waiting for calibration point\n"); 00180 break; 00181 } 00182 00183 } while(hasMorePoints); 00184 00185 printf("Calibration done\n"); 00186 00187 00188 } while (0); 00189 00190 00191 painter.clear(); 00192 painter.restoreContext(); 00193 } 00194 00195 void EwGuiImpl::drawCalibPoint(EwPainter &painter, int32_t x, int32_t y) { 00196 00197 painter.clear(); 00198 painter.setColor(EW_WHITE); 00199 painter.drawStringHorizCenter("Touch circle to calibrate", 00200 getDisplayWidth()/2, getDisplayHeight()/2); 00201 00202 painter.fillCircle(x, y, 10); 00203 painter.setColor(EW_BLACK); 00204 painter.fillCircle(x, y, 5); 00205 } 00206
Generated on Thu Jul 14 2022 02:11:06 by
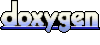