Wrapper classes for the emwin library
Dependents: app_emwin1 app_emwin2_pos lpc4088_ebb_gui_emwin
EwPainter.cpp
00001 #include "mbed.h" 00002 00003 #include "EwPainter.h" 00004 00005 00006 EwPainter::EwPainter() { 00007 _contextSaved = false; 00008 } 00009 00010 void EwPainter::enableAlpha(bool on) { 00011 GUI_EnableAlpha((on ? 1 : 0)); 00012 } 00013 00014 ewColor_t EwPainter::getBackgroundColor() { 00015 return GUI_GetBkColor(); 00016 } 00017 00018 void EwPainter::setBackgroundColor(ewColor_t c) { 00019 GUI_SetBkColor(c); 00020 } 00021 00022 ewColor_t EwPainter::getColor() { 00023 return GUI_GetColor(); 00024 } 00025 00026 void EwPainter::setColor(ewColor_t c) { 00027 GUI_SetColor(c); 00028 } 00029 00030 uint8_t EwPainter::getPenSize() { 00031 return GUI_GetPenSize(); 00032 } 00033 00034 void EwPainter::setPenSize(uint8_t size) { 00035 GUI_SetPenSize(size); 00036 } 00037 00038 void EwPainter::move(int32_t dx, int32_t dy) { 00039 GUI_MoveRel(dx, dy); 00040 } 00041 00042 void EwPainter::moveTo(int32_t x, int32_t y) { 00043 GUI_MoveTo(x, y); 00044 } 00045 00046 void EwPainter::clear() { 00047 GUI_Clear(); 00048 } 00049 00050 void EwPainter::clearRect(int32_t x0, int32_t y0, int32_t x1, int32_t y1) { 00051 GUI_ClearRect(x0, y0, x1, y1); 00052 } 00053 00054 void EwPainter::copyRect(int32_t x0, int32_t y0, int32_t x1, int32_t y1, int32_t width, int32_t height) { 00055 GUI_CopyRect(x0, y0, x1, y1, width, height); 00056 } 00057 00058 void EwPainter::drawHorizGradient(int32_t x0, int32_t y0, int32_t x1, int32_t y1, ewColor_t c0, ewColor_t c1) { 00059 GUI_DrawGradientH(x0, y0, x1, y1, c0, c1); 00060 } 00061 00062 void EwPainter::drawVertGradient(int32_t x0, int32_t y0, int32_t x1, int32_t y1, ewColor_t c0, ewColor_t c1) { 00063 GUI_DrawGradientV(x0, y0, x1, y1, c0, c1); 00064 } 00065 void EwPainter::drawHorizRoundGradient(int32_t x0, int32_t y0, int32_t x1, int32_t y1, int32_t radius, ewColor_t c0, ewColor_t c1) { 00066 GUI_DrawGradientRoundedH(x0, y0, x1, y1, radius, c0, c1); 00067 } 00068 00069 void EwPainter::drawVertRoundGradient(int32_t x0, int32_t y0, int32_t x1, int32_t y1, int32_t radius, ewColor_t c0, ewColor_t c1) { 00070 GUI_DrawGradientRoundedV(x0, y0, x1, y1, radius, c0, c1); 00071 } 00072 00073 void EwPainter::drawPixel(int32_t x, int32_t y) { 00074 GUI_DrawPixel(x, y); 00075 } 00076 00077 void EwPainter::drawPoint(int32_t x, int32_t y) { 00078 GUI_DrawPoint(x, y); 00079 } 00080 00081 void EwPainter::drawRect(int32_t x0, int32_t y0, int32_t x1, int32_t y1) { 00082 GUI_DrawRect(x0, y0, x1, y1); 00083 } 00084 00085 void EwPainter::drawRect(ewRect_t *pRect) { 00086 GUI_DrawRectEx(pRect); 00087 } 00088 00089 00090 void EwPainter::drawRoundedFrame(int32_t x0, int32_t y0, int32_t x1, int32_t y1, int32_t radius, int32_t width) { 00091 GUI_DrawRoundedFrame(x0, y0, x1, y1, radius, width); 00092 } 00093 00094 void EwPainter::drawRoundedRect(int32_t x0, int32_t y0, int32_t x1, int32_t y1, int32_t radius) { 00095 GUI_DrawRoundedRect(x0, y0, x1, y1, radius); 00096 } 00097 00098 void EwPainter::fillRect(int32_t x0, int32_t y0, int32_t x1, int32_t y1) { 00099 GUI_FillRect(x0, y0, x1, y1); 00100 } 00101 00102 void