
Example for the LPC4088 QSB Base Board
Embed:
(wiki syntax)
Show/hide line numbers
CubeDemo.h
00001 00002 #ifndef CUBEDEMO_H 00003 #define CUBEDEMO_H 00004 00005 #include "Graphics.h" 00006 #include "GFXFb.h" 00007 00008 class CubeDemo { 00009 public: 00010 00011 /** Set the address of the frame buffer to use. 00012 * 00013 * It is the content of the frame buffer that is shown on the 00014 * display. All the drawing on the frame buffer can be done 00015 * 'offline' and whenever it should be shown this function 00016 * can be called with the address of the offline frame buffer. 00017 * 00018 * @param pFrameBuf Pointer to the frame buffer, which must be 00019 * 3 times as big as the frame size (for tripple 00020 * buffering). 00021 * dispWidth The width of the display (in pixels). 00022 * dispHeight The height of the display (in pixels). 00023 * loops Number of loops in the demo code. 00024 * delayMs Delay in milliseconds between schreen updates. 00025 * 00026 * @returns 00027 * none 00028 */ 00029 CubeDemo(uint8_t *pFrameBuf, uint16_t dispWidth, uint16_t dispHeight); 00030 ~CubeDemo(); 00031 00032 void run(uint32_t loops, uint32_t delayMs); 00033 00034 00035 private: 00036 enum Constants { 00037 BACKGROUND_COLOR = BLACK, 00038 SMALL_CIRCLE_FRONT_COLOR = WHITE, 00039 00040 LENS = 256, 00041 X_OFFSET = 240, //120, 00042 Y_OFFSET = 130 00043 }; 00044 00045 typedef struct 00046 { 00047 uint32_t w; 00048 uint32_t h; 00049 void *pixels; 00050 } Surface_t; 00051 00052 typedef struct 00053 { 00054 int x; 00055 int y; 00056 } Coord2D_t; 00057 00058 typedef struct 00059 { 00060 int32_t x; //normal 3d coords 00061 int32_t y; 00062 int32_t z; 00063 int32_t xr; //rotated coords 00064 int32_t yr; 00065 int32_t zr; 00066 int32_t scrx; //translated and projected 3d points 00067 int32_t scry; 00068 } tPoint3d; 00069 00070 typedef struct 00071 { 00072 int32_t p1; //vertex 00073 int32_t p2; 00074 int32_t p3; 00075 int32_t u1; //texture coords 00076 int32_t v1; 00077 int32_t u2; 00078 int32_t v2; 00079 int32_t u3; 00080 int32_t v3; 00081 } tPoly; 00082 00083 typedef struct 00084 { 00085 int32_t x; 00086 int32_t y; 00087 int32_t z; 00088 } tVector; 00089 00090 int32_t windowX; 00091 int32_t windowY; 00092 uint16_t *pFrmBuf; 00093 uint16_t *pFrmBuf1; 00094 uint16_t *pFrmBuf2; 00095 uint16_t *pFrmBuf3; 00096 00097 Graphics graphics; 00098 00099 Surface_t sourcePicture1; 00100 Surface_t sourcePicture2; 00101 Surface_t activeFrame; 00102 00103 int32_t rx; 00104 int32_t ry; 00105 int32_t rx_; 00106 int32_t ry_; 00107 uint8_t mode; 00108 00109 tPoint3d cubeModel[8]; 00110 tPoly cubePoly[12]; 00111 00112 uint32_t camX; 00113 uint32_t camY; 00114 uint32_t camZ; 00115 00116 void plot4points( int32_t cx, int32_t cy, int32_t x, int32_t y, int16_t color, int32_t Filled ); 00117 void plot8points( int32_t cx, int32_t cy, int32_t x, int32_t y, int16_t color, int32_t Filled ); 00118 void put_circle( int32_t cx, int32_t cy, int16_t color, int32_t radius, int32_t Filled ); 00119 int32_t swim_abs(int32_t v1); 00120 void put_line(int32_t x1, int32_t y1, int32_t x2, int32_t y2, int16_t color); 00121 00122 int32_t sgn(int32_t val) const; 00123 short icosine(short x) const; 00124 short isine(short x) const; 00125 short _cos(short y) const; 00126 short _sin(short y) const; 00127 00128 void TriangleProjectFast(Surface_t *SrcRP_p, Surface_t *DstRP_p, Coord2D_t *SrcCoords_p, Coord2D_t *DstCoords_p); 00129 void CpPixel16Fast(int xSrc, int ySrc, int x, int y, Surface_t *SrcRP_p, Surface_t *DstRP_p); 00130 00131 void drawCubeZ(Surface_t *pSourcePicture, uint8_t shades); 00132 void rotateAndProject(uint16_t rotX, uint16_t rotY, uint16_t rotZ); 00133 void createCubeModel(uint32_t radius); 00134 00135 unsigned decodePNG(const unsigned char* pIn, size_t insize, Surface_t* pOut); 00136 00137 00138 void initialize(); 00139 void render(uint32_t idx); 00140 }; 00141 00142 00143 #endif /* CUBEDEMO_H */ 00144
Generated on Fri Jul 15 2022 14:51:32 by
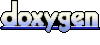