
Doxygen test
Embed:
(wiki syntax)
Show/hide line numbers
Gopher.cpp
00001 00002 #include "Gopher.h" 00003 #include "Game_engine.h" 00004 00005 // 2D array holding the information for rows and columns for game sprite. 1 shows a black block on screen, 0 no block. 00006 int game_gopher_sprite[7][10] = { 00007 {0,1,1,0,1,1,0,1,1,0}, 00008 {0,1,0,1,0,0,1,0,1,0}, 00009 {0,0,1,0,0,0,0,1,0,0}, 00010 {0,0,1,1,0,0,1,1,0,0}, 00011 {0,0,0,1,0,0,1,0,0,0}, 00012 {0,0,1,0,1,1,0,1,0,0}, 00013 {0,0,1,0,0,0,0,1,0,0}, 00014 }; 00015 00016 // 2D array to hold the positions of the 8 gophers on screen (x,y) 00017 int game_gopher_positions[8][2]= { 00018 {11,6}, 00019 {11,26}, 00020 {28,16}, 00021 {28,36}, 00022 {46,6}, 00023 {46,26}, 00024 {64,16}, 00025 {64,36}, 00026 }; 00027 00028 // Constructor for the object 00029 Gopher::Gopher() 00030 { 00031 00032 } 00033 00034 // Destructor for the object 00035 Gopher::~Gopher() 00036 { 00037 00038 } 00039 00040 00041 // Gets the gopher's current position 00042 Position2D Gopher::get_gopher_position() { 00043 00044 return pos; 00045 } 00046 00047 // Set gopher's random position (one of eight position options) 00048 void Gopher::set_random_position() { 00049 // variable to store the random number generated 00050 int g=0; 00051 00052 // Generates a random number (then divides by 8 to give 00053 // the remainder from 0 to 7 as there are 8 holes for the gopher) 00054 g=rand()%8; 00055 00056 // Using the random number g to select one of the gopher positions in the array (0 to 7) 00057 // Puts the position from the array into struct pos 00058 pos.x = game_gopher_positions[g][0]; 00059 pos.y = game_gopher_positions[g][1]; 00060 } 00061 00062 // Draws the gopher in one of eight set holes 00063 void Gopher::draw_game_gopher(N5110 &lcd) { 00064 // this draws the sprite at the x,y origin - how many rows and columns and name of sprite 00065 lcd.drawSprite(pos.x,pos.y,7,10,(int *)game_gopher_sprite); 00066 } 00067 00068 00069 00070 // function to draw 8 set position gopher holes 00071 void Gopher::draw_gopher_holes(N5110 &lcd) { 00072 00073 int i; 00074 for (i=0; i<8; i++) { 00075 00076 // this draws a line (start x(in relation to the gopher sprite size), start y(in relation to the gopher sprite size), 00077 //end x(in relation to the gopher sprite size), end y(in relation to the gopher sprite size), type) 00078 lcd.drawLine(game_gopher_positions[i][0],game_gopher_positions[i][1]+GOPHER_HEIGHT,game_gopher_positions[i][0]+(GOPHER_WIDTH-1),game_gopher_positions[i][1]+GOPHER_HEIGHT,1); 00079 } 00080 } 00081
Generated on Fri Jul 29 2022 10:01:21 by
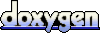