
This program simply connects to a HTS221 I2C device to proximity sensor
Embed:
(wiki syntax)
Show/hide line numbers
SerialBuffered.cpp
00001 00002 #include "mbed.h" 00003 #include "SerialBuffered.h" 00004 00005 SerialBuffered::SerialBuffered( PinName tx, PinName rx, size_t bufferSize ) : Serial( tx, rx ) 00006 { 00007 m_buffSize = 0; 00008 m_contentStart = 0; 00009 m_contentEnd = 0; 00010 m_timeout = -1.0; 00011 _txpin = tx; 00012 _rxpin = rx; 00013 00014 attach( this, &SerialBuffered::handleInterrupt ); 00015 00016 m_buff = (uint8_t *) malloc( bufferSize ); 00017 if( m_buff ) 00018 m_buffSize = bufferSize; 00019 } 00020 00021 00022 SerialBuffered::~SerialBuffered() 00023 { 00024 if( m_buff ) 00025 free( m_buff ); 00026 } 00027 00028 void SerialBuffered::setTimeout( float seconds ) 00029 { 00030 m_timeout = seconds; 00031 } 00032 00033 size_t SerialBuffered::readBytes( uint8_t *bytes, size_t requested ) 00034 { 00035 int i = 0; 00036 00037 for( ; i < requested; ) 00038 { 00039 int c = getc(); 00040 if( c < 0 ) 00041 break; 00042 bytes[i] = c; 00043 i++; 00044 } 00045 00046 return i; 00047 00048 } 00049 00050 00051 int SerialBuffered::_getc() 00052 { 00053 m_timer.reset(); 00054 m_timer.start(); 00055 while (m_contentStart == m_contentEnd) { 00056 if (m_timeout >= 0 && m_timer.read() >= m_timeout ) 00057 return EOF; 00058 wait_ms( 1 ); 00059 } 00060 00061 m_timer.stop(); 00062 00063 int nbp = (m_contentStart + 1) % m_buffSize; 00064 uint8_t result = m_buff[m_contentStart]; 00065 m_contentStart = nbp; 00066 return result; 00067 } 00068 00069 //int Serial::_getc() { 00070 // return _base_getc(); 00071 //} 00072 00073 int SerialBuffered::_putc(int c) { 00074 return _base_putc(c); 00075 } 00076 00077 int SerialBuffered::readable() 00078 { 00079 return m_contentStart != m_contentEnd ; 00080 } 00081 00082 void SerialBuffered::handleInterrupt() 00083 { 00084 while (serial_readable(&_serial)) { 00085 char c_in = _base_getc(); 00086 int nbp = (m_contentEnd + 1) % m_buffSize; 00087 00088 if (nbp != m_contentStart) { 00089 m_buff[m_contentEnd] = c_in; 00090 m_contentEnd = nbp; 00091 } 00092 } 00093 }
Generated on Tue Jul 12 2022 19:39:23 by
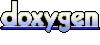