
revised version of F746_SD_GraphicEqualizer
Dependencies: BSP_DISCO_F746NG F746_GUI F746_SAI_IO FrequencyResponseDrawer LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed
Fork of F746_SD_GraphicEqualizer by
SD_WavReader.hpp
00001 //-------------------------------------------------------------- 00002 // SD_WavReader class ---- Header 00003 // SD カードの *.wav ファイルの内容を読み出す 00004 // 以下のフォーマット以外は扱わない 00005 // PCM,16 ビットステレオ,標本化周波数 44.1 kHz 00006 // 00007 // 2016/04/19, Copyright (c) 2016 MIKAMI, Naoki 00008 //-------------------------------------------------------------- 00009 00010 #ifndef SD_WAV_READER_HPP 00011 #define SD_WAV_READER_HPP 00012 00013 #include "SDFileSystem.h" 00014 #include "BlinkLabel.hpp" 00015 #include <string> 00016 00017 namespace Mikami 00018 { 00019 class SD_WavReader 00020 { 00021 public: 00022 SD_WavReader(int32_t bufferSize); 00023 ~SD_WavReader(); 00024 00025 void Open(const string fileName); 00026 00027 void Close() { fclose(fp_); } 00028 00029 // ファイルのヘッダ (RIFFxxxxWAVEfm ) 読み込み 00030 // 戻り値: *.wav で,16 ビットステレオ, 00031 // 標本化周波数:44.1 kHz の場合 true 00032 bool IsWavFile(); 00033 00034 // ファイルからデータの取得 00035 void Read(int16_t data[], uint32_t size); 00036 00037 // ファイルからデータをモノラルに変換しての取得 00038 void ReadAndToMono(int16_t data[], uint32_t size); 00039 00040 // データサイズ(標本化点の数)の取得 00041 int32_t GetSize(); 00042 00043 // ren: show a string 00044 /* void ren_Msg1(int pos, char msg[]) 00045 { int lin, str_ln, y_pos[7]= {0,70,130,160,190,220,250}; 00046 // char msg[501]; 00047 // strcpy(msg, str_msg.c_str()); 00048 // str_ln=str_msg.size(); 00049 // if(str_ln>500) str_ln=500; 00050 // msg[str_ln]='\0'; 00051 if(pos<0) lin=0; 00052 else if (pos>6) lin=6; 00053 else lin=pos; 00054 Label renLabel(0, y_pos[lin], msg, Label::LEFT,Font20); 00055 } 00056 */ 00057 private: 00058 const string STR_; 00059 00060 struct WaveFormatEx 00061 { 00062 uint16_t wFormatTag; // 1: PCM 00063 uint16_t nChannels; // 1:モノラル,2: ステレオ 00064 uint32_t nSamplesPerSec; // 標本化周波数 (Hz) 00065 uint32_t nAvgBytesPerSec; // 転送速度 (bytes/s) 00066 uint16_t nBlockAlign; // 4: 16ビットステレオの場合 00067 uint16_t wBitsPerSample; // データのビット数,8 または 16 00068 uint16_t cbSize; 00069 }; 00070 00071 SDFileSystem *sd_; 00072 FILE *fp_; 00073 00074 bool ok_; 00075 int32_t size_; // モノラルデータのサイズ 00076 int16_t *buffer; // ステレオをモノラルに変換する際の作業領域 00077 00078 void ErrorMsg(char msg[]) 00079 { BlinkLabel errLabel(240, 100, msg, Label::CENTER); } 00080 00081 // disallow copy constructor and assignment operator 00082 SD_WavReader(const SD_WavReader&); 00083 SD_WavReader& operator=(const SD_WavReader&); 00084 }; 00085 } 00086 #endif // SD_BINARY_READER_HPP
Generated on Wed Jul 13 2022 09:35:21 by
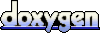