
Racing Cars game using N5110 LCD and thumb Joystick
Dependencies: N5110 PowerControl beep mbed
main.cpp
00001 /** 00002 @file main.cpp 00003 @brief Contains all functions implementations, and main program 00004 */ 00005 #include "main.h" 00006 00007 int main() 00008 00009 { 00010 00011 Peripheral_PowerDown(0xFFFFFFFF); //turn off all peripherals 00012 /* turn on only the required peripherals */ 00013 Peripheral_PowerUp(0x800000); 00014 Peripheral_PowerUp(0x200000); 00015 Peripheral_PowerUp(0x40); 00016 Peripheral_PowerUp(0x8000); 00017 Peripheral_PowerUp(0x1000); 00018 00019 sprintf(soundString ,"%s","YES"); //set the Sound Initial String to YES 00020 00021 calibrateJoystick(); // get centred values of joystick 00022 pollJoystick.attach(&updateJoystick,1.0/20.0); // read joystick 30 times per second 00023 00024 lcd.setXYAddress(0,0);//set the XY address to 0.0 as initialising condition 00025 initTable();//initialise the array table 00026 lcd.init();//initialise LCD screen 00027 lcd.refresh(); 00028 /* PRINT WELCOMING MESSAGES */ 00029 lcd.printString("RACING CARS",9,1); 00030 wait(0.5); 00031 lcd.printString("GAME",29,2); 00032 wait(0.5); 00033 lcd.printString("by Giorgos",14,4); 00034 wait(0.5); 00035 lcd.printString("Savvides",16,5); 00036 wait(2); 00037 lcd.clear(); 00038 lcd.refresh(); 00039 lcd.printString("USE JOYSTICK",0,0); 00040 lcd.printString("AND BUTTONS ",0,1); 00041 lcd.printString("ON THE RIGHT",0,2); 00042 lcd.printString("TO NAVIGATE",0,3); 00043 lcd.printString("HAVE FUN!!!",12,5); 00044 wait(3.5); 00045 start.rise(&startButtonPressed); // Call function startButtonPressed when START button pressed 00046 reset.rise(&resetButtonPressed); // Call function resetButtonPressed when RESET button pressed 00047 00048 MainMenu: 00049 /* INITIAL DISPLAY IN MAIN MENU */ 00050 00051 int menuPointer=1; // set the Pointer in Main Menu initially to 1 00052 startButtonFlag=0; // reset the pointing flags 00053 resetButtonFlag=0; // reset the pointing flags 00054 clearCells(84,48); // clear the whole Screen Cells 00055 lcd.refresh(); // refresh are done after and before setting or clearing Pixels 00056 lcd.drawCircle(22,19,2,1); //initial condition draw pointer around Play submenu 00057 lcd.drawCircle(53,19,2,1); //initial condition draw pointer around Play submenu 00058 00059 lcd.printString("MAIN MENU",15,0); //print title 00060 lcd.printString("Play",26,2); //print submenu title 00061 lcd.printString("Options",20,3); //print submenu title 00062 lcd.drawLine(15,9,65,9,1);//underline main menu title 00063 wait(0.1); 00064 /* While start Button is not pressed user can navigate through main Menu Submenus */ 00065 while(startButtonFlag==0) { 00066 00067 /* when joystick moves down, pointer selects Options subMenu */ 00068 if (joystick .direction==DOWN) { 00069 lcd.drawCircle(16,27,2,1); //draw pointer around Options submenu when selected 00070 lcd.drawCircle(64,27,2,1); //draw pointer around Options submenu when selected 00071 00072 lcd.clearCircle(53,19,2,1); //clear pointer around Play submenu 00073 lcd.clearCircle(22,19,2,1); //clear pointer around Play submenu 00074 00075 menuPointer=2; //set Pointer to 2 00076 } 00077 