EwPainter::fillRect(ewRect_t *pRect) { 00103 GUI_FillRectEx(pRect); 00104 } 00105 00106 void EwPainter::fillRoundedRect(int32_t x0, int32_t y0, int32_t x1, int32_t y1, int32_t radius) { 00107 GUI_FillRoundedRect(x0, y0, x1, y1, radius); 00108 } 00109 00110 void EwPainter::drawLine(int32_t x0, int32_t y0, int32_t x1, int32_t y1) { 00111 GUI_DrawLine(x0, y0, x1, y1); 00112 } 00113 00114 void EwPainter::drawLine(int32_t dx, int32_t dy) { 00115 GUI_DrawLineRel(dx, dy); 00116 } 00117 00118 void EwPainter::drawLineTo(int32_t x, int32_t y) { 00119 GUI_DrawLineTo(x, y); 00120 } 00121 00122 void EwPainter::drawHorizLine(int32_t y, int32_t x0, int32_t x1) { 00123 GUI_DrawHLine(y, x0, x1); 00124 } 00125 00126 void EwPainter::drawVertLine(int32_t x, int32_t y0, int32_t y1) { 00127 GUI_DrawVLine(x, y0, y1); 00128 } 00129 00130 void EwPainter::drawPolyLine(ewPoint_t* pPoints, int numPoints, int32_t x, int32_t y) { 00131 GUI_DrawPolyLine(pPoints, numPoints, x, y); 00132 } 00133 00134 ewLineStyle_t EwPainter::getLineStyle() { 00135 return (ewLineStyle_t) GUI_GetLineStyle(); 00136 } 00137 00138 ewLineStyle_t EwPainter::setLineStyle(ewLineStyle_t style) { 00139 return (ewLineStyle_t)GUI_SetLineStyle(style); 00140 } 00141 00142 void EwPainter::drawPolygon(ewPoint_t* pPoints, int numPoints, int32_t x, int32_t y) { 00143 GUI_DrawPolygon(pPoints, numPoints, x, y); 00144 } 00145 00146 void EwPainter::enlargePolygon(ewPoint_t* pDest, ewPoint_t* pSrc, int numPoints, int32_t len) { 00147 GUI_EnlargePolygon(pDest, pSrc, numPoints, len); 00148 } 00149 00150 void EwPainter::magnifyPolygon(ewPoint_t* pDest, ewPoint_t* pSrc, int numPoints, int32_t mag) { 00151 GUI_MagnifyPolygon(pDest, pSrc, numPoints, mag); 00152 } 00153 00154 void EwPainter::rotatePolygon(ewPoint_t* pDest, ewPoint_t* pSrc, int numPoints, float angle) { 00155 GUI_RotatePolygon(pDest, pSrc, numPoints, angle); 00156 } 00157 00158 void EwPainter::fillPolygon(ewPoint_t* pPoints, int numPoints, int32_t x, int32_t y) { 00159 GUI_FillPolygon(pPoints, numPoints, x, y); 00160 } 00161 00162 void EwPainter::drawCircle(int32_t x0, int32_t y0, int32_t radius) { 00163 GUI_DrawCircle(x0, y0, radius); 00164 } 00165 00166 void EwPainter::fillCircle(int32_t x0, int32_t y0, int32_t radius) { 00167 GUI_FillCircle(x0, y0, radius); 00168 } 00169 00170 void EwPainter::drawEllipse(int32_t x0, int32_t y0, int32_t rx, int32_t ry) { 00171 GUI_DrawEllipse(x0, y0, rx, ry); 00172 } 00173 00174 void EwPainter::fillEllipse(int32_t x0, int32_t y0, int32_t rx, int32_t ry) { 00175 GUI_FillEllipse(x0, y0, rx, ry); 00176 } 00177 00178 void EwPainter::drawArc(int32_t xCenter, int32_t yCenter, int32_t rx, int32_t ry, int32_t a0, int32_t a1) { 00179 GUI_DrawArc(xCenter, yCenter, rx, ry, a0, a1); 00180 } 00181 00182 void EwPainter::drawGraph(int16_t* paY, int numPoints, int32_t x0, int32_t y0) { 00183 GUI_DrawGraph(paY, numPoints, x0, y0); 00184 } 00185 00186 void EwPainter::drawPie(int32_t x0, int32_t y0, int32_t radius, int32_t a0, int32_t a1) { 00187 GUI_DrawPie(x0, y0, radius, a0, a1, 0); 00188 } 00189 00190 void EwPainter::drawChar(uint16_t c) { 00191 GUI_DispChar(c); 00192 } 00193 00194 void EwPainter::drawChar(uint16_t c, int32_t