
Example that shows how to use the ewgui wrapper classes in combination with Segger's emwin library.
Dependencies: EALib ewgui mbed
MyWindow.cpp
00001 #include "mbed.h" 00002 00003 #include "MyWindow.h" 00004 #include "EwPainter.h" 00005 00006 MyWindow::MyWindow(EwWindow* parent) : 00007 EwWindow(5, 2, 300, 250, parent), 00008 _changeBtn(130, 200, 40, 20, this), 00009 _checkBox(0, 0, 80, 25, this), 00010 _dropDownLst(0, 0, 100, 50, this){ 00011 00012 _changeBtn.setText("Click"); 00013 _changeBtn.setClickedListener(this, &MyWindow::clickListener); 00014 00015 _checkBox.setText("CheckBox"); 00016 _checkBox.setChangedListener(this, &MyWindow::checkedListener); 00017 00018 _dropDownLst.addString("First"); 00019 _dropDownLst.addString("Second"); 00020 _dropDownLst.addString("Third"); 00021 00022 _clickCnt = 0; 00023 _pressed = false; 00024 _pressX = 0; 00025 _pressY = 0; 00026 00027 resizeTo(parent->getWidth()-15, parent->getHeight()-35); 00028 _changeBtn.moveTo(getWidth()/2-_changeBtn.getWidth()/2, 00029 getHeight()-_changeBtn.getHeight()-5); 00030 00031 _checkBox.moveTo(5, 35); 00032 00033 _dropDownLst.move(getWidth()-_dropDownLst.getWidth()-5, 5); 00034 } 00035 00036 bool MyWindow::paintEvent() { 00037 char buf[30]; 00038 EwPainter painter; 00039 00040 painter.setBackgroundColor(EW_LIGHTGRAY); 00041 painter.clear(); 00042 00043 if (_clickCnt > 0) { 00044 painter.setColor(EW_BLUE); 00045 if (_clickCnt == 1) { 00046 sprintf(buf, "Clicked %d time", _clickCnt); 00047 } 00048 else { 00049 sprintf(buf, "Clicked %d times", _clickCnt); 00050 } 00051 painter.drawStringClearEOL(buf, 5, 5); 00052 } 00053 00054 if (_pressed) { 00055 painter.setColor(GUI_DARKRED); 00056 sprintf(buf, "Touch at %d,%d", _pressX, _pressY); 00057 painter.drawStringClearEOL(buf, 5, 15); 00058 } 00059 00060 return true; 00061 } 00062 00063 bool MyWindow::touchEvent(int x, int y, EwTouchState_t state) { 00064 00065 if (state == TouchStatePressed) { 00066 _pressed = true; 00067 _pressX = x; 00068 _pressY = y; 00069 } 00070 else { 00071 _pressed = false; 00072 } 00073 00074 invalidate(); 00075 00076 return true; 00077 } 00078 00079 void MyWindow::clickListener(EwWindow* w) { 00080 _clickCnt++; 00081 invalidate(); 00082 } 00083 00084 void MyWindow::checkedListener(EwWindow* w) { 00085 EwCheckBox* b = (EwCheckBox*)w; 00086 00087 ewCheckBoxState_t state = b->getState(); 00088 00089 if (state == CheckBoxStateUnchecked) { 00090 b->setText("Unchecked"); 00091 } 00092 else { 00093 b->setText("Checked"); 00094 } 00095 00096 }
Generated on Fri Jul 15 2022 19:49:24 by
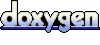