00078 /* when joystick moves up, pointer selects Options subMenu */ 00079 if(joystick .direction==UP) { 00080 lcd.drawCircle(22,19,2,1); //draw pointer around Play submenu when selected 00081 lcd.drawCircle(53,19,2,1); //draw pointer around Play submenu when selected 00082 00083 lcd.clearCircle(16,27,2,1); //clear pointer around Options submenu 00084 lcd.clearCircle(64,27,2,1); //clear pointer around Options submenu 00085 menuPointer=1; //set Pointer to 1 00086 } 00087 sleep(); 00088 } 00089 00090 /* SELECT POINTER CONDITION */ 00091 switch(menuPointer) { 00092 00093 /* Case when Play was selected */ 00094 case 1: 00095 /*INITIAL GAME CONDITIONS */ 00096 startButtonFlag=0; //reset start Button Flag 00097 gamePlays =1; // Begin the game with pointer set to 1 00098 lives =5; // set lives to 5 00099 round =1; // set round number to 1 00100 coins =0; // set coin number to 0 00101 clearCells(84,48); //clear the whole LCD screen 00102 00103 lcd.refresh(); 00104 lcd.printString("READY?",20,3); 00105 wait(0.75); 00106 lcd.printString("GET SET",20,3); 00107 wait(1); 00108 lcd.printString(" GO !!!",20,3); 00109 wait(1); 00110 lcd.clear(); 00111 lcd.refresh(); 00112 00113 00114 gameReset(); // set and draw initial player and enemies position 00115 timer.attach(&movePlayer,0.1);// attach timer to call function that moves the Player 00116 00117 00118 while(gamePlays ) { 00119 00120 //print Round number on buffer and display 00121 sprintf(roundBuffer ,"%d",round ); 00122 lcd.printString(roundBuffer ,74,3); 00123 lcd.refresh(); 00124 00125 //print Lives number on buffer and display 00126 sprintf(livesBuffer ,"%d",lives ); 00127 lcd.printString(livesBuffer ,74,1); 00128 lcd.refresh(); 00129 00130 //print Coins number on buffer and display 00131 sprintf(coinsBuffer ,"%d",coins ); 00132 lcd.printString(coinsBuffer ,72,5); 00133 00134 lcd.refresh(); 00135 enemy1MovesDown(); //move enemy 1 downwards 00136 00137 checkPlayerPos(); //check player position frequently 00138 if (gamePlays ==0) { // if gameplay is 0 returns to initial MAIN MENU 00139 goto MainMenu; 00140 } 00141 00142 lcd.refresh(); 00143 enemy2MovesDown(); //move enemy 2 downwards 00144 00145 checkPlayerPos(); 00146 if (gamePlays ==0) { 00147 goto MainMenu; 00148 } 00149 00150 lcd.refresh(); 00151 enemy3MovesDown(); //move enemy 3 downwards 00152 00153 checkPlayerPos(); 00154 if (gamePlays ==0) { 00155 goto MainMenu; 00156 } 00157 coinMoves(); // move coin downwards 00158 lcd.refresh(); 00159 checkPlayerPos(); 00160 if (gamePlays ==0) { 00161 goto MainMenu; 00162 } 00163 } 00164 00165 break; 00166 00167 case 2: 00168 00169 int optionsMenu=1; // set options Menu enabled 00170 optionsPointer =1; 00171 startButtonFlag=0; //reset START Button Flag 00172 00173 clearCells(84,48); // clear all screen Cells 00174 lcd.refresh(); 00175 wait(0.02); 00176 /* PRINT OPTIONS SUBMENU DISPLAY */ 00177 lcd.printString("OPTIONS",18,0); 00178 lcd.printString("Exit",23,5); 00179 lcd.printString("Brightness",6,3); 00180 lcd.printString("Sound",6,2); 00181 lcd.drawLine(15,8,61,8,1); //underline Options Menu title 00182 00183 /* Print brightness value ranged from 1 to 10 to user */ 00184 sprintf(brightnessBuffer ,"%d",brightnessDisplay ); 00185 lcd.printString(brightnessBuffer ,68,3); 00186 00187 /* Print Sound string value , "YES" or "NO"*/ 00188 lcd.printString(soundString ,40,2); 00189 00190 /* USER NAVIGATES WHILE IN OPTIONS MENU */ 00191 while(optionsMenu) { 00192 00193 /* If direction is up pointer value decrease by 1 (cannot decrease to less than 1) */ 00194 if (joystick .direction==UP) { 00195 optionsPointer --; 00196 if(optionsPointer ==0) { 00197 optionsPointer =1; 00198 } 00199 } 00200 wait(0.05); 00201 00202 /* If direction is down, pointer value increase by 1 (cannot increase to more than 3) */ 00203 if(joystick .direction==DOWN) { 00204 optionsPointer ++; 00205 if(optionsPointer >3) { 00206 optionsPointer =3; 00207 } 00208 } 00209 /* CASES FOR EACH POINTER VALUE */ 00210 /* when it is 1, Sound submenu is selected */ 00211 if (optionsPointer ==1) { 00212 /* print Circle pointer around Sound submenu */ 00213 lcd.drawCircle(2,19,2,1); 00214 lcd.clearCircle(2,27,2,1); 00215 lcd.clearCircle(19,44,2,1); 00216 /* if pointer is 1 and joystick direction is left, sound is enabled (=1)*/ 00217 if(joystick .direction==LEFT) { 00218 sounds =1; 00219 lcd.refresh(); 00220 sprintf(soundString ,"%s","YES"); 00221 lcd.printString(soundString ,40,2); 00222 } 00223 /* if pointer is 1 and joystick direction is right, sound is disabled (=0)*/ 00224 if(joystick .direction==RIGHT) { 00225 sounds =0; 00226 lcd.refresh(); 00227 sprintf(soundString ,"%s","NO "); //print string in buffer 00228 lcd.printString(soundString ,40,2); //display value of buffer 00229 } 00230 sleep(); 00231 } 00232 /* when it is 2, brightness submenu is selected */ 00233 if (optionsPointer ==2) { 00234 /* print Circle pointer around Brightness submenu */ 00235 lcd.drawCircle(2,27,2,1); 00236 lcd.clearCircle(2,19,2,1); 00237 lcd.clearCircle(19,44,2,1); 00238 00239 /* if pointer is 2 and joystick direction is left, brightness decreases by 0.1*/ 00240 if(joystick .direction==LEFT) { 00241 brightness =brightness -.1; 00242 brightnessDisplay =brightnessDisplay -1; // user displayed value also decrease 00243 if(brightness <0.1||brightnessDisplay <0) { // brightnessDisplay and brightness never go below 0 and 0.1 respectively 00244 brightnessDisplay =1; 00245 brightness =0.1; 00246 } 00247 lcd.setBrightness(brightness ); //sets the brightness 00248 00249 /* print brightness Display on screen */ 00250 sprintf(brightnessBuffer ,"%d",brightnessDisplay ); 00251 lcd.printString(brightnessBuffer ,68,3); 00252 } 00253 00254 /* if pointer is 2 and joystick direction is right, brightness increases by 0.1*/ 00255 if(joystick .direction==RIGHT) { 00256 brightness =brightness +.1; 00257 brightnessDisplay =brightnessDisplay +1; // user displayed value also increase 00258 if(brightness >0.9||brightnessDisplay >9) {// brightnessDisplay and brightness never go above 9 and 0.9 respectively 00259 brightnessDisplay =9; 00260 brightness =0.9; 00261 } 00262 lcd.setBrightness(brightness ); //sets the brightness 00263 00264 /* print brightness Display on screen */ 00265 sprintf(brightnessBuffer ,"%d",brightnessDisplay ); 00266 lcd.printString(brightnessBuffer ,68,3); 00267 } 00268 sleep(); 00269 } 00270 if (optionsPointer ==3) { 00271 /* print Circle pointer around Exit submenu */ 00272 lcd.drawCircle(19,44,2,1); 00273 lcd.clearCircle(2,19,2,1); 00274 lcd.clearCircle(2,27,2,1); 00275 00276 /* if START button is press while pointer is on Exit goes back to Main Menu label */ 00277 if(startButtonFlag) { 00278 startButtonFlag=0; 00279 wait(0.5); 00280 optionsMenu=0; 00281 goto MainMenu; 00282 } 00283 sleep(); 00284 } 00285 wait(0.05); 00286 sleep(); 00287 00288 } 00289 break; 00290 } 00291 } 00292 00293 void startButtonPressed() 00294 { 00295 startButtonFlag=!startButtonFlag; 00296 } 00297 00298 void resetButtonPressed() 00299 { 00300 resetButtonFlag=!resetButtonFlag; 00301 } 00302 00303 void movePlayer() 00304 { 00305 if (gamePlays ) { 00306 /* if direction is RIGHT clears the previous Car shape and draws the next one by increasing the x position */ 00307 if (joystick .direction==RIGHT&&x +w <58) { 00308 00309 clearRect(x ,v ,w ,h ); 00310 lcd.refresh(); 00311 x =x +4; 00312 lcd.drawRect(x ,v ,w ,h ,0); 00313 lcd.refresh(); 00314 } 00315 /* if direction is LEFT clears the previous Car shape and draws the next one by decreasing the x position */ 00316 if (joystick .direction==LEFT&&x +w >10) { 00317 00318 clearRect(x ,v ,w ,h ); 00319 lcd.refresh(); 00320 x =x -4; 00321 00322 lcd.drawRect(x ,v ,w ,h ,0); 00323 lcd.refresh(); 00324 } 00325 /* if direction is UP clears the previous Car shape and draws the next one by decreasing the y position */ 00326 if (joystick .direction==UP && v >2) { 00327 00328 clearRect(x ,v ,w ,h ); 00329 lcd.refresh(); 00330 v =v -4; 00331 lcd.drawRect(x ,v ,w ,h ,0); 00332 lcd.refresh(); 00333 } 00334 /* if direction is DOWN clears the previous Car shape and draws the next one by increasing the y position */ 00335 if (joystick .direction==DOWN && v <32) { 00336 clearRect(x ,v ,w ,h ); 00337 lcd.refresh(); 00338 v =v +4; 00339 lcd.drawRect(x ,v ,w ,h ,0); 00340 lcd.refresh(); 00341 } 00342 00343 } 00344 } 00345 00346 void calibrateJoystick() 00347 { 00348 button.mode(PullDown); 00349 // must not move during calibration 00350 joystick .x0 = xPot; // initial positions in the range 0.0 to 1.0 (0.5 if centred exactly) 00351 joystick .y0 = yPot; 00352 } 00353 void updateJoystick() 00354 { 00355 joystick .x = xPot - joystick .x0; 00356 joystick .y = yPot - joystick .y0; 00357 // read button state 00358 joystick .button = button; 00359 00360 // calculate direction depending on x,y values 00361 // tolerance allows a little lee-way in case joystick not exactly in the stated direction 00362 if ( fabs(joystick .y) < DIRECTION_TOLERANCE && fabs(joystick .x) < DIRECTION_TOLERANCE) { 00363 joystick .direction = CENTRE; 00364 } else if ( joystick .y > DIRECTION_TOLERANCE && fabs(joystick .x) < DIRECTION_TOLERANCE) { 00365 joystick .direction = UP; 00366 } else if ( joystick .y < DIRECTION_TOLERANCE && fabs(joystick .x) < DIRECTION_TOLERANCE) { 00367 joystick .direction = DOWN; 00368 } else if ( joystick .x > DIRECTION_TOLERANCE && fabs(joystick .y) < DIRECTION_TOLERANCE) { 00369 joystick .direction = LEFT; 00370 } else if ( joystick .x < DIRECTION_TOLERANCE && fabs(joystick .y) < DIRECTION_TOLERANCE) { 