x, int32_t y) { 00195 GUI_DispCharAt(c, x, y); 00196 } 00197 00198 void EwPainter::drawChars(uint16_t c, int32_t cnt) { 00199 GUI_DispChars(c, cnt); 00200 } 00201 00202 void EwPainter::newLine() { 00203 GUI_DispNextLine(); 00204 } 00205 00206 void EwPainter::drawString(const char* s, int32_t maxLen) { 00207 if (maxLen <= -1) { 00208 GUI_DispString(s); 00209 } 00210 else { 00211 GUI_DispStringLen(s, maxLen); 00212 } 00213 } 00214 void EwPainter::drawString(const char* s, int32_t x, int32_t y) { 00215 GUI_DispStringAt(s, x, y); 00216 } 00217 00218 void EwPainter::drawStringClearEOL(const char* s, int32_t x, int32_t y) { 00219 GUI_DispStringAtCEOL(s, x, y); 00220 } 00221 00222 void EwPainter::drawStringHorizCenter(const char* s, int32_t x, int32_t y) { 00223 GUI_DispStringHCenterAt(s, x, y); 00224 } 00225 00226 void EwPainter::drawStringRect(const char* s, ewRect_t *pRect, ewTextAlign_t align, ewWrapMode_t wrap) { 00227 if (wrap == WrapModeNone) { 00228 GUI_DispStringInRect(s, pRect, align); 00229 } 00230 else { 00231 GUI_DispStringInRectWrap(s, pRect, align, (GUI_WRAPMODE)wrap); 00232 } 00233 } 00234 00235 void EwPainter::drawStringRect(const char* s, ewRect_t *pRect, ewTextAlign_t align, int32_t maxLen, ewRotation_t rotation) { 00236 00237 GUI_ROTATION* ewRotation = GUI_ROTATE_0; 00238 switch (rotation) { 00239 case Rotation_0: 00240 break; 00241 case Rotation_180: 00242 ewRotation = GUI_ROTATE_180; 00243 break; 00244 case Rotation_CCW: 00245 ewRotation = GUI_ROTATE_CCW; 00246 break; 00247 case Rotation_CW: 00248 ewRotation = GUI_ROTATE_CW; 00249 break; 00250 } 00251 00252 GUI_DispStringInRectEx(s, pRect, align, maxLen, ewRotation); 00253 } 00254 00255 int32_t EwPainter::getNumWrapLines(const char* s, int32_t width, ewWrapMode_t wrap) { 00256 return GUI_WrapGetNumLines(s, width, (GUI_WRAPMODE)wrap); 00257 } 00258 00259 ewTextMode_t EwPainter::getTextMode() { 00260 return (ewTextMode_t) GUI_GetTextMode(); 00261 } 00262 00263 void EwPainter::setTextMode(ewTextMode_t mode) { 00264 GUI_SetTextMode(mode); 00265 } 00266 00267 00268 void EwPainter::setTextStyle(ewTextStyle_t style) { 00269 GUI_SetTextStyle(style); 00270 } 00271 00272 ewTextAlign_t EwPainter::getTextAlign() { 00273 return (ewTextAlign_t) GUI_GetTextAlign(); 00274 } 00275 00276 void EwPainter::setTextAlign(ewTextAlign_t align) { 00277 GUI_SetTextAlign(align); 00278 } 00279 00280 bool EwPainter::setTextPosition(int32_t x, int32_t y) { 00281 return (GUI_GotoXY(x, y) == 0); 00282 } 00283 00284 void EwPainter::getTextPosition(int32_t &x, int32_t &y) { 00285 x = GUI_GetDispPosX(); 00286 y = GUI_GetDispPosY(); 00287 } 00288 00289 const ewFont_t* EwPainter::setFont(const ewFont_t* pFont) { 00290 return GUI_SetFont(pFont); 00291 } 00292 00293 const ewFont_t* EwPainter::getFont() { 00294 return GUI_GetFont(); 00295 } 00296 00297 int32_t EwPainter::getCharWidth(uint16_t c) { 00298 return GUI_GetCharDistX(c); 00299 } 00300 00301 int32_t EwPainter::getFontHeight() { 00302 return GUI_GetFontSizeY(); 00303 } 00304 00305 int32_t EwPainter::getFontDistY() { 00306 return GUI_GetFontDistY(); 00307 } 00308 00309 int32_t EwPainter::getLeadingBlankCols(uint16_t c) { 00310 return