Wrapper classes for the emwin library
Dependents: app_emwin1 app_emwin2_pos lpc4088_ebb_gui_emwin
EwEdit.cpp
00001 #include "mbed.h" 00002 00003 #include "EwEdit.h" 00004 00005 00006 EwEdit::EwEdit(int maxLen, EwWindow* parent) : EwWindow(parent) { 00007 init(0, 0, 0, 0, maxLen, parent); 00008 } 00009 00010 EwEdit::EwEdit(int x, int y, int width, int height, int maxLen, EwWindow* parent) : 00011 EwWindow(x, y, width, height, parent) { 00012 00013 init(x, y, width, height, maxLen, parent); 00014 } 00015 00016 void EwEdit::addKey(int32_t key) { 00017 EDIT_AddKey(_hWnd, key); 00018 } 00019 00020 void EwEdit::enableBlink(int32_t period, bool on) { 00021 EDIT_EnableBlink(_hWnd, period, (on ? 1 : 0)); 00022 } 00023 00024 ewColor_t EwEdit::getBackgroundColor(ewEditColorIndex_t idx) { 00025 return EDIT_GetBkColor(_hWnd, idx); 00026 } 00027 00028 int32_t EwEdit::getCursorCharPosition() { 00029 return EDIT_GetCursorCharPos(_hWnd); 00030 } 00031 00032 void EwEdit::getCursorPixelPosition(int32_t* x, int32_t* y) { 00033 EDIT_GetCursorPixelPos(_hWnd, (int*)x, (int*)y); 00034 } 00035 00036 float EwEdit::getFloatValue() { 00037 return EDIT_GetFloatValue(_hWnd); 00038 } 00039 00040 const ewFont_t* EwEdit::getFont() { 00041 return EDIT_GetFont(_hWnd); 00042 } 00043 00044 int32_t EwEdit::getNumChars() { 00045 return EDIT_GetNumChars(_hWnd); 00046 } 00047 00048 void EwEdit::getText(char* pBuffer, int32_t maxLen) { 00049 EDIT_GetText(_hWnd, pBuffer, maxLen); 00050 } 00051 00052 ewColor_t EwEdit::getTextColor(ewEditColorIndex_t idx) { 00053 return EDIT_GetTextColor(_hWnd, idx); 00054 } 00055 00056 int32_t EwEdit::getValue() { 00057 return EDIT_GetValue(_hWnd); 00058 } 00059 00060 void EwEdit::setBinMode(uint32_t value, uint32_t min, uint32_t max) { 00061 EDIT_SetBinMode(_hWnd, value, min, max); 00062 } 00063 00064 void EwEdit::setBackgroundColor(ewEditColorIndex_t idx, ewColor_t c) { 00065 EDIT_SetBkColor(_hWnd, idx, c); 00066 } 00067 00068 void EwEdit::setCursorAtChar(int32_t charPos) { 00069 EDIT_SetCursorAtChar(_hWnd, charPos); 00070 } 00071 00072 void EwEdit::setCursorAtPixel(int32_t pos) { 00073 EDIT_SetCursorAtPixel(_hWnd, pos); 00074 } 00075 00076 void EwEdit::setDecimalMode(int32_t value, int32_t min, int32_t max, int32_t shift, ewEditMode_t mode) { 00077 EDIT_SetDecMode(_hWnd, value, min, max, shift, mode); 00078 } 00079 00080 void EwEdit::setFloatMode(float value, float min, float max, int32_t shift, ewEditMode_t mode) { 00081 EDIT_SetFloatMode(_hWnd, value, min, max, shift, mode); 00082 } 00083 00084 void EwEdit::setFloatValue(float value) { 00085 EDIT_SetFloatValue(_hWnd, value); 00086 } 00087 00088 void EwEdit::setFocussable(bool focussable) { 00089 EDIT_SetFocussable(_hWnd, (focussable ? 1 : 0)); 00090 } 00091 00092 void EwEdit::setFont(const ewFont_t* pFont) { 00093 EDIT_SetFont(_hWnd, pFont); 00094 } 00095 00096 void EwEdit::setHexMode(uint32_t value, uint32_t min, uint32_t max) { 00097 EDIT_SetHexMode(_hWnd, value, min, max); 00098 } 00099 00100 void EwEdit::setInsertMode(bool on) { 00101 EDIT_SetInsertMode(_hWnd, (on ? 