00371 joystick .direction = RIGHT; 00372 } else { 00373 joystick .direction = UNKNOWN; 00374 } 00375 00376 // set flag for printing 00377 printFlag = 1; 00378 } 00379 00380 00381 void clearRect(int x ,int v ,int w ,int h ) 00382 { 00383 // loop through the array cells, clear the Pixels and array cells value(=0) 00384 for(int i=x; i<=x+w ; i++) { 00385 for(int j =v; j <=v+h ; j ++) { 00386 lcd.clearPixel(i,j ); 00387 table [i][j ]=0; 00388 } 00389 } 00390 } 00391 00392 00393 void enemy1MovesDown() 00394 { 00395 clearRect(enemy1x ,(j -a ),w ,h ); // clears the previous Enemy Car 00396 lcd.refresh(); 00397 lcd.drawRect(enemy1x ,j ,w ,h ,1); //draws the next enemy Car 00398 lcd.refresh(); 00399 setRectCells(enemy1x ,j ,w ,h ); //set the current enemy cells to 1 00400 lcd.refresh(); 00401 wait(0.05); 00402 00403 j =j +a ; //increase the y-position counter 00404 00405 if(j >70) { // after reaching certain y position, randomize it again br 00406 j =-(rand()%95+85); 00407 } 00408 00409 } 00410 00411 void enemy2MovesDown() 00412 { 00413 clearRect(enemy2x ,(p -a ),w ,h ); // clears the previous Enemy Car 00414 lcd.refresh(); 00415 lcd.drawRect(enemy2x ,p ,w ,h ,1); //draws the next enemy Car 00416 lcd.refresh(); 00417 setRectCells(enemy2x ,p ,w ,h ); //set the current enemy cells to 1 00418 lcd.refresh(); 00419 wait(0.05); 00420 p =p +a ; //increase the y-position counter 00421 00422 if(p >70) { 00423 p =-(rand()%60+40); 00424 } 00425 00426 } 00427 00428 void enemy3MovesDown() 00429 { 00430 clearRect(enemy3x ,(q -a ),w ,h ); 00431 lcd.refresh(); 00432 lcd.drawRect(enemy3x ,q ,w ,h ,1); 00433 lcd.refresh(); 00434 setRectCells(enemy3x ,q ,w ,h ); 00435 lcd.refresh(); 00436 wait(0.05); 00437 lcd.refresh(); 00438 q =q +a ; 00439 if (q >70) { 00440 q =-(rand()%34+3); 00441 } 00442 00443 } 00444 00445 void setRectCells(int x ,int v ,int w ,int h ) 00446 { 00447 // Sets the Rectangle Array Cells equal to 1, indicating there is an enemy 00448 for(int i=x; i<=x+w ; i++) { 00449 for(int j =v; j <=v+h ; j ++) { 00450 if(j >0&&j <48) { 00451 table [i][j ]=1; 00452 } 00453 } 00454 } 00455 } 00456 00457 void initTable() 00458 { 00459 // initialise the Array's cells, set them equal to 0 00460 for (int i=0; i<=83; i++) { 00461 for(int j =0; j <=47; j ++) { 00462 table [i][j ]=0; 00463 } 00464 } 00465 } 00466 00467 void checkPlayerPos() 00468 { 00469 // frequently calling this function to check if player touched an enemy or a coin 00470 /* if player cells equal to 1, means he touched enemy, so loose function is being called */ 00471 if (table [x +w ][v +h ]==1||table [x ][v +h ]==1||table [x ][v ]==1||table [x +w ][v ]==1) { 00472 loose(); 00473 } 00474 /* if player cells equal to 2, means he touched a coin */ 00475 if (table [x +w ][v +h ]==2||table [x ][v +h ]==2||table [x ][v ]==2||table [x +w ][v ]==2) { 00476 coins =coins +5; //each coin's value is 5,total coins increase by 5 00477 clearCoin(c ); //clear the collected Coin 00478 lcd.drawRect(x ,v ,w ,h ,0);//redraw Player boundaries that were cleared by Coin 00479 if(sounds ) { // if sound is enabled in options menu sound produced when got a coin 00480 buzzer.beep(2200,0.4); //produce sound of