Modified version of the DmTftLibrary, optimized for the LPC4088 Experiment Base Board
Dependents: lpc4088_ebb_dm_calc lpc4088_ebb_dm_bubbles
Fork of DmTftLibrary by
DmTftIli9325.cpp
00001 /********************************************************************************************** 00002 Copyright (c) 2014 DisplayModule. All rights reserved. 00003 00004 Redistribution and use of this source code, part of this source code or any compiled binary 00005 based on this source code is permitted as long as the above copyright notice and following 00006 disclaimer is retained. 00007 00008 DISCLAIMER: 00009 THIS SOFTWARE IS SUPPLIED "AS IS" WITHOUT ANY WARRANTIES AND SUPPORT. DISPLAYMODULE ASSUMES 00010 NO RESPONSIBILITY OR LIABILITY FOR THE USE OF THE SOFTWARE. 00011 ********************************************************************************************/ 00012 #include "DmTftIli9325.h" 00013 00014 DmTftIli9325::DmTftIli9325(uint8_t wr, uint8_t cs, uint8_t dc, uint8_t rst) : DmTftBase(240, 320) { 00015 _wr = wr; 00016 _cs = cs; 00017 _dc = dc; 00018 _rst = rst; 00019 } 00020 00021 DmTftIli9325::~DmTftIli9325() { 00022 #if defined (DM_TOOLCHAIN_MBED) 00023 delete _pinRST; 00024 delete _pinCS; 00025 delete _pinWR; 00026 delete _pinDC; 00027 delete _virtualPortD; 00028 _pinRST = NULL; 00029 _pinCS = NULL; 00030 _pinWR = NULL; 00031 _pinDC = NULL; 00032 _virtualPortD = NULL; 00033 #endif 00034 } 00035 00036 void DmTftIli9325::writeBus(uint8_t data) { 00037 #if defined (DM_TOOLCHAIN_ARDUINO) 00038 PORTD = data; 00039 #elif defined (DM_TOOLCHAIN_MBED) 00040 *_virtualPortD = data; 00041 #endif 00042 pulse_low(_pinWR, _bitmaskWR); 00043 } 00044 00045 void DmTftIli9325::sendCommand(uint8_t index) { 00046 cbi(_pinDC, _bitmaskDC); 00047 writeBus(0x00); 00048 writeBus(index); 00049 } 00050 00051 void DmTftIli9325::sendData(uint16_t data) { 00052 sbi(_pinDC, _bitmaskDC); 00053 writeBus(data>>8); 00054 writeBus(data); 00055 } 00056 00057 void DmTftIli9325::setAddress(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1) { 00058 sendCommand(0x50); // Set Column 00059 sendData(x0); 00060 sendCommand(0x51); 00061 sendData(x1); 00062 00063 sendCommand(0x52); // Set Page 00064 sendData(y0); 00065 sendCommand(0x53); 00066 sendData(y1); 00067 00068 sendCommand(0x20); 00069 sendData(x0); 00070 sendCommand(0x21); 00071 sendData(y0); 00072 sendCommand(0x22); 00073 } 00074 00075 void DmTftIli9325::init(void) { 00076 setTextColor(BLACK, WHITE); 00077 #if defined (DM_TOOLCHAIN_ARDUINO) 00078 /************************************** 00079 DM-DmTftIli932522-102 Arduino UNO NUM 00080 00081 RST A2 16 00082 CS A3 17 00083 WR A4 18 00084 RS A5 19 00085 00086 DB8 0 0 00087 DB9 1 1 00088 DB10 2 2 00089 DB11 3 3 00090 DB12 4 4 00091 DB13 5 5 00092 DB14 6 6 00093 DB15 7 7 00094 00095 ***************************************/ 00096 DDRD = DDRD | B11111111; // SET PORT D AS OUTPUT 00097 00098 _pinRST = portOutputRegister(digitalPinToPort(_rst)); 00099 _bitmaskRST = digitalPinToBitMask(_rst); 00100 _pinCS = portOutputRegister(digitalPinToPort(_cs)); 00101 _bitmaskCS = digitalPinToBitMask(_cs); 00102 _pinWR = portOutputRegister(digitalPinToPort(_wr)); 00103 _bitmaskWR = digitalPinToBitMask(_wr); 00104 _pinDC = portOutputRegister(digitalPinToPort(_dc)); 00105 _bitmaskDC = digitalPinToBitMask(_dc); 00106 