
Example using the GFX graphical library, EaLcdBoard, TSC2046 touch screen and SDRAM initialization
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 /****************************************************************************** 00003 * Includes 00004 *****************************************************************************/ 00005 00006 #include "mbed.h" 00007 00008 00009 #include "LcdController.h" 00010 #include "EaLcdBoard.h" 00011 #include "TSC2046.h" 00012 #include "AR1021.h" 00013 #include "sdram.h" 00014 00015 #include "wchar.h" 00016 #include "GFXFb.h" 00017 00018 00019 00020 /****************************************************************************** 00021 * Typedefs and defines 00022 *****************************************************************************/ 00023 00024 00025 /****************************************************************************** 00026 * Local variables 00027 *****************************************************************************/ 00028 00029 // EA LCD Board interface 00030 static EaLcdBoard lcdBoard(P0_27, P0_28); 00031 00032 static uint16_t const colors[16] = { 00033 BLACK, 00034 LIGHTGRAY, 00035 DARKGRAY, 00036 WHITE, 00037 RED, 00038 GREEN, 00039 BLUE, 00040 MAGENTA, 00041 CYAN, 00042 YELLOW, 00043 LIGHTRED, 00044 LIGHTGREEN, 00045 LIGHTBLUE, 00046 LIGHTMAGENTA, 00047 LIGHTCYAN, 00048 LIGHTYELLOW 00049 }; 00050 00051 /****************************************************************************** 00052 * Local functions 00053 *****************************************************************************/ 00054 00055 static uint16_t random(uint16_t max) 00056 { 00057 uint16_t temp; 00058 00059 temp = rand(); 00060 temp = temp % max; 00061 return temp; 00062 } 00063 00064 static void demo1(GFXFb &gfx) { 00065 00066 int16_t x0 = 0; 00067 int16_t y0 = 0; 00068 int16_t radius = 0; 00069 int color = 0; 00070 int fill = 0; 00071 00072 00073 gfx.fillScreen(BLACK); 00074 00075 for (int i = 0; i < 100; i++) { 00076 x0 = random(gfx.width()); 00077 y0 = random(gfx.height()); 00078 color = random(16); 00079 radius = random(50); 00080 fill = random(2); 00081 00082 if (!fill) { 00083 gfx.drawCircle(x0, y0, radius, colors[color]); 00084 } else { 00085 gfx.fillCircle(x0, y0, radius, colors[color]); 00086 } 00087 00088 wait_ms(10); 00089 } 00090 00091 } 00092 00093 00094 static void demo2(GFXFb &gfx) { 00095 int32_t margin = 5; 00096 int32_t rowHeight = gfx.height() / 3; 00097 int32_t colWidth = gfx.width() / 3; 00098 int32_t graphPosX = gfx.getStringWidth("drawRoundRect"); 00099 int32_t maxGraphW = colWidth - graphPosX; 00100 00101 gfx.fillScreen(BLACK); 00102 00103 // ############## 00104 // C O L U M N 1 00105 // ############## 00106 00107 // drawLine 00108 gfx.setCursor(0, rowHeight/2); 00109 gfx.writeString("drawLine"); 00110 gfx.drawLine(0+graphPosX+margin, margin, 00111 0+graphPosX+maxGraphW-margin, rowHeight-margin, colors[1]); 00112 00113 // drawRect 00114 gfx.setCursor(0, rowHeight+rowHeight/2); 00115 gfx.writeString("drawRect"); 00116 gfx.drawRect(0+graphPosX+margin, rowHeight+margin, 