
This program simply connects to a HTS221 I2C device to proximity sensor
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include <cctype> 00003 #include <string> 00004 #include "SerialBuffered.h" 00005 #include "HTS221.h" 00006 #include "config_me.h" 00007 #include "sensors.h" 00008 #include "Proximity.h" 00009 #include "Wnc.h" 00010 00011 #include "hardware.h" 00012 00013 extern SerialBuffered mdm; 00014 Wnc wnc; 00015 00016 I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used 00017 00018 #define PROXIMITYON 1 00019 00020 #if PROXIMITYON == 1 00021 Proximity proximityStrip; 00022 #endif 00023 00024 // comment out the following line if color is not supported on the terminal 00025 #define USE_COLOR 00026 #ifdef USE_COLOR 00027 #define BLK "\033[30m" 00028 #define RED "\033[31m" 00029 #define GRN "\033[32m" 00030 #define YEL "\033[33m" 00031 #define BLU "\033[34m" 00032 #define MAG "\033[35m" 00033 #define CYN "\033[36m" 00034 #define WHT "\033[37m" 00035 #define DEF "\033[39m" 00036 #else 00037 #define BLK 00038 #define RED 00039 #define GRN 00040 #define YEL 00041 #define BLU 00042 #define MAG 00043 #define CYN 00044 #define WHT 00045 #define DEF 00046 #endif 00047 00048 #define MDM_DBG_OFF 0 00049 #define MDM_DBG_AT_CMDS (1 << 0) 00050 00051 #define MUXADDRESS 0x70 00052 #define PROXIMITYADDRESS 0x39 00053 00054 00055 00056 00057 int mdm_dbgmask = MDM_DBG_OFF; 00058 00059 Serial pc(USBTX, USBRX); 00060 00061 DigitalOut led_green(LED_GREEN); 00062 DigitalOut led_red(LED_RED); 00063 DigitalOut led_blue(LED_BLUE); 00064 00065 00066 00067 00068 DigitalIn slot1(PTB3,PullUp); 00069 DigitalIn slot2(PTB10,PullUp); 00070 DigitalIn slot3(PTB11,PullUp); 00071 00072 int lastSlot1; 00073 int lastSlot2; 00074 int lastSlot3; 00075 char *iccid; 00076 #define TOUPPER(a) (a) //toupper(a) 00077 00078 00079 #define MDM_OK 0 00080 #define MDM_ERR_TIMEOUT -1 00081 00082 #define MAX_AT_RSP_LEN 255 00083 00084 bool proximityChange = false; 00085 00086 bool toggleLed = false; 00087 int seqNum; 00088 00089 00090 //******************************************************************************************************************************************** 00091 //* Set the RGB LED's Color 00092 //* LED Color 0=Off to 7=White. 3 bits represent BGR (bit0=Red, bit1=Green, bit2=Blue) 00093 //******************************************************************************************************************************************** 00094 void SetLedColor(unsigned char ucColor) 00095 { 00096 if(wnc.isPowerSaveOn()) 00097 { 00098 led_red = !0; 00099 led_green = !0; 00100 led_blue = !(ucColor & 0x4); 00101 } 00102 else 00103 { 00104 //Note that when an LED is on, you write a 0 to it: 00105 led_red = !(ucColor & 0x1); //bit 0 00106 led_green = !(ucColor & 0x2); //bit 1 00107 led_blue = !(ucColor & 0x4); //bit 2 00108 } 00109 } //SetLedColor() 00110 00111 00112 void system_reset() 00113 { 00114 printf(RED "\n\rSystem resetting..." DEF "\n"); 00115 NVIC_SystemReset(); 00116 } 00117 00118 // These are built on the fly 00119 string MyServerIpAddress; 00120 string MySocketData; 00121 00122 // These are to be built on the fly 00123 string my_temp; 00124 string my_humidity; 00125 00126 #define CTOF(x) ((x)*1.8+32) 00127 00128 //******************************************************************************************************************************************** 00129 //* Create string with sensor readings that can be sent to flow as an HTTP get 00130 //******************************************************************************************************************************************** 00131 K64F_Sensors_t SENSOR_DATA = 00132 { 00133 .Temperature = "0", 00134 .Humidity = "0", 00135 .AccelX = "0", 00136 .AccelY = "0", 00137 .AccelZ = "0", 00138 .MagnetometerX = "0", 00139 .MagnetometerY = "0", 00140 .MagnetometerZ = "0", 00141 .AmbientLightVis = "0", 00142 .AmbientLightIr = "0", 00143 .UVindex = "0", 