
This program simply connects to a HTS221 I2C device to proximity sensor
Embed:
(wiki syntax)
Show/hide line numbers
SerialBuffered.h
00001 #pragma once 00002 00003 // This is a buffered serial reading class, using the serial interrupt introduced in mbed library version 18 on 17/11/09 00004 00005 // In the simplest case, construct it with a buffer size at least equal to the largest message you 00006 // expect your program to receive in one go. 00007 00008 class SerialBuffered : public Serial 00009 { 00010 public: 00011 SerialBuffered( PinName tx, PinName rx, size_t bufferSize ); 00012 virtual ~SerialBuffered(); 00013 00014 int readable(); // returns 1 if there is a character available to read, 0 otherwise 00015 00016 void setTimeout( float seconds ); // maximum time in seconds that getc() should block 00017 // while waiting for a character 00018 // Pass -1 to disable the timeout. 00019 00020 size_t readBytes( uint8_t *bytes, size_t requested ); // read requested bytes into a buffer, 00021 // return number actually read, 00022 // which may be less than requested if there has been a timeout 00023 00024 void disable_flow_ctrl(void) { serial_set_flow_control(&_serial, FlowControlNone, NC, NC); } 00025 // void suspend(bool enable) { 00026 // if (enable) { 00027 // serial_irq_set(&_serial, (SerialIrq)RxIrq, 0); 00028 // serial_break_set(&_serial); 00029 // _serial.uart->ENABLE = (UART_ENABLE_ENABLE_Disabled << UART_ENABLE_ENABLE_Pos); 00030 // } else { 00031 // _serial.uart->ENABLE = (UART_ENABLE_ENABLE_Enabled << UART_ENABLE_ENABLE_Pos); 00032 // serial_break_clear(&_serial); 00033 // _serial.uart->EVENTS_RXDRDY = 0; 00034 // // dummy write needed or TXDRDY trails write rather than leads write. 00035 // // pins are disconnected so nothing is physically transmitted on the wire 00036 // _serial.uart->TXD = 0; 00037 // serial_irq_set(&_serial, (SerialIrq)RxIrq, 1); 00038 // } 00039 // } 00040 00041 protected: 00042 PinName _txpin; 00043 PinName _rxpin; 00044 00045 protected: 00046 virtual int _getc(); 00047 virtual int _putc(int c); 00048 00049 private: 00050 00051 void handleInterrupt(); 00052 00053 00054 uint8_t *m_buff; // points at a circular buffer, containing data from m_contentStart, for m_contentSize bytes, wrapping when you get to the end 00055 volatile uint16_t m_contentStart; // index of first bytes of content 00056 volatile uint16_t m_contentEnd; // index of bytes after last byte of content 00057 uint16_t m_buffSize; 00058 float m_timeout; 00059 Timer m_timer; 00060 00061 };
Generated on Tue Jul 12 2022 19:39:23 by
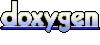