
revised version of F746_SD_GraphicEqualizer
Dependencies: BSP_DISCO_F746NG F746_GUI F746_SAI_IO FrequencyResponseDrawer LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed
Fork of F746_SD_GraphicEqualizer by
FileSelectorWav.hpp
00001 //-------------------------------------------------------------- 00002 // FileSelector class 00003 // SD カード内のファイル名の一覧を表示し,ファイルを選択する 00004 // 00005 // 2016/04/18, Copyright (c) 2016 MIKAMI, Naoki 00006 //-------------------------------------------------------------- 00007 00008 #ifndef FILE_SELECTOR_HPP 00009 #define FILE_SELECTOR_HPP 00010 00011 #include "mbed.h" 00012 #include "Label.hpp" 00013 #include "ButtonGroup.hpp" 00014 #include "SD_WavReader.hpp" 00015 #include "SDFileSystem.h" 00016 #include <algorithm> // sort() で使用 00017 #include <string> 00018 00019 00020 namespace Mikami 00021 { 00022 class FileSelector 00023 { 00024 public: 00025 FileSelector(uint8_t x0, uint8_t y0, int maxFiles, 00026 int maxNameLength, SD_WavReader &reader) 00027 : X_(x0), Y_(y0), W_H_(24), V_L_(36), 00028 MAX_FILES_(maxFiles), MAX_NAME_LENGTH_(maxNameLength), 00029 BASE_COLOR_(0xFFDBAD17), TOUCHED_COLOR_(0xFFF8F8FB), 00030 fileNames_(new string[maxFiles]), 00031 sortedFileNames_(new string[maxFiles]), 00032 nonString_(NULL), rect_(NULL), fileNameLabels_(NULL), 00033 lcd_(GuiBase::GetLcdPtr()), 00034 sdReader_(reader), prevFileCount_(0), prev_(-1) {} 00035 00036 ~FileSelector() 00037 { 00038 for (int n=0; n<fileCount_; n++) 00039 delete fileNameLabels_[n]; 00040 delete[] fileNameLabels_; 00041 delete rect_; 00042 delete[] nonString_; 00043 delete[] sortedFileNames_; 00044 delete[] fileNames_; 00045 } 00046 // ren: show a string 00047 void ren_Msg(int pos, char msg[]) 00048 { int lin, y_pos[7]= {0,70,130,160,190,220,250}; 00049 if(pos<0) lin=0; 00050 else if (pos>6) lin=6; 00051 else lin=pos; 00052 Label renLabel(240, y_pos[lin], msg, Label::CENTER,Font20); 00053 } 00054 00055 bool CreateTable() 00056 { 00057 DIR* dp = opendir("/sd"); 00058 fileCount_ = 0; 00059 if (dp != NULL) 00060 { 00061 dirent* entry; 00062 // ren: change n<256 to n<2560 00063 for (int n=0; n<2560; n++) 00064 { 00065 entry = readdir(dp); 00066 if (entry == NULL) break; 00067 00068 string strName = entry->d_name; 00069 if ( (strName.find(".wav") != string::npos) || 00070 (strName.find(".WAV") != string::npos) ) 00071 { 00072 sdReader_.Open(strName); // ファイルオープン 00073 /**{ 00074 char tmpmsg[500]; 00075 sprintf(tmpmsg, "1..found a file: %s", strName.c_str()); 00076 ren_Msg(1,tmpmsg); 00077 sprintf(tmpmsg, "2..fileCount_: %d", fileCount_); 00078 ren_Msg(2,tmpmsg); 00079 }**/ 00080 // PCM,16 ビットステレオ,標本化周波数 44.1 kHz 以外のファイルは除外 00081 if (sdReader_.IsWavFile()) 00082 { 00083 fileNames_[fileCount_] = strName; 00084 fileCount_++; 00085 } 00086 /**{ 00087 char tmpmsg[500]; 00088 sprintf(tmpmsg, "fileCount_: %d", fileCount_); 00089 ren_Msg(3, tmpmsg); 00090 }**/ 00091 sdReader_.Close(); 00092 } 00093 00094 00095 if (fileCount_ >= MAX_FILES_) break; 00096 } 00097 closedir(dp); 00098 } 00099 else 00100 return false; 00101 00102 if (fileCount_ == 0) return false; 00103 00104 if (nonString_ == NULL) delete[] nonString_; 00105 nonString_ = new string[fileCount_]; 00106 for (int