00144 .Proximity = "0", 00145 .Temperature_Si7020 = "0", 00146 .Humidity_Si7020 = "0" 00147 }; 00148 00149 void GenerateModemString(char * modem_string,int sensor) 00150 { 00151 switch(sensor) 00152 { 00153 #if PROXIMITYON == 1 00154 case PROXIMITY_ONLY: 00155 { 00156 00157 char* data = proximityStrip.getDataStr(); 00158 seqNum++; 00159 sprintf(modem_string, "GET %s%s?serial=%s&seq=%d&data=%s %s%s\r\n\r\n", FLOW_BASE_URL, "/shelf", iccid, seqNum, data, FLOW_URL_TYPE, MY_SERVER_URL); 00160 break; 00161 } 00162 #endif 00163 case SWITCH_ONLY: 00164 { 00165 char data[32]; 00166 sprintf(data,"[{\"p\":%d},{\"p\":%d},{\"p\":%d}]",lastSlot1*26,lastSlot2*26,lastSlot3*26); 00167 seqNum++; 00168 sprintf(modem_string, "GET %s%s?serial=%s&seq=%d&data=%s %s%s\r\n\r\n", FLOW_BASE_URL, "/car", iccid, seqNum, data, FLOW_URL_TYPE, MY_SERVER_URL); 00169 break; 00170 } 00171 case TEMP_HUMIDITY_ONLY: 00172 { 00173 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, FLOW_URL_TYPE, MY_SERVER_URL); 00174 break; 00175 } 00176 case TEMP_HUMIDITY_ACCELEROMETER: 00177 { 00178 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s&accelX=%s&accelY=%s&accelZ=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, SENSOR_DATA.AccelX,SENSOR_DATA.AccelY,SENSOR_DATA.AccelZ, FLOW_URL_TYPE, MY_SERVER_URL); 00179 break; 00180 } 00181 case TEMP_HUMIDITY_ACCELEROMETER_PMODSENSORS: 00182 { 00183 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s&accelX=%s&accelY=%s&accelZ=%s&proximity=%s&light_uv=%s&light_vis=%s&light_ir=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, SENSOR_DATA.AccelX,SENSOR_DATA.AccelY,SENSOR_DATA.AccelZ, SENSOR_DATA.Proximity, SENSOR_DATA.UVindex, SENSOR_DATA.AmbientLightVis, SENSOR_DATA.AmbientLightIr, FLOW_URL_TYPE, MY_SERVER_URL); 00184 break; 00185 } 00186 default: 00187 { 00188 sprintf(modem_string, "Invalid sensor selected\r\n\r\n"); 00189 break; 00190 } 00191 } //switch(iSensorsToReport) 00192 } //GenerateModemString 00193 00194 00195 //******************************************************************************************************************************************** 00196 //* Process JSON response messages 00197 //******************************************************************************************************************************************** 00198 bool extract_reply(char* search_field, char* found_string) 00199 { 00200 char* beginquote; 00201 char* endquote; 00202 beginquote = strstr(search_field, "\r\n\r\n"); //start of data 00203 endquote = strchr(search_field, '\0'); 00204 if (beginquote != 0) 00205 { 00206 uint16_t ifoundlen; 00207 if (endquote != 0) 00208 { 00209 ifoundlen = (uint16_t) (endquote - beginquote) + 1; 00210 strncpy(found_string, beginquote, ifoundlen ); 00211 found_string[ifoundlen] = 0; //null terminate 00212 return true; 00213 } 00214 } 00215 00216 return false; 00217 00218 } //extract_reply 00219 00220 00221 void parse_reply(char* reply) 00222 { 00223 char *tokens[5]; 00224 int index = 0; 00225 tokens[index++] = strtok(reply," "); 00226 while( index < 5 ) 00227 { 00228 char* token = strtok(NULL, " "); 00229 if(token == NULL) break; 00230 tokens[index++] = token; 00231 } 00232 if(strcmp("PSM",tokens[1]) == 0) 00233 { 00234 00235 int t3412 = atoi(tokens[3]); 00236 int t3324 = atoi(tokens[4]); 00237 pc.printf("t3412 %d t3324 %d\r\n",t3412,t3324); 00238 // setTauTimer(t3412); 00239 //setActivityTimer(t3324); 00240 00241 if(strcmp("true",tokens[2]) == 0) 00242 { 00243 pc.printf("PSM ON\r\n"); 00244 wnc.setPowerSave(true,t3412,t3324); 00245 } 00246 else 00247 { 00248 pc.printf("PSM OFF\r\n"); 00249 wnc.setPowerSave(false,t3412,t3324); 00250 } 00251 } 00252 } 00253 00254 bool checkSlots() 00255 { 00256 bool changed = false; 00257 int s1 = !slot1; 00258 int s2 = !slot2; 00259 int s3 = !slot3; 00260 if(lastSlot1 != s1 || lastSlot2 != s2 ||lastSlot3 != s3) 00261 { 00262 pc.printf("slot 1 %d\r\n",s1); 00263 pc.printf("slot 2 %d\r\n",s2); 00264 pc.printf("slot 3 %d\r\n",s3); 00265 changed = true; 00266 } 00267 lastSlot1 = s1; 00268 lastSlot2 = s2; 00269 lastSlot3 = s3; 00270 return changed; 00271 } 00272 00273 int main() { 00274 00275 int i; 00276 00277 HTS221 hts221; 00278 pc.baud(115200); 00279 00280 void hts221_init(void); 00281 00282 // Set LED to RED until init finishes 00283 SetLedColor(0x1); 00284 00285 pc.printf(BLU "Hello World from AT&T Shape!