Wrapper classes for the emwin library
Dependents: app_emwin1 app_emwin2_pos lpc4088_ebb_gui_emwin
EwFunctionPointer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef EWFUNCTIONPOINTER_H 00017 #define EWFUNCTIONPOINTER_H 00018 00019 #include <string.h> 00020 #include <stdint.h> 00021 #include "EwWindow.h" 00022 00023 00024 00025 00026 /** A class for storing and calling a pointer to a static or member void function 00027 */ 00028 class EwFunctionPointer { 00029 public: 00030 00031 /** Create a FunctionPointer, attaching a static function 00032 * 00033 * @param function The void static function to attach (default is none) 00034 */ 00035 EwFunctionPointer(void (*function)(EwWindow* w) = 0); 00036 00037 /** Create a FunctionPointer, attaching a member function 00038 * 00039 * @param object The object pointer to invoke the member function on (i.e. the this pointer) 00040 * @param function The address of the void member function to attach 00041 */ 00042 template<typename T> 00043 EwFunctionPointer(T *object, void (T::*member)(EwWindow* w)) { 00044 attach(object, member); 00045 } 00046 00047 /** Attach a static function 00048 * 00049 * @param function The void static function to attach (default is none) 00050 */ 00051 void attach(void (*function)(EwWindow* w) = 0); 00052 00053 /** Attach a member function 00054 * 00055 * @param object The object pointer to invoke the member function on (i.e. the this pointer) 00056 * @param function The address of the void member function to attach 00057 */ 00058 template<typename T> 00059 void attach(T *object, void (T::*member)(EwWindow* w)) { 00060 _object = static_cast<void*>(object); 00061 memcpy(_member, (char*)&member, sizeof(member)); 00062 _membercaller = &EwFunctionPointer::membercaller<T>; 00063 _function = 0; 00064 } 00065 00066 /** Call the attached static or member function 00067 */ 00068 void call(EwWindow* w); 00069 00070 00071 00072 00073 private: 00074 template<typename T> 00075 static void membercaller(void *object, char *member, EwWindow* w) { 00076 T* o = static_cast<T*>(object); 00077 void (T::*m)(EwWindow* w); 00078 memcpy((char*)&m, member, sizeof(m)); 00079 (o->*m)(w); 00080 } 00081 00082 void (*_function)(EwWindow* w); // static function pointer - 0 if none attached 00083 void *_object; // object this pointer - 0 if none attached 00084 char _member[16]; // raw member function pointer storage - converted back by registered _membercaller 00085 void (*_membercaller)(void*, char*, EwWindow* w); // registered membercaller function to convert back and call _member on _object 00086 }; 00087 00088 00089 00090 #endif
Generated on Tue Jul 12 2022 19:57:49 by
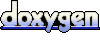