n=0; n<fileCount_; n++) nonString_[n] = ""; 00107 00108 if (rect_ != NULL) delete rect_; 00109 rect_ = new ButtonGroup(X_, Y_, W_H_, W_H_, fileCount_, 00110 nonString_, 0, V_L_-W_H_, 1, 00111 -1, true,Font12, 0, GuiBase::ENUM_BACK, 00112 BASE_COLOR_, TOUCHED_COLOR_); 00113 for (int n=0; n<fileCount_; n++) rect_->Erase(n); 00114 CreateLabels(); 00115 prevFileCount_ = fileCount_; 00116 00117 return true; 00118 } 00119 00120 // ファイルを選択する 00121 bool Select(string fileList[],int *idx) 00122 { 00123 int n; 00124 if (rect_->GetTouchedNumber(n)) 00125 { 00126 fileNameLabels_[n]->Draw(GetFileNameNoExt(n), TOUCHED_COLOR_); 00127 if ((prev_ >= 0) && (prev_ != n)) 00128 fileNameLabels_[prev_]->Draw(GetFileNameNoExt(prev_)); 00129 prev_ = n; 00130 *idx = n; 00131 for (int n=0; n<fileCount_; n++) fileList[n] = sortedFileNames_[n]; 00132 // fileName = sortedFileNames_[n]; 00133 //printf("\nSelected file : %s", fileName); 00134 return true; 00135 } 00136 else 00137 return false; 00138 } 00139 00140 // ファイルの一覧の表示 00141 void DisplayFileList(int highlighted, bool sort = true) 00142 { 00143 for (int n=0; n<fileCount_; n++) 00144 sortedFileNames_[n] = fileNames_[n]; 00145 if (sort) 00146 std::sort(sortedFileNames_, sortedFileNames_+fileCount_); 00147 00148 Erase(X_, 0, MAX_NAME_LENGTH_*((sFONT *)(&Font16))->Width, 288); 00149 rect_->DrawAll(); 00150 for (int n=0; n<fileCount_; n++) 00151 fileNameLabels_[n]->Draw(GetFileNameNoExt(n)); 00152 if((highlighted>-1) && (highlighted < fileCount_)) 00153 { 00154 fileNameLabels_[highlighted]->Draw(GetFileNameNoExt(highlighted), TOUCHED_COLOR_); 00155 prev_ = highlighted; 00156 } 00157 } 00158 00159 void Erase(uint16_t x, uint16_t y, uint16_t width, uint16_t height, 00160 uint32_t color = GuiBase::ENUM_BACK) 00161 { 00162 lcd_->SetTextColor(color); 00163 lcd_->FillRect(x, y, width, height); 00164 } 00165 00166 private: 00167 const uint8_t X_, Y_, W_H_, V_L_; 00168 const int MAX_FILES_; 00169 const int MAX_NAME_LENGTH_; 00170 const uint32_t BASE_COLOR_; 00171 const uint32_t TOUCHED_COLOR_; 00172 00173 string *fileNames_; 00174 string *sortedFileNames_; 00175 string *nonString_; 00176 ButtonGroup *rect_; 00177 Label **fileNameLabels_; 00178 LCD_DISCO_F746NG *lcd_; 00179 SD_WavReader &sdReader_; 00180 int fileCount_, prevFileCount_; 00181 int prev_; 00182 00183 // Label を生成 00184 void CreateLabels() 00185 { 00186 if (fileNameLabels_ != NULL) 00187 { 00188 for (int n=0; n<prevFileCount_; n++) 00189 delete fileNameLabels_[n]; 00190 delete[] fileNameLabels_; 00191 } 00192 fileNameLabels_ = new Label *[fileCount_+1]; 00193 for (int n=0; n<fileCount_; n++) 00194 fileNameLabels_[n] = new Label(X_+30, Y_+5+V_L_*n, "", 00195 Label::LEFT, Font16, 00196 BASE_COLOR_); 00197 } 00198 00199 // 拡張子を削除した文字列を取得 00200 string GetFileNameNoExt(int n) 00201 { 00202 string name = sortedFileNames_[n]; 00203 name.erase(name.find(".")); 00204 return name.substr(0, MAX_NAME_LENGTH_); 00205 } 00206 00207 // disallow copy constructor and assignment operator 00208 FileSelector(const FileSelector&); 00209 FileSelector& operator=(const FileSelector&); 00210 }; 00211 } 00212 #endif // FILE_SELECTOR_HPP
Generated on Wed Jul 13 2022 09:35:21 by
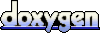