Wrapper classes for the emwin library
Dependents: app_emwin1 app_emwin2_pos lpc4088_ebb_gui_emwin
EwFrameWindow.cpp
00001 #include "mbed.h" 00002 00003 #include "EwFrameWindow.h" 00004 00005 00006 EwFrameWindow::EwFrameWindow(const char* pTitle, EwWindow* parent) : EwWindow(parent) { 00007 init(0, 0, 0, 0, pTitle, parent); 00008 } 00009 00010 EwFrameWindow::EwFrameWindow(int x, int y, int width, int height, const char* pTitle, EwWindow* parent) : 00011 EwWindow(x, y, width, height, parent) { 00012 00013 init(x, y, width, height, pTitle, parent); 00014 } 00015 00016 00017 void EwFrameWindow::addCloseButton(ewFrameWindowButtonAlign_t align, int32_t offset) { 00018 FRAMEWIN_AddCloseButton(_hWnd, align, offset); 00019 } 00020 00021 void EwFrameWindow::addMaxButton(ewFrameWindowButtonAlign_t align, int32_t offset) { 00022 FRAMEWIN_AddMaxButton(_hWnd, align, offset); 00023 } 00024 00025 void EwFrameWindow::addMinButton(ewFrameWindowButtonAlign_t align, int32_t offset) { 00026 FRAMEWIN_AddMinButton(_hWnd, align, offset); 00027 } 00028 00029 bool EwFrameWindow::isActive() { 00030 return (FRAMEWIN_GetActive(_hWnd) == 1); 00031 } 00032 00033 ewColor_t EwFrameWindow::getBarColor(ewFrameWindowColorIndex_t idx) { 00034 return FRAMEWIN_GetBarColor(_hWnd, idx); 00035 } 00036 00037 int32_t EwFrameWindow::getBorderSize() { 00038 return FRAMEWIN_GetBorderSize(_hWnd); 00039 } 00040 00041 const ewFont_t* EwFrameWindow::getFont() { 00042 return FRAMEWIN_GetFont(_hWnd); 00043 } 00044 00045 void EwFrameWindow::getText(char* pBuffer, int32_t maxLen) { 00046 FRAMEWIN_GetText(_hWnd, pBuffer, maxLen); 00047 } 00048 00049 ewTextAlign_t EwFrameWindow::getTextAlign() { 00050 return (ewTextAlign_t) FRAMEWIN_GetTextAlign(_hWnd); 00051 } 00052 00053 int32_t EwFrameWindow::getTitleHeight() { 00054 return FRAMEWIN_GetTitleHeight(_hWnd); 00055 } 00056 00057 bool EwFrameWindow::isMaximized() { 00058 return (FRAMEWIN_IsMaximized(_hWnd) == 1); 00059 } 00060 00061 bool EwFrameWindow::isMinimized() { 00062 return (FRAMEWIN_IsMinimized(_hWnd) == 1); 00063 } 00064 00065 void EwFrameWindow::maximize() { 00066 FRAMEWIN_Maximize(_hWnd); 00067 } 00068 00069 void EwFrameWindow::minimize() { 00070 FRAMEWIN_Minimize(_hWnd); 00071 } 00072 00073 void EwFrameWindow::restore() { 00074 FRAMEWIN_Restore(_hWnd); 00075 } 00076 00077 void EwFrameWindow::setActive(bool active) { 00078 FRAMEWIN_SetActive(_hWnd, (active ? 1 : 0)); 00079 } 00080 00081 void EwFrameWindow::setBarColor(ewFrameWindowColorIndex_t idx, ewColor_t c) { 00082 FRAMEWIN_SetBarColor(_hWnd, idx, c); 00083 } 00084 00085 void EwFrameWindow::setBorderSize(uint32_t size) { 00086 FRAMEWIN_SetBorderSize(_hWnd, size); 00087 } 00088 00089 void EwFrameWindow::setClientColor(ewColor_t c) { 00090 FRAMEWIN_SetClientColor(_hWnd, c); 00091 } 00092 00093 void EwFrameWindow::setFont(const ewFont_t* pFont) { 00094 FRAMEWIN_SetFont(_hWnd, pFont); 00095 } 00096 00097 void EwFrameWindow::setMoveable(bool moveable) { 00098 FRAMEWIN_SetMoveable(_hWnd, (moveable ? 