1 : 0)); 00102 } 00103 00104 void EwEdit::setMaximumLength(int32_t maxLen) { 00105 EDIT_SetMaxLen(_hWnd, maxLen); 00106 } 00107 00108 void EwEdit::setSelection(int32_t firstChar, int32_t lastChar) { 00109 EDIT_SetSel(_hWnd, firstChar, lastChar); 00110 } 00111 00112 void EwEdit::setText(const char* s) { 00113 EDIT_SetText(_hWnd, s); 00114 } 00115 00116 void EwEdit::setTextAlign(ewTextAlign_t align) { 00117 EDIT_SetTextAlign(_hWnd, align); 00118 } 00119 00120 void EwEdit::setTextColor(ewEditColorIndex_t idx, ewColor_t c) { 00121 EDIT_SetTextColor(_hWnd, idx, c); 00122 } 00123 00124 void EwEdit::setTextMode() { 00125 EDIT_SetTextMode(_hWnd); 00126 } 00127 00128 void EwEdit::setULongMode(uint32_t value, uint32_t min, uint32_t max) { 00129 EDIT_SetUlongMode(_hWnd, value, min, max); 00130 } 00131 00132 void EwEdit::setValue(int32_t value) { 00133 EDIT_SetValue(_hWnd, value); 00134 } 00135 00136 00137 ewColor_t EwEdit::getDefaultBackgroundColor(ewEditColorIndex_t idx) { 00138 return EDIT_GetDefaultBkColor(idx); 00139 } 00140 00141 const ewFont_t* EwEdit::getDefaultFont() { 00142 return EDIT_GetDefaultFont(); 00143 } 00144 00145 ewTextAlign_t EwEdit::getDefaultTextAlign() { 00146 return (ewTextAlign_t)EDIT_GetDefaultTextAlign(); 00147 } 00148 00149 ewColor_t EwEdit::getDefaultTextColor(ewEditColorIndex_t idx) { 00150 return EDIT_GetDefaultTextColor(idx); 00151 } 00152 00153 void EwEdit::setDefaultBackgroundColor(ewEditColorIndex_t idx, ewColor_t c) { 00154 EDIT_SetDefaultBkColor(idx, c); 00155 } 00156 00157 void EwEdit::setDefaultFont(const ewFont_t* pFont) { 00158 EDIT_SetDefaultFont(pFont); 00159 } 00160 00161 void EwEdit::setDefaultTextAlign(ewTextAlign_t align) { 00162 EDIT_SetDefaultTextAlign(align); 00163 } 00164 00165 void EwEdit::setDefaultTextColor(ewEditColorIndex_t idx, ewColor_t c) { 00166 EDIT_SetDefaultTextColor(idx, c); 00167 } 00168 00169 uint32_t EwEdit::editBin(uint32_t value, uint32_t min, uint32_t max, int32_t len, int32_t width) { 00170 return GUI_EditBin(value, min, max, len, width); 00171 } 00172 00173 uint32_t EwEdit::editDec(int32_t value, int32_t min, int32_t max, int32_t len, int32_t width, int32_t shift, ewEditMode_t mode) { 00174 return GUI_EditDec(value, min, max, len, width, shift, mode); 00175 } 00176 00177 float EwEdit::editFloat(float value, float min, float max, int32_t len, int32_t width, int32_t shift, ewEditMode_t mode) { 00178 return GUI_EditFloat(value, min, max, len, width, shift, mode); 00179 } 00180 00181 uint32_t EwEdit::editHex(uint32_t value, uint32_t min, uint32_t max, int32_t len, int32_t width) { 00182 return GUI_EditHex(value, min, max, len, width); 00183 } 00184 00185 void EwEdit::editString(char* pString, int32_t len, int32_t width) { 00186 GUI_EditString(pString, len, width); 00187 } 00188 00189 00190 void EwEdit::init(int x, int y, int width, int height, int maxLen, EwWindow* parent) { 00191 EDIT_Handle h; 00192 GUI_HWIN hWin = 0; 00193 00194 00195 if (parent) { 00196 hWin = _getHandle(parent); 00197 } 00198 00199 h = EDIT_CreateEx(x, y, width, height, hWin, (WM_CF_SHOW| WM_CF_MEMDEV), 00200 0, _guiWidgetId++, maxLen); 00201 _setNewHandle(h, _callback); 00202 } 00203 00204 00205 void EwEdit::_callback(WM_MESSAGE* pMsg, EwWindow* w) { 00206 EDIT_Callback(pMsg); 00207 } 00208
Generated on Tue Jul 12 2022 19:57:49 by
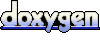