
This program simply connects to a HTS221 I2C device to proximity sensor
Embed:
(wiki syntax)
Show/hide line numbers
hts221_driver.cpp
00001 00002 #include "HTS221.h" 00003 00004 00005 // ------------------------------------------------------------------------------ 00006 //jmf -- define I2C pins and functions to read & write to I2C device 00007 00008 #include <string> 00009 #include "mbed.h" 00010 00011 #include "hardware.h" 00012 //I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used. Defined in a 00013 //common locatioin since sensors also use I2C 00014 00015 // Read a single unsigned char from addressToRead and return it as a unsigned char 00016 unsigned char HTS221::readRegister(unsigned char slaveAddress, unsigned char ToRead) 00017 { 00018 char data = ToRead; 00019 00020 i2c.write(slaveAddress, &data, 1, 1); 00021 i2c.read(slaveAddress, &data, 1, 0); 00022 return data; 00023 } 00024 00025 // Writes a single unsigned char (dataToWrite) into regToWrite 00026 int HTS221::writeRegister(unsigned char slaveAddress, unsigned char regToWrite, unsigned char dataToWrite) 00027 { 00028 const char data[] = {regToWrite, dataToWrite}; 00029 00030 return i2c.write(slaveAddress,data,2,0); 00031 } 00032 00033 00034 //jmf end 00035 // ------------------------------------------------------------------------------ 00036 00037 //static inline int humidityReady(uint8_t data) { 00038 // return (data & 0x02); 00039 //} 00040 //static inline int temperatureReady(uint8_t data) { 00041 // return (data & 0x01); 00042 //} 00043 00044 00045 HTS221::HTS221(void) : _address(HTS221_ADDRESS) 00046 { 00047 _temperature = 0; 00048 _humidity = 0; 00049 } 00050 00051 00052 int HTS221::begin(void) 00053 { 00054 uint8_t data; 00055 00056 data = readRegister(_address, WHO_AM_I); 00057 if (data == WHO_AM_I_RETURN){ 00058 if (activate()){ 00059 storeCalibration(); 00060 return data; 00061 } 00062 } 00063 00064 return 0; 00065 } 00066 00067 int 00068 HTS221::storeCalibration(void) 00069 { 00070 uint8_t data; 00071 uint16_t tmp; 00072 00073 for (int reg=CALIB_START; reg<=CALIB_END; reg++) { 00074 if ((reg!=CALIB_START+8) && (reg!=CALIB_START+9) && (reg!=CALIB_START+4)) { 00075 00076 data = readRegister(HTS221_ADDRESS, reg); 00077 00078 switch (reg) { 00079 case CALIB_START: 00080 _h0_rH = data; 00081 break; 00082 case CALIB_START+1: 00083 _h1_rH = data; 00084 break; 00085 case CALIB_START+2: 00086 _T0_degC = data; 00087 break; 00088 case CALIB_START+3: 00089 _T1_degC = data; 00090 break; 00091 00092 case CALIB_START+5: 00093 tmp = _T0_degC; 00094 _T0_degC = (data&0x3)<<8; 00095 _T0_degC |= tmp; 00096 00097 tmp = _T1_degC; 00098 _T1_degC = ((data&0xC)>>2)<<8; 00099 _T1_degC |= tmp; 00100 break; 00101 case CALIB_START+6: 00102 _H0_T0 = data; 00103 break; 00104 case CALIB_START+7: 00105 _H0_T0 |= data<<8; 00106 break; 00107 case CALIB_START+0xA: 00108 _H1_T0 = data; 00109 break; 00110 case CALIB_START+0xB: 00111 _H1_T0 |= data <<8; 00112 break; 00113 case CALIB_START+0xC: 00114 _T0_OUT = data; 00115 break; 00116 case CALIB_START+0xD: 00117 _T0_OUT |= data << 8; 00118 break; 00119 case CALIB_START+0xE: 00120 _T1_OUT = data; 00121 break; 00122 case CALIB_START+0xF: 00123 _T1_OUT |= data << 8; 00124 break; 00125 00126 00127 case CALIB_START+8: 00128 case CALIB_START+9: 00129 case CALIB_START+4: 00130 //DO NOTHING 00131 break; 00132 00133 // to cover any possible error 00134 default: 00135 return false; 00136 } /* switch */ 00137 } /* if */ 00138 } /* for */ 00139 return true; 00140 } 00141 00142 00143 int 00144 HTS221::activate(void) 00145 { 00146 uint8_t data; 00147 00148 data = readRegister(_address, CTRL_REG1); 00149 data |= POWER_UP; 00150 data |= ODR0_SET; 00151 writeRegister(_address, CTRL_REG1, data); 00152 00153 return true; 00154 } 00155 00156 00157 int HTS221::deactivate(void) 00158 { 00159 uint8_t data; 00160 00161 data = readRegister(_address, CTRL_REG1); 00162 data &= ~POWER_UP; 00163 writeRegister(_address, CTRL_REG1, data); 00164 return true; 00165 } 00166 00167 00168 int 00169 HTS221::bduActivate(void) 00170 { 00171 uint8_t data; 00172 00173 data = readRegister(_address, CTRL_REG1); 00174 data |= BDU_SET; 00175 writeRegister(_address, CTRL_REG1, data); 00176 00177 return true; 00178 } 00179 00180 00181 int 00182 HTS221::bduDeactivate(void) 00183 { 00184 uint8_t data; 00185 00186 data = readRegister(_address, CTRL_REG1); 00187 data &= ~BDU_SET; 00188 writeRegister(_address, CTRL_REG1, data); 00189 return true; 00190 } 00191 00192 00193 int 00194 HTS221::readHumidity(void) 00195 { 00196 uint8_t data = 0; 00197 uint16_t h_out = 0; 00198 double h_temp = 0.0; 00199 double hum = 0.0; 00200 00201 data = readRegister(_address, STATUS_REG); 00202 00203 if (data & HUMIDITY_READY) { 00204 data = readRegister(_address, HUMIDITY_H_REG); 00205 h_out = data << 8; // MSB 00206 data = readRegister(_address, HUMIDITY_L_REG); 00207 h_out |= data; // LSB 00208 00209 // Decode Humidity 00210 hum = ((int16_t)(_h1_rH) - (int16_t)(_h0_rH))/2.0; // remove x2 multiple 00211 00212 // Calculate humidity in decimal of grade centigrades i.e. 15.0 = 150. 00213 h_temp = (((int16_t)h_out - (int16_t)_H0_T0) * hum) / ((int16_t)_H1_T0 - (int16_t)_H0_T0); 00214 hum = ((int16_t)_h0_rH) / 2.0; // remove x2 multiple 00215 _humidity = (int16_t)((hum + h_temp)); // provide signed % measurement unit 00216 } 00217 return _humidity; 00218 } 00219 00220 00221 00222 double 00223 HTS221::readTemperature(void) 00224 { 00225 uint8_t data = 0; 00226 uint16_t t_out = 0; 00227 double t_temp = 0.0; 00228 double deg = 0.0; 00229 00230 data = readRegister(_address, STATUS_REG); 00231 00232 if (data & TEMPERATURE_READY) { 00233 00234 data= readRegister(_address, TEMP_H_REG); 00235 t_out = data << 8; // MSB 00236 data = readRegister(_address, TEMP_L_REG); 00237 t_out |= data; // LSB 00238 00239 // Decode Temperature 00240 deg = ((int16_t)(_T1_degC) - (int16_t)(_T0_degC))/8.0; // remove x8 multiple 00241 00242 // Calculate Temperature in decimal of grade centigrades i.e. 15.0 = 150. 00243 t_temp = (((int16_t)t_out - (int16_t)_T0_OUT) * deg) / ((int16_t)_T1_OUT - (int16_t)_T0_OUT); 00244 deg = ((int16_t)_T0_degC) / 8.0; // remove x8 multiple 00245 _temperature = deg + t_temp; // provide signed celsius measurement unit 00246 } 00247 00248 return _temperature; 00249 }
Generated on Tue Jul 12 2022 19:39:23 by
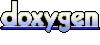