Interface to the MTK3339 GPS module
Dependents: app_gps lpc812_exp_solution_exp-port-gps-lib
MTK3339.h
00001 #ifndef MTK3339_H 00002 #define MTK3339_H 00003 00004 /** 00005 * An interface to the MTK3339 GPS module. 00006 */ 00007 class MTK3339 { 00008 public: 00009 00010 00011 enum NmeaSentence { 00012 NmeaInvalid = 0, 00013 NmeaGga = 0x01, 00014 // NmeaGsa = 0x02, 00015 // NmeaGsv = 0x04, 00016 // NmeaRmc = 0x08, 00017 NmeaVtg = 0x10 00018 }; 00019 00020 struct GgaType { 00021 /** UTC time - hours */ 00022 int hours; 00023 /** UTC time - minutes */ 00024 int minutes; 00025 /** UTC time - seconds */ 00026 int seconds; 00027 /** UTC time - milliseconds */ 00028 int milliseconds; 00029 00030 /** The latitude in ddmm.mmmm format (d = degrees, m = minutes) */ 00031 double latitude; 00032 /** The longitude in dddmm.mmmm format */ 00033 double longitude; 00034 /** North / South indicator */ 00035 char nsIndicator; 00036 /** East / West indicator */ 00037 char ewIndicator; 00038 00039 /** 00040 * Position indicator: 00041 * 0 = Fix not available 00042 * 1 = GPS fix 00043 * 2 = Differential GPS fix 00044 */ 00045 int fix; 00046 00047 /** Number of used satellites */ 00048 int satellites; 00049 /** Horizontal Dilution of Precision */ 00050 double hdop; 00051 /** antenna altitude above/below mean sea-level */ 00052 double altitude; 00053 /** geoidal separation */ 00054 double geoidal; 00055 }; 00056 00057 struct VtgType { 00058 /** heading in degrees */ 00059 double course; 00060 /** speed in Knots */ 00061 double speedKnots; 00062 /** Speed in kilometer per hour */ 00063 double speedKmHour; 00064 /** 00065 * Mode 00066 * A = Autonomous mode 00067 * D = Differential mode 00068 * E = Estimated mode 00069 */ 00070 char mode; 00071 }; 00072 00073 /** 00074 * Create an interface to the MTK3339 GPS module 00075 * 00076 * @param tx UART TX line pin 00077 * @param rx UART RX line pin 00078 */ 00079 MTK3339(PinName tx, PinName rx); 00080 00081 /** 00082 * Start to read data from the GPS module. 00083 * 00084 * @param fptr A pointer to a void function that will be called when there 00085 * is data available. 00086 * @param mask specifies which sentence types (NmeaSentence) that are of 00087 * interest. The callback function will only be called for messages 00088 * specified in this mask. 00089 */ 00090 void start(void (*fptr)(void), int mask); 00091 00092 /** 00093 * Start to read data from the GPS module. 00094 * 00095 * @param tptr pointer to the object to call the member function on 00096 * @param mptr pointer to the member function to be called 00097 * @param mask specifies which sentence types (NmeaSentence) that are of 00098 * interest. The member function will only be called for messages 00099 * specified in this mask. 00100 */ 00101 template<typename T> 00102 void start(T* tptr, void (T::*mptr)(void), int mask) { 00103 if((mptr != NULL) && (tptr != NULL) && mask) { 00104 _dataCallback.attach(tptr, mptr); 00105 _sentenceMask = mask; 00106 _serial.attach(this, &MTK3339::uartIrq, Serial::RxIrq); 00107 } 00108 } 00109 00110 /** 00111 * Stop to read data from GPS module 00112 */ 00113 void stop(); 00114 00115 /** 00116 * Get the type of the data reported in available data callback. 00117 * This method will only return a valid type when called within the 00118 * callback. 00119 */ 00120 NmeaSentence getAvailableDataType(); 00121 00122 /** 00123 * Get latitude in degrees (decimal format) 00124 */ 00125 double getLatitudeAsDegrees(); 00126 /** 00127 * Get longitude in degrees (decimal format) 00128 */ 00129 double getLongitudeAsDegrees(); 00130 00131 /** 00132 * Time, position and fix related data 00133 */ 00134 GgaType gga; 00135 00136 /** 00137 * Course and speed information relative to ground 00138 */ 00139 VtgType vtg; 00140 00141 00142 private: 00143 00144 enum PrivConstants { 00145 MTK3339_BUF_SZ = 255 00146 }; 00147 00148 enum DataState { 00149 StateStart = 0, 00150 StateData 00151 }; 00152 00153 FunctionPointer _dataCallback; 00154 char _buf[MTK3339_BUF_SZ]; 00155 int _bufPos; 00156 DataState _state; 00157 int _sentenceMask; 00158 NmeaSentence _availDataType; 00159 00160 Serial _serial; 00161 00162 00163 void parseGGA(char* data, int dataLen); 00164 void parseVTG(char* data, int dataLen); 00165 void parseData(char* data, int len); 00166 void uartIrq(); 00167 00168 00169 }; 00170 00171 #endif 00172
Generated on Tue Jul 12 2022 23:30:58 by
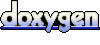