
A simple example using a display and networking at the same time.
Dependencies: EALib EthernetInterface mbed-rtos mbed
main.cpp
00001 /****************************************************************************** 00002 * Includes 00003 *****************************************************************************/ 00004 00005 #include "mbed.h" 00006 00007 #include "LcdController.h" 00008 #include "EaLcdBoard.h" 00009 #include "sdram.h" 00010 00011 #include "BubbleDemo.h" 00012 #include "EthernetInterface.h" 00013 #include "TCPSocketConnection.h" 00014 #include "cmsis_os.h" 00015 00016 00017 /****************************************************************************** 00018 * Typedefs and defines 00019 *****************************************************************************/ 00020 00021 #define RESET_FLAG \ 00022 do { \ 00023 if (abortTest) { \ 00024 abortTest = false; \ 00025 wait(0.04); \ 00026 } \ 00027 } while(false) 00028 00029 /****************************************************************************** 00030 * Local variables 00031 *****************************************************************************/ 00032 00033 static InterruptIn buttonInterrupt(P2_10); 00034 static DigitalOut led(LED1); 00035 static DigitalOut led3(LED3); 00036 00037 static BubbleDemo* bubbleDemo; 00038 00039 /****************************************************************************** 00040 * Global variables 00041 *****************************************************************************/ 00042 00043 EaLcdBoard lcdBoard(P0_27, P0_28); 00044 bool abortTest = false; 00045 00046 /****************************************************************************** 00047 * Interrupt functions 00048 *****************************************************************************/ 00049 00050 void trigger() { 00051 abortTest = true; 00052 } 00053 00054 /****************************************************************************** 00055 * Main 00056 *****************************************************************************/ 00057 00058 void bubble_thread(void const *argument) { 00059 while (true) { 00060 bubbleDemo->run(750, 20); 00061 RESET_FLAG; 00062 } 00063 } 00064 void net_thread(void const *argument) { 00065 EthernetInterface eth; 00066 eth.init(); //Use DHCP 00067 eth.connect(); 00068 printf("IP Address is %s\n", eth.getIPAddress()); 00069 TCPSocketConnection sock; 00070 while (true) { 00071 led3 = !led3; 00072 sock.connect("mbed.org", 80); 00073 sock.set_blocking(false, 3000); 00074 00075 char http_cmd[] = "GET /media/uploads/donatien/hello.txt HTTP/1.1\r\nHost: %s\r\n\r\n"; 00076 printf("sending:\n---\n%s\n---\n", http_cmd); 00077 sock.send(http_cmd, sizeof(http_cmd) - 1);//, 3000); 00078 00079 char in_buf[256]; 00080 bool firstIteration = true; 00081 int ret; 00082 do { 00083 printf("reading...\n"); 00084 ret = sock.receive(in_buf, 255);//, firstIteration?3000:0); 00085 in_buf[ret] = '\0'; 00086 00087 printf("Received %d chars from server: %s\n", ret, in_buf); 00088 firstIteration = false; 00089 } while ( ret == 255 ); // once we get less that full buffer we know all data has been received 00090 // } while ( ret > 0 ); 00091 00092 sock.close(); 00093 00094 //eth.disconnect(); 00095 00096 led3 = !led3; 00097 osDelay(500); 00098 } 00099 } 00100 00101 osThreadDef(bubble_thread, osPriorityNormal, DEFAULT_STACK_SIZE); 00102 osThreadDef(net_thread, osPriorityNormal, DEFAULT_STACK_SIZE); 00103 00104 int main (void) { 00105 00106 EaLcdBoard::Result result; 00107 LcdController::Config lcdCfg; 00108 uint32_t frameBuf1 = (uint32_t) SDRAM_BASE; 00109 00110 printf("Simple example of using network (HTTP GET) and LCD at the same time\n"); 00111 00112 // Listen for button presses 00113 buttonInterrupt.mode(PullUp); 00114 buttonInterrupt.fall(&trigger); 00115 00116 do { 00117 // framebuffer is put in SDRAM 00118 if (sdram_init() == 1) { 00119 printf("Failed to initialize SDRAM\n"); 00120 break; 00121 } 00122 00123 result = lcdBoard.open(NULL, NULL); 00124 if (result != EaLcdBoard::Ok) { 00125 printf("Failed to open display: %d\n", result); 00126 break; 00127 } 00128 00129 result = lcdBoard.setFrameBuffer(frameBuf1); 00130 if (result != EaLcdBoard::Ok) { 00131 printf("Failed to activate frameBuffer: %d\n", result); 00132 break; 00133 } 00134 00135 result = lcdBoard.getLcdConfig(&lcdCfg); 00136 if (result != EaLcdBoard::Ok) { 00137 printf("Failed to get LCD configuration: %d\n", result); 00138 break; 00139 } 00140 00141 // Prepare 3 consequtive framebuffers (2 will be used for background buffers) 00142 memset((void*)frameBuf1, 0x0, lcdCfg.width*lcdCfg.height*2 *3); 00143 00144 bubbleDemo = new BubbleDemo((uint8_t *)frameBuf1, lcdCfg.width, lcdCfg.height); 00145 osThreadCreate(osThread(bubble_thread), NULL); 00146 00147 osThreadCreate(osThread(net_thread), NULL); 00148 00149 while (true) { 00150 led = 0; 00151 wait(0.1); 00152 led = 1; 00153 wait(0.5); 00154 } 00155 } while(0); 00156 00157 // Blink to indicate error 00158 while (1) { 00159 led = 0; 00160 wait(0.2); 00161 led = 1; 00162 wait(0.2); 00163 } 00164 }
Generated on Wed Jul 13 2022 19:05:43 by
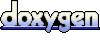