00117 maxGraphW-2*margin, rowHeight-margin, colors[2]); 00118 00119 // fillRect 00120 gfx.setCursor(0, rowHeight*2+rowHeight/2); 00121 gfx.writeString("fillRect"); 00122 gfx.fillRect(0+graphPosX+margin, rowHeight*2+margin, 00123 maxGraphW-2*margin, rowHeight-margin, colors[3]); 00124 00125 // ############## 00126 // C O L U M N 2 00127 // ############## 00128 00129 // drawCircle 00130 gfx.setCursor(colWidth, rowHeight/2); 00131 gfx.writeString("drawCircle"); 00132 gfx.drawCircle(colWidth+graphPosX+maxGraphW/2, rowHeight/2, 00133 rowHeight/2-2*margin, colors[4]); 00134 00135 // fillCircle 00136 gfx.setCursor(colWidth, rowHeight+rowHeight/2); 00137 gfx.writeString("fillCircle"); 00138 gfx.fillCircle(colWidth+graphPosX+maxGraphW/2, rowHeight+rowHeight/2, 00139 rowHeight/2-2*margin, colors[5]); 00140 00141 // drawTriangle 00142 gfx.setCursor(colWidth, rowHeight*2+rowHeight/2); 00143 gfx.writeString("drawTriangle"); 00144 gfx.drawTriangle(colWidth+graphPosX+margin, rowHeight*3-margin, 00145 colWidth+graphPosX+maxGraphW/2, rowHeight*2+margin, 00146 colWidth+graphPosX+maxGraphW-margin, rowHeight*3-margin, colors[6]); 00147 00148 // ############## 00149 // C O L U M N 3 00150 // ############## 00151 00152 // fillTriangle 00153 gfx.setCursor(colWidth*2, rowHeight/2); 00154 gfx.writeString("fillTriangle"); 00155 gfx.fillTriangle(colWidth*2+graphPosX+margin, rowHeight-margin, 00156 colWidth*2+graphPosX+maxGraphW/2, margin, 00157 colWidth*2+graphPosX+maxGraphW-margin, rowHeight-margin, colors[7]); 00158 00159 00160 // drawRoundRect 00161 gfx.setCursor(colWidth*2, rowHeight+rowHeight/2); 00162 gfx.writeString("drawRoundRect"); 00163 gfx.drawRoundRect(colWidth*2+graphPosX+margin, rowHeight+margin, 00164 maxGraphW-2*margin, rowHeight-margin, 10, colors[8]); 00165 00166 // fillRoundRect 00167 gfx.setCursor(colWidth*2, rowHeight*2+rowHeight/2); 00168 gfx.writeString("fillRoundRect"); 00169 gfx.fillRoundRect(colWidth*2+graphPosX+margin, rowHeight*2+margin, 00170 maxGraphW-2*margin, rowHeight-margin, 10, colors[9]); 00171 00172 00173 } 00174 00175 static void demo3(GFXFb &gfx, TouchPanel* touchPanel) { 00176 00177 uint16_t x = 0; 00178 uint16_t y = 0; 00179 bool hasMorePoints = false; 00180 TouchPanel::touchCoordinate_t coord; 00181 00182 00183 do { 00184 if (!touchPanel->init(gfx.width(), gfx.height())) { 00185 printf("TouchPanel.init failed\n"); 00186 break; 00187 } 00188 00189 printf("Starting calibration\n"); 00190 if (!touchPanel->calibrateStart()) { 00191 printf("Failed to start calibration\n"); 00192 break; 00193 } 00194 00195 00196 do { 00197 if (!touchPanel->getNextCalibratePoint(&x, &y)) { 00198 printf("Failed to get next calibrate point\n"); 00199 break; 00200 } 00201 00202 printf("calib: x=%d, y=%d\n", x, y); 00203 gfx.fillScreen(BLACK); 00204 gfx.setCursor(0, 0); 00205 gfx.writeString("Calibrate Touch Screen"); 00206 gfx.drawRect(x-5, y-5, 10, 10, WHITE); 00207 00208 00209 if (!touchPanel->waitForCalibratePoint(&hasMorePoints, 0)) { 00210 printf("Failed waiting for calibration point\n"); 00211 break; 00212 } 00213 00214 } while(hasMorePoints); 