Wrapper classes for the emwin library
Dependents: app_emwin1 app_emwin2_pos lpc4088_ebb_gui_emwin
EwDropDown.h
00001 00002 #ifndef EWDROPDOWN_H 00003 #define EWDROPDOWN_H 00004 00005 #include "EwPainter.h" 00006 #include "EwWindow.h" 00007 #include "EwFunctionPointer.h" 00008 00009 #include "DROPDOWN.h" 00010 00011 // TODO: move to EwScrollbar.h -----------------> 00012 #include "SCROLLBAR.h" 00013 00014 enum ewScrollBarColorIndex_t { 00015 ScrollBarColorIndexThumb = SCROLLBAR_CI_THUMB, 00016 ScrollBarColorIndexShaft = SCROLLBAR_CI_SHAFT, 00017 ScrollBarColorIndexArrow = SCROLLBAR_CI_ARROW, 00018 }; 00019 // <--------------------------------------------- 00020 00021 enum ewDropDownColorIndex_t { 00022 DropDownColorIndexUnselected = DROPDOWN_CI_UNSEL, 00023 DropDownColorIndexSelected = DROPDOWN_CI_SEL, 00024 DropDownColorIndexSelFocus = DROPDOWN_CI_SELFOCUS, 00025 }; 00026 00027 /** 00028 * This is a wrapper class for the emwin DROPDOWN interface. 00029 */ 00030 class EwDropDown : public EwWindow { 00031 public: 00032 00033 EwDropDown(EwWindow* parent = 0); 00034 EwDropDown(int x, int y, int width, int height, EwWindow* parent = 0); 00035 00036 00037 void addString(const char* s); 00038 void collapse(); 00039 void decrementSelection(); 00040 void deleteItem(uint32_t idx); 00041 void expand(); 00042 bool isItemDisabled(uint32_t idx); 00043 bool getItemText(uint32_t idx, char* pBuffer, int maxSize); 00044 int getNumItems(); 00045 int32_t getSelectedIndex(); 00046 void incrementSelection(); 00047 void insertString(const char* s, uint32_t idx); 00048 void setAutoScroll(bool on); 00049 void setBackgroundColor(ewDropDownColorIndex_t idx, ewColor_t c); 00050 void setColor(ewDropDownColorIndex_t idx, ewColor_t c); 00051 void setFont(const ewFont_t* pFont); 00052 void setItemEnableState(uint32_t idx, bool enabled); 00053 void setScrollbarColor(ewScrollBarColorIndex_t idx, ewColor_t c); 00054 void setScrollbarWidth(uint32_t width); 00055 void setSelectedItem(int32_t idx); 00056 void setItemSpacing(uint32_t spacing); 00057 void setTextAlign(ewTextAlign_t align); 00058 void setTextColor(ewDropDownColorIndex_t idx, ewColor_t c); 00059 void setTextHeight(uint32_t height); 00060 void setUpMode(bool upMode); 00061 00062 /** 00063 * Register a member function that will called when a selection 00064 * in the drop-down list has changed. 00065 * 00066 * @param tptr pointer to the object to call the member function on 00067 * @param mptr pointer to the member function to be called 00068 */ 00069 template<typename T> 00070 void setSelectionChangedListener(T* tptr, void (T::*mptr)(EwWindow* w)) { 00071 if((mptr != NULL) && (tptr != NULL)) { 00072 _selChangedListener.attach(tptr, mptr); 00073 } 00074 } 00075 00076 /** 00077 * Register a function that will called when the a selection 00078 * in the drop-down list has changed. 00079 * 00080 * @param fptr A pointer to a void function that will be called 00081 */ 00082 void setSelectionChangedListener(void (*fptr)(EwWindow* w)); 00083 00084 static ewColor_t getDefaultBackgroundColor(ewDropDownColorIndex_t idx); 00085 static ewColor_t getDefaultColor(ewDropDownColorIndex_t idx); 00086 static ewColor_t getDefaultScrollbarColor(ewDropDownColorIndex_t idx); 00087 static const ewFont_t* getDefaultFont(); 00088 00089 void setDefaultBackgroundColor(ewDropDownColorIndex_t idx, ewColor_t c); 00090 static void setDefaultColor(ewDropDownColorIndex_t idx, ewColor_t c); 00091 static void setDefaultFont(const ewFont_t* pFont); 00092 static void setDefaultScrollbarColor(ewScrollBarColorIndex_t idx, ewColor_t c); 00093 00094 private: 00095 00096 EwFunctionPointer _selChangedListener; 00097 00098 void init(int x, int y, int width, int height, EwWindow* parent); 00099 void handleSelectionChanged(); 00100 00101 static void _callback(WM_MESSAGE* pMsg, EwWindow* w); 00102 00103 00104 }; 00105 00106 #endif
Generated on Tue Jul 12 2022 19:57:49 by
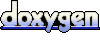