Wrapper classes for the emwin library
Dependents: app_emwin1 app_emwin2_pos lpc4088_ebb_gui_emwin
EwButton.cpp
00001 #include "mbed.h" 00002 00003 #include "EwButton.h" 00004 00005 00006 EwButton::EwButton(EwWindow* parent) : EwWindow(parent) { 00007 init(0, 0, 0, 0, parent); 00008 } 00009 00010 EwButton::EwButton(int x, int y, int width, int height, EwWindow* parent) : 00011 EwWindow(x, y, width, height, parent) { 00012 00013 init(x, y, width, height, parent); 00014 } 00015 00016 void EwButton::setText(const char* text) { 00017 BUTTON_SetText(_hWnd, text); 00018 } 00019 00020 void EwButton::getText(char* pBuf, int bufSz) { 00021 BUTTON_GetText(_hWnd, pBuf, bufSz); 00022 } 00023 00024 ewColor_t EwButton::getBackgroundColor(ewButtonColorIndex_t idx) { 00025 return BUTTON_GetBkColor(_hWnd, idx); 00026 } 00027 00028 void EwButton::setBackgroundColor(ewButtonColorIndex_t idx, ewColor_t c) { 00029 BUTTON_SetBkColor(_hWnd, idx, c); 00030 } 00031 00032 const ewFont_t* EwButton::getFont() { 00033 return BUTTON_GetFont(_hWnd); 00034 } 00035 00036 void EwButton::setFont(const ewFont_t* pFont) { 00037 BUTTON_SetFont(_hWnd, pFont); 00038 } 00039 00040 ewTextAlign_t EwButton::getTextAlign() { 00041 return (ewTextAlign_t)BUTTON_GetTextAlign(_hWnd); 00042 } 00043 00044 void EwButton::setTextAlign(ewTextAlign_t align) { 00045 BUTTON_SetTextAlign(_hWnd, align); 00046 } 00047 00048 ewColor_t EwButton::getTextColor(ewButtonColorIndex_t idx) { 00049 return BUTTON_GetTextColor(_hWnd, idx); 00050 } 00051 00052 void EwButton::setTextColor(ewButtonColorIndex_t idx, ewColor_t c) { 00053 BUTTON_SetTextColor(_hWnd, idx, c); 00054 } 00055 00056 void EwButton::setFocusColor(ewColor_t c) { 00057 BUTTON_SetFocusColor(_hWnd, c); 00058 } 00059 00060 void EwButton::setFocussable(bool focussable) { 00061 BUTTON_SetFocussable(_hWnd, (focussable ? 1 : 0)); 00062 } 00063 00064 void EwButton::setPressed(bool pressed) { 00065 BUTTON_SetPressed(_hWnd, (pressed ? 1 : 0)); 00066 } 00067 00068 bool EwButton::isPressed() { 00069 return (BUTTON_IsPressed(_hWnd) == 1); 00070 } 00071 00072 00073 void EwButton::setTextOffset(int32_t xPos, int32_t yPos) { 00074 BUTTON_SetTextOffset(_hWnd, xPos, yPos); 00075 } 00076 00077 const ewBitmap_t* EwButton::getBitmap(ewButtonColorIndex_t idx) { 00078 return BUTTON_GetBitmap(_hWnd, idx); 00079 } 00080 00081 void EwButton::setBitmap(ewButtonColorIndex_t idx, const ewBitmap_t* pBitmap) { 00082 BUTTON_SetBitmap(_hWnd, idx, pBitmap); 00083 } 00084 00085 void EwButton::setBitmap(ewButtonColorIndex_t idx, const ewBitmap_t* pBitmap, int32_t x, int32_t y) { 00086 BUTTON_SetBitmapEx(_hWnd, idx, pBitmap, x, y); 00087 } 00088 00089 void EwButton::setPressedListener(void (*fptr)(EwWindow* w)) { 00090 _pressedListener.attach(fptr); 00091 } 00092 00093 void EwButton::setReleasedListener(void (*fptr)(EwWindow* w)) { 00094 _releasedListener.attach(fptr); 00095 } 00096 00097 void EwButton::setClickedListener(void (*fptr)(EwWindow* w)) { 00098 _clickedListener.attach(fptr); 00099 } 00100 00101 ewColor_t EwButton::getDefaultBackgroundColor(ewButtonColorIndex_t idx) { 00102 return BUTTON_GetDefaultBkColor(idx); 00103 } 00104 00105 void EwButton::setDefaultBackgroundColor(ewButtonColorIndex_t idx, ewColor_t c) { 00106 BUTTON_SetDefaultBkColor(c, idx); 00107 } 00108 00109 void EwButton::setDefaultFont(const ewFont_t* pFont) { 00110 BUTTON_SetDefaultFont(pFont); 00111 } 00112 00113 const ewFont_t* EwButton::getDefaultFont() { 00114 return BUTTON_GetDefaultFont(); 00115 } 00116 00117 ewTextAlign_t EwButton::getDefaultTextAlign() { 00118 return (ewTextAlign_t) BUTTON_GetDefaultTextAlign(); 00119 } 00120 00121 void EwButton::setDefaultTextAlign(ewTextAlign_t align) { 00122 BUTTON_SetDefaultTextAlign(align); 00123 } 00124 00125 ewColor_t EwButton::getDefaultTextColor(ewButtonColorIndex_t idx) { 00126 return BUTTON_GetDefaultTextColor(idx); 00127 } 00128 00129 void EwButton::setDefaultTextColor(ewButtonColorIndex_t idx, ewColor_t c) { 00130 BUTTON_SetDefaultTextColor(c, idx); 00131 } 00132 00133 void EwButton::setDefaultFocusColor(ewColor_t c) { 00134 BUTTON_SetDefaultFocusColor(c); 00135 } 00136 00137 00138 void EwButton::init(int x, int y, int width, int height, EwWindow* parent) { 00139 BUTTON_Handle h; 00140 GUI_HWIN hWin = 0; 00141 00142 _inputStatePressed = false; 00143 00144 if (parent) { 00145 hWin = _getHandle(parent); 00146 } 00147 00148 h = BUTTON_CreateEx(x, y, width, height, hWin, (WM_CF_SHOW| WM_CF_MEMDEV), 00149 0, _guiWidgetId++); 00150 _setNewHandle(h, _callback); 00151 } 00152 00153 void EwButton::handleInput(bool pressed, bool onWidget) { 00154 00155 // pressed will always be on top of widget 00156 if (pressed && !_inputStatePressed) { 00157 _pressedListener.call(this); 00158 } 00159 00160 // always generate a released event (even if not released on top of widget) 00161 if (!pressed && _inputStatePressed) { 00162 _releasedListener.call(this); 00163 } 00164 00165 if (!pressed && _inputStatePressed && onWidget) { 00166 _clickedListener.call(this); 00167 } 00168 00169 _inputStatePressed = pressed; 00170 } 00171 00172 void EwButton::_callback(WM_MESSAGE* pMsg, EwWindow* w) { 00173 GUI_PID_STATE* pidState; 00174 00175 // always let button widget process the event first 00176 BUTTON_Callback(pMsg); 00177 00178 switch(pMsg->MsgId) { 00179 case WM_TOUCH: 00180 pidState = (GUI_PID_STATE*)pMsg->Data.p; 00181 00182 EwButton* b = (EwButton*)w; 00183 b->handleInput((pidState->Pressed == 1), 00184 (pidState->Pressed == 1 || pidState->Pressed == 0)); 00185 00186 00187 break; 00188 } 00189 00190 } 00191
Generated on Tue Jul 12 2022 19:57:49 by
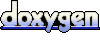