00215 00216 printf("Calibration done\n"); 00217 00218 gfx.fillScreen(BLACK); 00219 00220 00221 while(1) { 00222 touchPanel->read(coord); 00223 if (coord.z > 0) { 00224 gfx.drawPixel(coord.x, coord.y, WHITE); 00225 } 00226 wait_ms(2); 00227 } 00228 00229 } while (0); 00230 00231 00232 00233 while(1); 00234 } 00235 00236 /****************************************************************************** 00237 * Main function 00238 *****************************************************************************/ 00239 00240 00241 int main (void) { 00242 bool initSuccessful = false; 00243 00244 EaLcdBoard::Result result; 00245 LcdController::Config lcdCfg; 00246 EaLcdBoard::TouchParams_t touchParams; 00247 uint32_t frameBuf1 = 0; 00248 TouchPanel* tp = NULL; 00249 00250 // frame buffer is put in SDRAM 00251 if (sdram_init() == 1) { 00252 printf("Failed to initialize SDRAM\n"); 00253 return 1; 00254 } 00255 00256 do { 00257 00258 result = lcdBoard.open(NULL, NULL); 00259 if (result != EaLcdBoard::Ok) { 00260 printf("Failed to open display: %d\n", result); 00261 break; 00262 } 00263 00264 00265 result = lcdBoard.getLcdConfig(&lcdCfg); 00266 if (result != EaLcdBoard::Ok) { 00267 printf("Failed to get LCD configuration: %d\n", result); 00268 break; 00269 } 00270 00271 00272 // allocate framebuffer, width x height x 2 (2 bytes = 16 bit color data) 00273 frameBuf1 = (uint32_t)malloc(lcdCfg.width*lcdCfg.height*2); 00274 if (frameBuf1 == 0) { 00275 printf("Failed to allocate frame buffer\n"); 00276 break; 00277 } 00278 00279 00280 result = lcdBoard.setFrameBuffer(frameBuf1); 00281 if (result != EaLcdBoard::Ok) { 00282 printf("Failed to activate frameBuffer: %d\n", result); 00283 break; 00284 } 00285 00286 00287 memset((void*)frameBuf1, 0x0, lcdCfg.width*lcdCfg.height*2); 00288 00289 result = lcdBoard.getTouchParameters(&touchParams); 00290 if (result != EaLcdBoard::Ok) { 00291 printf("Failed to get touch panel parameters: %d\n", result); 00292 break; 00293 } 00294 00295 // create touch panel 00296 switch(touchParams.panelId) { 00297 case EaLcdBoard::TouchPanel_AR1021: 00298 printf("creating AR1021 touch panel\n"); 00299 tp = new AR1021(P2_27, P2_26, P2_22, P2_21, P2_25); 00300 break; 00301 00302 case EaLcdBoard::TouchPanel_TSC2046: 00303 case EaLcdBoard::TouchPanelUnknown: 00304 default: 00305 // we always default to TSC2046 even if we cannot 00306 // detect which panel is used 00307 00308 printf("creating TSC2046 touch panel\n"); 00309 00310 tp = new TSC2046(P2_27, P2_26, P2_22, P2_21); 00311 break; 00312 00313 } 00314 00315 00316 initSuccessful = true; 00317 00318 00319 } while(0); 00320 00321 00322 00323 00324 if (initSuccessful) { 00325 00326 GFXFb gfx(lcdCfg.width, lcdCfg.height, (uint16_t*)frameBuf1); 00327 00328 while (1) { 00329 demo1(gfx); 00330 wait_ms(5000); 00331 demo2(gfx); 00332 wait_ms(5000); 00333 demo3(gfx, tp); 00334 } 00335 00336 } 00337 else { 00338 printf("Couldn't start demo -> Initialization failed\n"); 00339 } 00340 00341 00342 return 0; 00343 } 00344
Generated on Fri Jul 15 2022 21:58:31 by
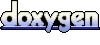