GUI parts for DISCO-F746NG. GuiBase, Button, ButtonGroup, Label, BlinkLabel, NumericLabel, SeekBar, SeekbarGroup
Dependents: F746_SD_GraphicEqualizer_ren0620
Fork of F746_GUI by
SeekBar.hpp
00001 //----------------------------------------------------------- 00002 // SeekBar class -- Header 00003 // 00004 // 2016/04/30, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 00007 #ifndef F746_SEEKBAR_HPP 00008 #define F746_SEEKBAR_HPP 00009 00010 #include "GuiBase.hpp" 00011 #include "Label.hpp" 00012 00013 namespace Mikami 00014 { 00015 class SeekBar : public GuiBase 00016 { 00017 public: 00018 enum Orientation { Holizontal, Vertical }; 00019 00020 struct Point 00021 { 00022 uint16_t x, y; 00023 Point(uint16_t x0 = 0, uint16_t y0 = 0) : x(x0), y(y0) {} 00024 }; 00025 00026 // Constructor 00027 SeekBar(uint16_t x, uint16_t y, uint16_t length, 00028 float min, float max, float initialValue, 00029 Orientation hv = Holizontal, 00030 uint32_t thumbColor = 0xFFB0B0FF, 00031 uint16_t thumbSize = 30, uint16_t width = 4, 00032 uint32_t colorL = LCD_COLOR_LIGHTGRAY, 00033 uint32_t colorH = 0xFFB0B0B0, 00034 uint32_t backColor = GuiBase::ENUM_BACK) 00035 : GuiBase(x, y, Font12, 0, backColor, thumbColor), 00036 L_(length), W_(width), 00037 SIZE_(thumbSize), COLOR_L_(colorL), COLOR_H_(colorH), 00038 MIN_(min), MAX_(max), ORIENT_(hv), v_(initialValue), 00039 labelOn_(false), slided_(false), active_(true) 00040 { Draw(initialValue); } 00041 00042 // Constructor with scale value (only horizontal) 00043 SeekBar(uint16_t x, uint16_t y, uint16_t length, 00044 float min, float max, float initialValue, 00045 string left, string center, string right, 00046 uint32_t thumbColor = 0xFFB0B0FF, 00047 uint16_t thumbSize = 30, uint16_t width = 4, 00048 uint32_t colorL = LCD_COLOR_LIGHTGRAY, 00049 uint32_t colorH = 0xFFB0B0B0, 00050 uint32_t backColor = GuiBase::ENUM_BACK); 00051 00052 ~SeekBar(); 00053 00054 bool Slide(); 00055 float GetValue() { return v_; } 00056 int GetIntValue() { return Round(v_); } 00057 00058 void Activate(); 00059 void Inactivate(); 00060 bool IsActive() { return active_; } 00061 00062 bool IsOnThumb(uint16_t &x, uint16_t &y); 00063 void Draw(float value, bool fill = false); 00064 float ToValue(Point pt); 00065 00066 void SetValue(float v) { v_ = v; } 00067 void SetSlided(bool tf) { slided_ = tf; } 00068 bool GetSlided() { return slided_; } 00069 00070 int Round(float x) { return x + 0.5f - (x < 0); } // Round up on 5 00071 00072 private: 00073 const uint16_t L_, W_; 00074 const uint16_t SIZE_; // Size of thumb 00075 const uint32_t COLOR_L_, COLOR_H_; 00076 const float MIN_, MAX_; 00077 const Orientation ORIENT_; 00078 00079 Label **labelLCR; 00080 float v_; // value of seekbar 00081 bool labelOn_; 00082 bool slided_; 00083 bool active_; 00084 00085 Point ToPoint(float value); 00086 float Saturate(float value); 00087 00088 // disallow copy constructor and assignment operator 00089 SeekBar(const SeekBar&); 00090 SeekBar& operator=(const SeekBar&); 00091 }; 00092 } 00093 #endif // F746_SEEKBAR_HPP
Generated on Fri Jul 22 2022 12:03:18 by
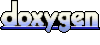