
The purpose of this application is to allow easy manipulation of the QSPI file system from a PC
Dependencies: EALib USBDevice mbed
crc.cpp
00001 /***************************************************************************** 00002 * 00003 * Copyright(C) 2011, Embedded Artists AB 00004 * All rights reserved. 00005 * 00006 ****************************************************************************** 00007 * Software that is described herein is for illustrative purposes only 00008 * which provides customers with programming information regarding the 00009 * products. This software is supplied "AS IS" without any warranties. 00010 * Embedded Artists AB assumes no responsibility or liability for the 00011 * use of the software, conveys no license or title under any patent, 00012 * copyright, or mask work right to the product. Embedded Artists AB 00013 * reserves the right to make changes in the software without 00014 * notification. Embedded Artists AB also make no representation or 00015 * warranty that such application will be suitable for the specified 00016 * use without further testing or modification. 00017 *****************************************************************************/ 00018 00019 00020 00021 /****************************************************************************** 00022 * Includes 00023 *****************************************************************************/ 00024 00025 #include "mbed.h" 00026 #include "crc.h" 00027 00028 00029 /****************************************************************************** 00030 * Defines and typedefs 00031 *****************************************************************************/ 00032 00033 #define NUM_CRC_BUFF_ENTRIES (100) 00034 00035 #define CRC32_INIT() do { LPC_CRC->MODE = 0x00000036; LPC_CRC->SEED = 0xffffffff; } while(0) 00036 00037 #define CRC32_WRITE8(__val) LPC_CRC->WR_DATA_BYTE.DATA = (uint8_t)(__val) 00038 #define CRC32_WRITE16(__val) LPC_CRC->WR_DATA_WORD.DATA = (uint16_t)(__val) 00039 #define CRC32_WRITE32(__val) LPC_CRC->WR_DATA_DWORD.DATA = (__val) 00040 00041 #define CRC32_SUM() LPC_CRC->SUM 00042 00043 /****************************************************************************** 00044 * External global variables 00045 *****************************************************************************/ 00046 00047 /****************************************************************************** 00048 * Local variables 00049 *****************************************************************************/ 00050 00051 static uint32_t crcbuff[NUM_CRC_BUFF_ENTRIES]; 00052 00053 /****************************************************************************** 00054 * Local Functions 00055 *****************************************************************************/ 00056 00057 00058 /****************************************************************************** 00059 * Public Functions 00060 *****************************************************************************/ 00061 00062 uint32_t crc_Read(FILE* f) 00063 { 00064 CRC32_INIT(); 00065 fseek(f, 0, SEEK_SET); 00066 memset(crcbuff, 0, sizeof(uint32_t)*NUM_CRC_BUFF_ENTRIES); 00067 int numRead = fread(crcbuff, sizeof(uint32_t), 100, f); 00068 while (numRead > 0) { 00069 for (int i = 0; i < numRead; i++) { 00070 CRC32_WRITE32(crcbuff[i]); 00071 } 00072 numRead = fread(crcbuff, sizeof(uint32_t), 100, f); 00073 } 00074 return CRC32_SUM(); 00075 } 00076
Generated on Wed Jul 13 2022 17:18:12 by
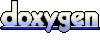