frequency 2200 Hz and duration 0.4 s 00481 buzzer.beep(1000,0.2); 00482 lcd.setBrightness(brightness ); //set brightness again, frequency produced interfere with LCD pin 00483 } 00484 coinAppear =0; //switch coin status to disappear 00485 00486 /* then Check the total number of coins, to produce to next Round */ 00487 00488 if(coins ==30) { // if coins are 40 proceed to next Round 00489 round =2; // change Round Value 00490 gamePlays =0; // stop gameplay, preventing user moving on screen 00491 clearCells(84,48); //clear all Cells 00492 lcd.refresh(); 00493 lcd.printString("ROUND 2",10,2); //print Round message 00494 wait(1); 00495 clearCells(84,48);// clear all Cells 00496 lcd.refresh(); 00497 gamePlays =1;//start gameplay 00498 gameReset();//reset player and enemies positions 00499 a =6; //change enemies acceleration (a is added to y positions counter) 00500 } 00501 if(coins ==70) { // if coins are 70 proceed to next Round 00502 round =3; // change Round Value 00503 gamePlays =0;// stop gameplay, preventing user moving on screen 00504 clearCells(84,48); //clear all Cells 00505 lcd.refresh(); 00506 lcd.printString("ROUND 3",10,2); //print Round message 00507 wait(1); 00508 clearCells(84,48); // clear all Cells 00509 lcd.refresh(); 00510 gamePlays =1; //start gameplay 00511 gameReset(); //reset player and enemies positions 00512 a =8; //change enemies acceleration (a is added to y positions counter) 00513 } 00514 if(coins ==90) { // if coins are 90, player WINS the game 00515 timer.detach(); //stop player moving 00516 clearCells(84,48); //clear pixels 00517 lcd.clear(); //clear LCD 00518 lcd.refresh(); 00519 lcd.printString("CONGRATS!",0,2); //print win message 00520 lcd.printString("YOU WON!",8,3); //print win message 00521 wait(4); 00522 gamePlays =0; //game finishes 00523 } 00524 } 00525 } 00526 00527 void coinMoves() 00528 { 00529 if(table [xPos ][c ]==1||table [xPos ][c +4]==1||table [xPos +4][c +4]==1) { //prevent coin from appearing above enemies 00530 clearCoin(c ); //clear current Coin 00531 coinAppear =0; //make coin disappear (prevent from being redrawn) 00532 } 00533 clearCoin(c ); //clear current Coin 00534 c =c +4; // increase Coin's y coordinate counter 00535 drawCoin(c ); //draw next Coin position 00536 if(c >80) { // if counter exceeds 80, randomizes x and y positions and make it reappear 00537 c =-(rand()%10); 00538 xPos =rand()%52+4; 00539 coinAppear =1; 00540 } 00541 } 00542 00543 void drawCoin(int c ) 00544 { 00545 if (c>4&&c<48&&coinAppear ==1) { //check if coin c point(y pos) is within LCD limits 00546 lcd.drawCircle(xPos ,c,4,0); //Draw Coin Circle Pixels 00547 setCircleCells(xPos ,c,4);// Set Coin array's cells values to 2 00548 00549 // "c" character Print on coin 00550 lcd.setPixel(xPos ,c-1); 00551 lcd.setPixel(xPos ,c+2); 00552 lcd.setPixel(xPos +1,c-1); 00553 lcd.setPixel(xPos +1,c+2); 00554 lcd.setPixel(xPos -1,c); 00555 lcd.setPixel(xPos -1,c+1); 00556 00557 lcd.refresh(); 00558 } 00559 } 00560 00561 void clearCoin(int c ) 00562 { 00563 if(c>4&&c<48&&coinAppear ==1) { // if c point of the Coin within the LCD limits 00564 lcd.clearCircle(xPos ,c,4,1); //Clear the Coin 00565 clearCircleCells(xPos ,c,4);// Set Coin array's cells values to 0 00566 lcd.refresh(); 00567 } 00568 } 00569 00570 void loose() 00571 { 00572 00573 if (sounds ) { //check if sound is enabled and produce a "loose" sound of frequency 400 Hz 00574 buzzer.beep(400,0.2); 00575 lcd.setBrightness(brightness ); //set brightness 00576 } 00577 timer.detach(); //detach moving Player function timer, so player cannot move 00578 lives =lives -1; //decrease lives value 00579 startButtonFlag=0; //reset START button flag 00580 resetButtonFlag=0;//reset SELECT button flag 00581 if(lives ==0) { //if lives are equal to 0, Game Over is displayed 00582 00583 clearCells(84,48); 00584 lcd.refresh(); 00585 lcd.clear(); 00586 00587 wait(1); 00588 while(startButtonFlag==0&&resetButtonFlag==0) { //while no button is pressed 00589 lcd.printString("Game Over!!!",0,0); 00590 lcd.printString("Press START",0,2); 00591 lcd.printString("to try again",0,3); 00592 lcd.printString("or RESET",0,4); 00593 lcd.printString("to exit :)",0,5); 00594 } 00595 if(startButtonFlag) { //START button pressed, resets the game 00596 a =4; 00597 gamePlays =1; 00598 lives =5; 00599 coins =0; 00600 round =1; 00601 clearCells(84,48); 00602 gameReset(); 00603 startButtonFlag=0; 00604 wait(1); 00605 timer.attach(&movePlayer,0.1); 00606 } 00607 if (resetButtonFlag) { //RESET button pressed, sets gameplay to 0 ( when gameplay is 0 , returns to MAIN MENU) 00608 gamePlays =0; 00609 resetButtonFlag=0; 00610 } 00611 } 00612 00613 else { 00614 clearCells(60,48); //else if lives are not 0, clearCells and reset the game 00615 lcd.refresh(); 00616 gameReset(); // reset player and enemies values 00617 wait(2); 00618 gamePlays =1;// game is being played 00619 timer.attach(&movePlayer,0.1); //attach move Player function 00620 } 00621 } 00622 00623 void gameReset() 00624 { 00625 initTable(); // initialise the array (set cells values to 0) 00626 x =26; //initial player x position 00627 v =30; //initial player y position 00628 lcd.drawRect(x ,v ,w ,h ,0); //draw player at initial position 00629 lcd.refresh(); 00630 j =-70; // enemy y position, initialise 00631 q =-10; // enemy y position, initialise 00632 p =-40; // enemy y position, initialise 00633 00634 } 00635 00636 00637 void setCircleCells(int x0,int y0,int radius) 00638 { 00639 //function from N5110 Library, works only for filled circle 00640 int x = radius; 00641 int y = 0; 00642 00643 while(x >= y) { 00644 00645 setLineCells(x+x0,y+y0,-x+x0,y+y0,0); //set line's Cells values to 2 00646 setLineCells(y+x0,x+y0,-y+x0,x+y0,0);//set line's Cells values to 2 00647 setLineCells(y+x0,-x+y0,-y+x0,-x+y0,0);//set line's Cells values to 2 00648 setLineCells(x+x0,-y+y0,-x+x0,-y+y0,0);//set line's Cells values to 2 00649 00650 y++; 00651 } 00652 } 00653 00654 void clearCircleCells(int x0,int y0,int radius) 00655 { 00656 //function from N5110 Library, works only for filled circle 00657 int x = radius; 00658 int y = 0; 00659 00660 while(x >= y) { 00661 clearLineCells(x+x0,y+y0,-x+x0,y+y0,0);//set line's Cells values to 0 00662 clearLineCells(y+x0,x+y0,-y+x0,x+y0,0);//set line's Cells values to 0 00663 clearLineCells(y+x0,-x+y0,-y+x0,-x+y0,0);//set line's Cells values to 0 00664 