
Example code for the EM027BS013 ePaper display module.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "EM027BS013.h" 00003 #include "image_data.h" 00004 00005 /* The following macros makes it easier to specify all the 12 arguments to the 00006 * EM027BS013 constructor: 00007 */ 00008 00009 /* 00010 * LPC4088QSB_SEC 00011 * -------------- 00012 * Embedded Artists' LPC4088 QuickStart Board + LPC4088 QSB Base Board. 00013 * The display module should be connected using the 14-pos serial expansion 00014 * connector on the base board. 00015 */ 00016 #define LPC4088QSB_SEC p13,p11,p12,p14,p10,p9,p20,p19,p37,p38,p17,p18 00017 00018 /* 00019 * LPC4088QSB_ARDUINO 00020 * ------------------ 00021 * Embedded Artists' LPC4088 QuickStart Board + LPC4088 QSB Base Board. 00022 * The display module should be connected with jumper cables between the 00023 * Arduino pinlist on the base board and the 14-pos connector (J3) on the 00024 * display module like this: 00025 * 00026 * Arduino Display 00027 * 00028 * GND J3-1 00029 * AREF J3-2 00030 * D13 J3-3 00031 * D11 J3-4 00032 * D12 J3-5 00033 * D10 J3-6 00034 * D0 J3-7 00035 * D1 J3-8 00036 * SCL J3-9 00037 * SDA J3-10 00038 * D9 J3-11 00039 * D6 J3-12 00040 * D7 J3-13 00041 * D8 J3-14 00042 */ 00043 #define LPC4088QSB_ARDUINO D13,D11,D12,D10,D0,D1,P1_31,P1_30,D9,D6,D7,D8 00044 00045 /* 00046 * LPC11U68_ARDUINO 00047 * ---------------- 00048 * Connections for the LPCXpresso11U68 board. 00049 * The display module should be connected with jumper cables between the 00050 * Arduino pinlist on the LPCXpresso board and the 14-pos connector (J3) on the 00051 * display module like this: 00052 * 00053 * Arduino Display 00054 * 00055 * GND J3-1 00056 * AREF J3-2 00057 * D13 J3-3 00058 * D11 J3-4 00059 * D12 J3-5 00060 * D10 J3-6 00061 * D0 J3-7 00062 * D1 J3-8 00063 * SCL J3-9 00064 * SDA J3-10 00065 * D9 J3-11 00066 * D6 J3-12 00067 * D7 J3-13 00068 * D8 J3-14 00069 */ 00070 #define LPC11U68_ARDUINO D13,D11,D12,D10,D0,D1,SCL,SDA,D9,D6,D7,D8 00071 00072 /* 00073 * LPC1549_ARDUINO 00074 * --------------- 00075 * Connections for the LPCXpresso1549 board. 00076 * The display module should be connected with jumper cables between the 00077 * Arduino pinlist on the LPCXpresso board and the 14-pos connector (J3) on the 00078 * display module like this: 00079 * 00080 * Arduino Display 00081 * 00082 * GND J3-1 00083 * AREF J3-2 00084 * D13 J3-3 00085 * D11 J3-4 00086 * D12 J3-5 00087 * D10 J3-6 00088 * D0 J3-7 00089 * D1 J3-8 00090 * SCL J3-9 00091 * SDA J3-10 00092 * D9 J3-11 00093 * D6 J3-12 00094 * D7 J3-13 00095 * D8 J3-14 00096 */ 00097 #define LPC1549_ARDUINO D13,D11,D12,D10,D0,D1,SCL,SDA,D9,D6,D7,D8 00098 00099 /* 00100 * LPC768_MBED 00101 * ----------- 00102 * Connections for the mbed LPC1768 module. 00103 * The display module should be connected with jumper cables between the 00104 * pinlist on the mbed board and the 14-pos connector (J3) on the 00105 * display module like this: 00106 * 00107 * Mbed Display 00108 * 00109 * GND J3-1 00110 * VOUT J3-2 00111 * p7 J3-3 00112 * p5 J3-4 00113 * p6 J3-5 00114 * p8 J3-6 00115 * p13 J3-7 00116 * p10 J3-8 00117 * p27 J3-9 00118 * p28 J3-10 00119 * p26 J3-11 00120 * p12 J3-12 00121 * p9 J3-13 00122 * p11 J3-14 00123 */ 00124 #define LPC1768_MBED p7,p5,p6,p8,p13,p10,p27,p28,p26,p12,p9,p11 00125 00126 00127 EM027BS013 epaper(LPC1768_MBED); 00128 00129 int main() { 00130 while(1) { 00131 epaper.drawImage((uint8_t*)&image_array_270_ea); 00132 wait(5); 00133 epaper.drawImage((uint8_t*)&image_array_270_text); 00134 wait(5); 00135 epaper.drawImage((uint8_t*)&image_array_270_1); 00136 wait(5); 00137 epaper.drawImage((uint8_t*)&image_array_270_2); 00138 wait(5); 00139 } 00140 }
Generated on Fri Jul 15 2022 19:02:09 by
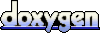