1 : 0)); 00099 } 00100 00101 void EwFrameWindow::setResizeable(bool resizeable) { 00102 FRAMEWIN_SetResizeable(_hWnd, (resizeable ? 1 : 0)); 00103 } 00104 00105 void EwFrameWindow::setText(const char* s) { 00106 FRAMEWIN_SetText(_hWnd, s); 00107 } 00108 00109 void EwFrameWindow::setTextAlign(ewTextAlign_t align) { 00110 FRAMEWIN_SetTextAlign(_hWnd, align); 00111 } 00112 00113 void EwFrameWindow::setTextColor(ewFrameWindowColorIndex_t idx, ewColor_t c) { 00114 FRAMEWIN_SetTextColorEx(_hWnd, idx, c); 00115 } 00116 00117 void EwFrameWindow::setTitleHeight(int32_t height) { 00118 FRAMEWIN_SetTitleHeight(_hWnd, height); 00119 } 00120 00121 void EwFrameWindow::setTitleVisible(bool visible) { 00122 FRAMEWIN_SetTitleVis(_hWnd, (visible ? 1 : 0)); 00123 } 00124 00125 00126 ewColor_t EwFrameWindow::getDefaultBarColor(ewFrameWindowColorIndex_t idx) { 00127 return FRAMEWIN_GetDefaultBarColor(idx); 00128 } 00129 00130 int32_t EwFrameWindow::getDefaultBorderSize() { 00131 return FRAMEWIN_GetDefaultBorderSize(); 00132 } 00133 00134 ewColor_t EwFrameWindow::getDefaultClientColor() { 00135 return FRAMEWIN_GetDefaultClientColor(); 00136 } 00137 00138 const ewFont_t* EwFrameWindow::getDefaultFont() { 00139 return FRAMEWIN_GetDefaultFont(); 00140 } 00141 00142 ewColor_t EwFrameWindow::getDefaultTextColor(ewFrameWindowColorIndex_t idx) { 00143 return FRAMEWIN_GetDefaultTextColor(idx); 00144 } 00145 00146 int32_t EwFrameWindow::getDefaultTitleHeight() { 00147 return FRAMEWIN_GetDefaultTitleHeight(); 00148 } 00149 00150 void EwFrameWindow::setDefaultBarColor(ewFrameWindowColorIndex_t idx, ewColor_t c) { 00151 FRAMEWIN_SetDefaultBarColor(idx, c); 00152 } 00153 00154 void EwFrameWindow::setDefaultBorderSize(int32_t size) { 00155 FRAMEWIN_SetDefaultBorderSize(size); 00156 } 00157 00158 void EwFrameWindow::setDefaultClientColor(ewColor_t c) { 00159 FRAMEWIN_SetDefaultClientColor(c); 00160 } 00161 00162 void EwFrameWindow::setDefaultTextColor(ewFrameWindowColorIndex_t idx, ewColor_t c) { 00163 FRAMEWIN_SetDefaultTextColor(idx, c); 00164 } 00165 00166 void EwFrameWindow::setDefaultTitleHeight(int32_t height) { 00167 FRAMEWIN_SetDefaultTitleHeight(height); 00168 } 00169 00170 00171 00172 void EwFrameWindow::init(int x, int y, int width, int height, const char* pTitle, EwWindow* parent) { 00173 FRAMEWIN_Handle h; 00174 GUI_HWIN hWin = 0; 00175 00176 if (parent) { 00177 hWin = _getHandle(parent); 00178 } 00179 00180 h = FRAMEWIN_CreateEx(x, y, width, height, hWin, (WM_CF_SHOW| WM_CF_MEMDEV), 00181 FRAMEWIN_CF_MOVEABLE, _guiWidgetId++, pTitle, NULL); 00182 _setNewHandle(h, NULL); 00183 } 00184
Generated on Tue Jul 12 2022 19:57:49 by
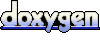