clearLineCells(x+x0,-y+y0,-x+x0,-y+y0,0);//set line's Cells values to 0 00665 y++; 00666 } 00667 } 00668 00669 void clearCells(int x ,int y) 00670 { 00671 // loops through cells, clears the pixels and set the cells value equal to 0 00672 for(int i=0; i<=x ; i++) { 00673 for (int j =0; j <=y; j ++) { 00674 lcd.clearPixel(i,j ); 00675 table [i][j ]=0; 00676 } 00677 } 00678 lcd.refresh(); 00679 } 00680 00681 00682 void setLineCells(int x0,int y0,int x1,int y1,int type) 00683 { 00684 //function from N5110 Library 00685 int y_range = y1-y0; // calc range of y and x 00686 int x_range = x1-x0; 00687 int start,stop,step; 00688 00689 // if dotted line, set step to 2, else step is 1 00690 step = (type==2) ? 2:1; 00691 00692 // make sure we loop over the largest range to get the most pixels on the display 00693 // for instance, if drawing a vertical line (x_range = 0), we need to loop down the y pixels 00694 // or else we'll only end up with 1 pixel in the x column 00695 if ( abs(x_range) > abs(y_range) ) { 00696 00697 // ensure we loop from smallest to largest or else for-loop won't run as expected 00698 start = x1>x0 ? x0:x1; 00699 stop = x1>x0 ? x1:x0; 00700 00701 // loop between x pixels 00702 for (int x = start; x <= stop ; x +=step) { 00703 // do linear interpolation 00704 int y = y0 + (y1-y0)*(x -x0)/(x1-x0); 00705 table [x ][y]=2; // set table values to 2 00706 } 00707 } else { 00708 00709 // ensure we loop from smallest to largest or else for-loop won't run as expected 00710 start = y1>y0 ? y0:y1; 00711 stop = y1>y0 ? y1:y0; 00712 00713 for (int y = start; y<= stop ; y+=step) { 00714 // do linear interpolation 00715 int x = x0 + (x1-x0)*(y-y0)/(y1-y0); 00716 table [x ][y]=2; // set table values to 2 00717 } 00718 } 00719 00720 } 00721 00722 void clearLineCells(int x0,int y0,int x1,int y1,int type) 00723 { 00724 //function from N5110 Library 00725 int y_range = y1-y0; // calc range of y and x 00726 int x_range = x1-x0; 00727 int start,stop,step; 00728 00729 // if dotted line, set step to 2, else step is 1 00730 step = (type==2) ? 2:1; 00731 00732 // make sure we loop over the largest range to get the most pixels on the display 00733 // for instance, if drawing a vertical line (x_range = 0), we need to loop down the y pixels 00734 // or else we'll only end up with 1 pixel in the x column 00735 if ( abs(x_range) > abs(y_range) ) { 00736 00737 // ensure we loop from smallest to largest or else for-loop won't run as expected 00738 start = x1>x0 ? x0:x1; 00739 stop = x1>x0 ? x1:x0; 00740 // loop between x pixels 00741 for (int x = start; x <= stop ; x +=step) { 00742 // do linear interpolation 00743 int y = y0 + (y1-y0)*(x -x0)/(x1-x0); 00744 table [x ][y]=0; // set Table Cell to 0 00745 } 00746 } else { 00747 // ensure we loop from smallest to largest or else for-loop won't run as expected 00748 start = y1>y0 ? y0:y1; 00749 stop = y1>y0 ? y1:y0; 00750 00751 for (int y = start; y<= stop ; y+=step) { 00752 // do linear interpolation 00753 int x = x0 + (x1-x0)*(y-y0)/(y1-y0); 00754 table [x ][y]=0; // set Table Cell to 0 00755 } 00756 } 00757 } 00758
Generated on Wed Jul 13 2022 05:14:51 by
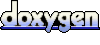