
Example for the LPC4088 QSB Base Board
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /****************************************************************************** 00002 * Includes 00003 *****************************************************************************/ 00004 00005 #include "mbed.h" 00006 00007 #include "LcdController.h" 00008 #include "EaLcdBoard.h" 00009 #include "sdram.h" 00010 00011 #include "BubbleDemo.h" 00012 00013 00014 /****************************************************************************** 00015 * Typedefs and defines 00016 *****************************************************************************/ 00017 00018 #define RESET_FLAG \ 00019 do { \ 00020 if (abortTest) { \ 00021 abortTest = false; \ 00022 wait(0.04); \ 00023 } \ 00024 } while(false) 00025 00026 /****************************************************************************** 00027 * Local variables 00028 *****************************************************************************/ 00029 00030 static InterruptIn buttonInterrupt(P2_10); 00031 static DigitalOut led(LED1); 00032 00033 /****************************************************************************** 00034 * Global variables 00035 *****************************************************************************/ 00036 00037 EaLcdBoard lcdBoard(P0_27, P0_28); 00038 bool abortTest = false; 00039 00040 /****************************************************************************** 00041 * Interrupt functions 00042 *****************************************************************************/ 00043 00044 void trigger() { 00045 abortTest = true; 00046 } 00047 00048 /****************************************************************************** 00049 * Main 00050 *****************************************************************************/ 00051 00052 int main (void) { 00053 00054 EaLcdBoard::Result result; 00055 LcdController::Config lcdCfg; 00056 uint32_t frameBuf1 = (uint32_t) SDRAM_BASE; 00057 00058 printf("EA LCD Board Bubbles Demo\n"); 00059 00060 // Listen for button presses 00061 buttonInterrupt.mode(PullUp); 00062 buttonInterrupt.fall(&trigger); 00063 00064 do { 00065 // framebuffer is put in SDRAM 00066 if (sdram_init() == 1) { 00067 printf("Failed to initialize SDRAM\n"); 00068 break; 00069 } 00070 00071 result = lcdBoard.open(NULL, NULL); 00072 if (result != EaLcdBoard::Ok) { 00073 printf("Failed to open display: %d\n", result); 00074 break; 00075 } 00076 00077 result = lcdBoard.setFrameBuffer(frameBuf1); 00078 if (result != EaLcdBoard::Ok) { 00079 printf("Failed to activate frameBuffer: %d\n", result); 00080 break; 00081 } 00082 00083 result = lcdBoard.getLcdConfig(&lcdCfg); 00084 if (result != EaLcdBoard::Ok) { 00085 printf("Failed to get LCD configuration: %d\n", result); 00086 break; 00087 } 00088 00089 // Prepare 3 consequtive framebuffers (2 will be used for background buffers) 00090 memset((void*)frameBuf1, 0x0, lcdCfg.width*lcdCfg.height*2 *3); 00091 00092 BubbleDemo bubbleDemo((uint8_t *)frameBuf1, lcdCfg.width, lcdCfg.height); 00093 while (1) { 00094 bubbleDemo.run(750, 20); 00095 RESET_FLAG; 00096 } 00097 } while(0); 00098 00099 // Blink to indicate error 00100 while (1) { 00101 led = 0; 00102 wait(0.2); 00103 led = 1; 00104 wait(0.2); 00105 } 00106 }
Generated on Mon Jul 18 2022 21:55:07 by
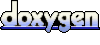