
revised version of F746_SD_GraphicEqualizer
Dependencies: BSP_DISCO_F746NG F746_GUI F746_SAI_IO FrequencyResponseDrawer LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed
Fork of F746_SD_GraphicEqualizer by
GrEqParamsCalculator.hpp
00001 //------------------------------------------------------------------------------ 00002 // Parameters calculator class of buquad unit for graphic equalizer 00003 // グラフィックイコライザで使う biquad フィルタの係数を計算するクラス 00004 // 00005 // 2016/05/09, Copyright (c) 2016 MIKAMI, Naoki 00006 //------------------------------------------------------------------------------ 00007 00008 #ifndef GRAPHIC_EQALIZER_PARAMETER_CALCULATOR_HPP 00009 #define GRAPHIC_EQALIZER_PARAMETER_CALCULATOR_HPP 00010 00011 #include "mbed.h" 00012 #include "BiquadGrEq.hpp" 00013 00014 namespace Mikami 00015 { 00016 class GrEqParams 00017 { 00018 public: 00019 // Constructor 00020 GrEqParams(int bands, float fs) : BANDS_(bands), FS_(fs) {} 00021 00022 // 係数を計算する 00023 BiquadGrEq::Coefs Execute(int band, float f0, float gDb, float qVal) 00024 { 00025 const float PI = 3.1415926536f; 00026 BiquadGrEq::Coefs coefs; 00027 00028 float gSqrt = sqrtf(powf(10, gDb/20.0f)); 00029 float w0 = 2.0f*PI*f0/FS_; 00030 00031 if ( (band != 0) && (band != BANDS_-1) ) 00032 { 00033 float alpha = sinf(w0)/(2.0f*qVal); 00034 float a0 = 1.0f + alpha/gSqrt; 00035 coefs.a1 = 2.0f*cosf(w0)/a0; 00036 coefs.a2 = -(1.0f - alpha/gSqrt)/a0; 00037 coefs.b0 = (1.0f + alpha*gSqrt)/a0; 00038 coefs.b1 = -coefs.a1; 00039 coefs.b2 = (1.0f - alpha*gSqrt)/a0; 00040 } 00041 else 00042 { 00043 w0 = (band == 0) ? w0*1.414f : w0/1.414f; 00044 float alpha = sinf(w0)/sqrtf(2.0f); // Q = 1/sqrt(2) 00045 float g_a = sqrtf(gSqrt); 00046 float cosW0 = cosf(w0); 00047 float gSqrtP1 = gSqrt+1.0f; 00048 float gSqrtM1 = gSqrt-1.0f; 00049 if (band == 0) 00050 { 00051 float a0 = gSqrtP1 + gSqrtM1*cosW0 00052 + 2.0f*g_a*alpha; 00053 coefs.a1 = 2.0f*(gSqrtM1 + gSqrtP1*cosW0)/a0; 00054 coefs.a2 = -(gSqrtP1 + gSqrtM1*cosW0 00055 - 2.0f*g_a*alpha)/a0; 00056 coefs.b0 = gSqrt*(gSqrtP1 - gSqrtM1*cosW0 00057 + 2.0f*g_a*alpha)/a0; 00058 coefs.b1 = 2.0f*gSqrt*(gSqrtM1 00059 - gSqrtP1*cosW0)/a0; 00060 coefs.b2 = gSqrt*(gSqrtP1 - gSqrtM1*cosW0 00061 - 2.0f*g_a*alpha)/a0; 00062 } 00063 else 00064 { 00065 float a0 = gSqrtP1 - gSqrtM1*cosW0 00066 + 2.0f*g_a*alpha; 00067 coefs.a1 = -2.0f*(gSqrtM1 - gSqrtP1*cosW0)/a0; 00068 coefs.a2 = -(gSqrtP1 - gSqrtM1*cosW0 00069 - 2.0f*g_a*alpha)/a0; 00070 coefs.b0 = gSqrt*(gSqrtP1 + gSqrtM1*cosW0 00071 + 2.0f*g_a*alpha)/a0; 00072 coefs.b1 = -2.0f*gSqrt*(gSqrtM1 + gSqrtP1*cosW0)/a0; 00073 coefs.b2 = gSqrt*(gSqrtP1 + gSqrtM1*cosW0 00074 - 2.0f*g_a*alpha)/a0; 00075 } 00076 } 00077 00078 return coefs; 00079 } 00080 00081 private: 00082 const int BANDS_; 00083 const float FS_; 00084 }; 00085 } 00086 #endif // GRAPHIC_EQALIZER_PARAMETER_CALCULATOR_HPP
Generated on Wed Jul 13 2022 09:35:21 by
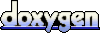