GUI_GetLeadingBlankCols(c); 00311 } 00312 00313 int32_t EwPainter::getTrailingBlankCols(uint16_t c) { 00314 return GUI_GetTrailingBlankCols(c); 00315 } 00316 00317 int32_t EwPainter::getStringWidth(const char* s) { 00318 return GUI_GetStringDistX(s); 00319 } 00320 00321 bool EwPainter::isCharInFont(const ewFont_t* pFont, uint16_t c) { 00322 return (GUI_IsInFont(pFont, c) == 1); 00323 } 00324 00325 void EwPainter::drawBitmap(const ewBitmap_t* pBm, int32_t x, int32_t y) { 00326 GUI_DrawBitmap(pBm, x, y); 00327 } 00328 00329 void EwPainter::drawBitmapMagnified(const ewBitmap_t* pBm, int32_t x, int32_t y, int32_t xMul, int32_t yMul) { 00330 GUI_DrawBitmapMag(pBm, x, y, xMul, yMul); 00331 } 00332 00333 void EwPainter::drawBitmapScaled(const ewBitmap_t* pBm, int32_t x, int32_t y, int32_t xCenter, int32_t yCenter, int32_t xMag, int32_t yMag) { 00334 GUI_DrawBitmapEx(pBm, x, y, xCenter, yCenter, xMag, yMag); 00335 } 00336 00337 void EwPainter::drawJpeg(const void* pData, int32_t dataSize, int32_t x, int32_t y) { 00338 GUI_JPEG_Draw(pData, dataSize, x, y); 00339 } 00340 00341 void EwPainter::drawJpegScaled(const void* pData, int32_t dataSize, int32_t x, int32_t y, int32_t num, int32_t denom) { 00342 GUI_JPEG_DrawScaled(pData, dataSize, x, y, num, denom); 00343 } 00344 00345 void EwPainter::getJpegSize(const void* pData, int32_t dataSize, int32_t &width, int32_t &height) { 00346 GUI_JPEG_INFO info; 00347 GUI_JPEG_GetInfo(pData, dataSize, &info); 00348 00349 width = info.XSize; 00350 height = info.YSize; 00351 } 00352 00353 void EwPainter::drawGif(const void* pData, int32_t dataSize, int32_t x, int32_t y, int32_t imgIdx) { 00354 if (imgIdx < 0) { 00355 GUI_GIF_Draw(pData, dataSize, x, y); 00356 } 00357 else { 00358 GUI_GIF_DrawSub(pData, dataSize, x, y, imgIdx); 00359 } 00360 } 00361 00362 void EwPainter::drawGifScaled(const void* pData, int32_t dataSize, int32_t x, int32_t y, int32_t imgIdx, int32_t num, int32_t denom) { 00363 GUI_GIF_DrawSubScaled(pData, dataSize, x, y, imgIdx, num, denom); 00364 } 00365 00366 void EwPainter::getGifSize(const void* pData, int32_t dataSize, int32_t &width, int32_t &height) { 00367 width = GUI_GIF_GetXSize(pData); 00368 height = GUI_GIF_GetYSize(pData); 00369 } 00370 00371 int32_t EwPainter::getGifNumImages(const void* pData, int32_t dataSize) { 00372 GUI_GIF_INFO info; 00373 GUI_GIF_GetInfo(pData, dataSize, &info); 00374 00375 return info.NumImages; 00376 } 00377 00378 void EwPainter::drawPng(const void* pData, int32_t dataSize, int32_t x, int32_t y) { 00379 GUI_PNG_Draw(pData, dataSize, x, y); 00380 } 00381 00382 void EwPainter::getPngSize(const void* pData, int32_t dataSize, int32_t &width, int32_t &height) { 00383 width = GUI_PNG_GetXSize(pData, dataSize); 00384 height = GUI_PNG_GetYSize(pData, dataSize);; 00385 } 00386 00387 00388 void EwPainter::saveContext() { 00389 GUI_SaveContext_W(&_context); 00390 _contextSaved = true; 00391 } 00392 00393 void EwPainter::restoreContext() { 00394 if (!_contextSaved) return; 00395 00396 GUI_RestoreContext(&_context); 00397 00398 _contextSaved = false; 00399 } 00400 00401
Generated on Tue Jul 12 2022 19:57:49 by
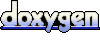