00107 pinMode(_rst, OUTPUT); 00108 pinMode(_cs, OUTPUT); 00109 pinMode(_wr, OUTPUT); 00110 pinMode(_dc,OUTPUT); 00111 #elif defined (DM_TOOLCHAIN_MBED) 00112 _pinRST = new DigitalOut((PinName)_rst); 00113 _pinCS = new DigitalOut((PinName)_cs); 00114 _pinWR = new DigitalOut((PinName)_wr); 00115 _pinDC = new DigitalOut((PinName)_dc); 00116 _virtualPortD = new BusOut(D0, D1, D2, D3, D4, SPECIAL_D5, D6, D7); 00117 #endif 00118 00119 sbi(_pinRST, _bitmaskRST); 00120 delay(5); 00121 cbi(_pinRST, _bitmaskRST); 00122 delay(15); 00123 sbi(_pinRST, _bitmaskRST); 00124 sbi(_pinCS, _bitmaskCS); 00125 sbi(_pinWR, _bitmaskWR); 00126 delay(15); 00127 cbi(_pinCS, _bitmaskCS); 00128 00129 sendCommand(0xE5); sendData(0x78F0); 00130 sendCommand(0x01); sendData(0x0100); 00131 sendCommand(0x02); sendData(0x0700); 00132 sendCommand(0x03); sendData(0x1030); 00133 sendCommand(0x04); sendData(0x0000); 00134 sendCommand(0x08); sendData(0x0207); 00135 sendCommand(0x09); sendData(0x0000); 00136 sendCommand(0x0A); sendData(0x0000); 00137 sendCommand(0x0C); sendData(0x0000); 00138 sendCommand(0x0D); sendData(0x0000); 00139 sendCommand(0x0F); sendData(0x0000); 00140 00141 sendCommand(0x10); sendData(0x0000); 00142 sendCommand(0x11); sendData(0x0007); 00143 sendCommand(0x12); sendData(0x0000); 00144 sendCommand(0x13); sendData(0x0000); 00145 00146 sendCommand(0x10); sendData(0x1290); 00147 sendCommand(0x11); sendData(0x0227); 00148 sendCommand(0x12); sendData(0x001D); 00149 sendCommand(0x13); sendData(0x1500); 00150 00151 sendCommand(0x29); sendData(0x0018); 00152 sendCommand(0x2B); sendData(0x000D); 00153 00154 sendCommand(0x30); sendData(0x0004); 00155 sendCommand(0x31); sendData(0x0307); 00156 sendCommand(0x32); sendData(0x0002); 00157 sendCommand(0x35); sendData(0x0206); 00158 sendCommand(0x36); sendData(0x0408); 00159 sendCommand(0x37); sendData(0x0507); 00160 sendCommand(0x38); sendData(0x0204); 00161 sendCommand(0x39); sendData(0x0707); 00162 sendCommand(0x3C); sendData(0x0405); 00163 sendCommand(0x3D); sendData(0x0f02); 00164 00165 sendCommand(0x50); sendData(0x0000); 00166 sendCommand(0x51); sendData(0x00EF); 00167 sendCommand(0x52); sendData(0x0000); 00168 sendCommand(0x53); sendData(0x013F); 00169 sendCommand(0x60); sendData(0xA700); 00170 sendCommand(0x61); sendData(0x0001); 00171 sendCommand(0x6A); sendData(0x0000); 00172 00173 sendCommand(0x80); sendData(0x0000); 00174 sendCommand(0x81); sendData(0x0000); 00175 sendCommand(0x82); sendData(0x0000); 00176 sendCommand(0x83); sendData(0x0000); 00177 sendCommand(0x84); sendData(0x0000); 00178 sendCommand(0x85); sendData(0x0000); 00179 00180 sendCommand(0x90); sendData(0x0010); 00181 sendCommand(0x92); sendData(0x0600); 00182 sendCommand(0x93); sendData(0x0003); 00183 sendCommand(0x95); sendData(0x0110); 00184 sendCommand(0x97); sendData(0x0000); 00185 sendCommand(0x98); sendData(0x0000); 00186 sendCommand(0x07); sendData(0x0133); 00187 sbi(_pinCS, _bitmaskCS); 00188 delay(500); 00189 clearScreen(); 00190 } 00191 00192 /********************************************************************************************************* 00193 END FILE 00194 *********************************************************************************************************/ 00195
Generated on Tue Jul 12 2022 19:30:31 by
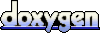