\r\n\n\r"); 00286 pc.printf(GRN "Initialize the HTS221\n\r"); 00287 00288 i = hts221.begin(); 00289 if( i ) 00290 pc.printf(BLU "HTS221 Detected! (0x%02X)\n\r",i); 00291 else 00292 pc.printf(RED "HTS221 NOT DETECTED!!\n\r"); 00293 00294 printf("Temp is: %0.2f F \n\r",CTOF(hts221.readTemperature())); 00295 printf("Humid is: %02d %%\n\r",hts221.readHumidity()); 00296 00297 00298 // Initialize the modem 00299 printf(GRN "Modem initializing... will take up to 60 seconds" DEF "\r\n"); 00300 do { 00301 i=wnc.init(); 00302 if (!i) { 00303 pc.printf(RED "Modem initialization failed!" DEF "\n"); 00304 } 00305 } while (!i); 00306 00307 // wnc.setIn(); 00308 wnc.send("AT+CPSMS=0",WNC_WAIT_TIME_MS); 00309 00310 wnc.toggleWake(); 00311 00312 00313 iccid = wnc.getIccid(); 00314 printf(GRN "ICCID = %s" DEF "\r\n",iccid); 00315 00316 //Software init 00317 wnc.startInternet(); 00318 //wnc.ping("108.244.165.22"); 00319 00320 // Set LED BLUE for partial init 00321 SetLedColor(0x4); 00322 00323 wnc.setPowerSave(false,12*60*60,20); 00324 00325 #if PROXIMITYON == 1 00326 proximityStrip.init(); 00327 proximityStrip.on(); 00328 #endif 00329 00330 int count = 0; 00331 // Send and receive data perpetually 00332 while(1) { 00333 00334 wnc.checkPassthrough(); 00335 //sprintf(SENSOR_DATA.Temperature, "%0.2f", CTOF(hts221.readTemperature())); 00336 //sprintf(SENSOR_DATA.Humidity, "%02d", hts221.readHumidity()); 00337 // read_sensors(); //read available external sensors from a PMOD and the on-board motion sensor 00338 toggleLed = !toggleLed; 00339 if(toggleLed) 00340 SetLedColor(0x2); //green 00341 else 00342 SetLedColor(0); //off 00343 00344 #if PROXIMITYON == 1 00345 proximityStrip.scan(); 00346 #else 00347 bool slotsChanged = checkSlots(); 00348 #endif 00349 00350 00351 #if PROXIMITYON == 1 00352 if(count >= 5*60 || proximityStrip.changed(50)) 00353 #else 00354 if(count >= 5*60 || slotsChanged) 00355 #endif 00356 { 00357 if(wnc.isPowerSaveOn()) 00358 { 00359 wnc.wakeFromPowerSave(); 00360 //wnc.ping("108.244.165.22"); 00361 } 00362 count = 0; 00363 SetLedColor(0x04); //blue 00364 00365 char modem_string[512]; 00366 00367 #if PROXIMITYON == 1 00368 GenerateModemString(&modem_string[0],PROXIMITY_ONLY); 00369 #else 00370 GenerateModemString(&modem_string[0],SWITCH_ONLY); 00371 #endif 00372 00373 printf(BLU "Sending to modem : %s" DEF "\r\n", modem_string); 00374 00375 if(wnc.connect("108.244.165.22",5005)) 00376 { 00377 char * reply = wnc.writeSocket(modem_string); 00378 00379 char* mydata; 00380 int tries = 4; 00381 while(tries > 0) // wait for reply 00382 { 00383 tries--; 00384 mydata = wnc.readSocket(); 00385 if (strlen(mydata) > 0) 00386 break; 00387 00388 wait(0.2); 00389 } 00390 00391 if (strlen(mydata) > 0) 00392 { 00393 00394 SetLedColor(0x2); // green 00395 //only copy on sucessful send 00396 00397 printf(BLU "Read back : %s" DEF "\r\n", mydata); 00398 char datareply[512]; 00399 if (extract_reply(mydata, datareply)) 00400 { 00401 printf(GRN "JSON : %s" DEF "\r\n", datareply); 00402 parse_reply(datareply); 00403 } 00404 SetLedColor(0); // off 00405 } 00406 else // no reply reset 00407 { 00408 SetLedColor(0x1); //red 00409 system_reset(); 00410 } 00411 wnc.disconnect(); 00412 } 00413 else // failed to connect reset 00414 { 00415 SetLedColor(0x1); //red 00416 system_reset(); 00417 } 00418 if(wnc.isPowerSaveOn()) 00419 wnc.resumePowerSave(); 00420 } 00421 count++; 00422 wait(0.2); 00423 } //forever loop 00424 }
Generated on Tue Jul 12 2022 19:39:23 by
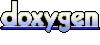