This a Library that can be used to make ping pong the Nokia Lcd 5110.
Embed:
(wiki syntax)
Show/hide line numbers
Ball.cpp
Go to the documentation of this file.
00001 00002 /** 00003 ** 00004 @file Ball.cpp 00005 @brief File containing all the functions prototypes , void etc for the ball. 00006 @brief Shows examples of creating Doxygen documentation. 00007 @brief Revision 1.0. 00008 @author Jefferson Sanchez 00009 @date April 2016 00010 */ 00011 00012 00013 #include "Ball.h" 00014 // @paramFunction to set the x value 00015 void ball::setX(int value) 00016 { 00017 x = value; 00018 } 00019 // Function to set the y value 00020 void ball::setY(int value) 00021 { 00022 y = value; 00023 } 00024 // Function to look for or check for the x value to be later used on the code . 00025 int ball::lookforX() 00026 { 00027 return x; 00028 } 00029 // Function to look for or check for the y value to be later used on the code 00030 00031 int ball::lookforY () 00032 { 00033 return y; 00034 } 00035 // Function to look for or check for the x value to ve later used on the code 00036 00037 int ball::lookforPos_stateX () 00038 { 00039 return x_Pos_state; 00040 } 00041 00042 int ball::lookforPos_stateY() 00043 { 00044 return y_Pos_state; 00045 } 00046 00047 void ball::BallcollisionState(int xPos_state, int yPos_state) 00048 { 00049 x_Pos_state = xPos_state; 00050 y_Pos_state = yPos_state; 00051 } 00052 00053 void ball::dbal(N5110 &display) 00054 { 00055 // this states the diameter of the ball 00056 for(int i = -3; i < 3; i++) 00057 for(int j = -3; j < 3; j++) 00058 display.setPixel(x + j, y + i); 00059 00060 display.refresh(); 00061 } 00062 00063 void ball::clear_dbal(N5110 &display) 00064 { 00065 //states the clearing of the ball as it moves 00066 for(int i = -3; i < 3; i++) 00067 for(int j = -3; j < 3; j++) 00068 display.clearPixel(x + j, y + i); 00069 00070 display.refresh(); 00071 } 00072 00073 void ball::Refresh_pos() 00074 { 00075 x += x_Pos_state; 00076 y += y_Pos_state; 00077 } 00078 // end of code
Generated on Thu Jul 21 2022 10:50:37 by
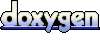