
This program simply connects to a HTS221 I2C device to proximity sensor
Embed:
(wiki syntax)
Show/hide line numbers
Wnc.h
00001 00002 #ifndef Wnc_ 00003 #define Wnc_ 00004 00005 #define WNC_WAIT_TIME_MS 500 00006 00007 00008 extern void SetLedColor(unsigned char ucColor); 00009 00010 00011 class Wnc { 00012 public: 00013 Wnc(void); 00014 int init(void); 00015 bool isModemResponding(); 00016 00017 char* read(int timeout_ms); 00018 char* send(const char *cmd, int timeout_ms); 00019 00020 00021 void setPowerSave(bool on,int t3412,int t3324); 00022 void resumePowerSave(); 00023 bool isPowerSaveOn(); 00024 void wakeFromPowerSave(); 00025 00026 char* getIccid(); 00027 void startInternet(); 00028 bool connect(char* ip, int port); 00029 void disconnect(); 00030 char* writeSocket(const char * s); 00031 char* readSocket(); 00032 char* ping(char* ip); 00033 void setIn(); 00034 void passthrough(); 00035 void checkPassthrough(); 00036 void toggleWake(); 00037 00038 private: 00039 int secToTau(int time); 00040 int secToActivity(int time); 00041 int hex_to_int(char c); 00042 int hex_to_ascii(char h, char l); 00043 int indexOf(char* str, char c); 00044 char* encode(int value, char* result, int base); 00045 }; 00046 00047 #endif
Generated on Tue Jul 12 2022 19:39:23 by
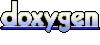